Microservices Scalability: Overcoming Dependencies
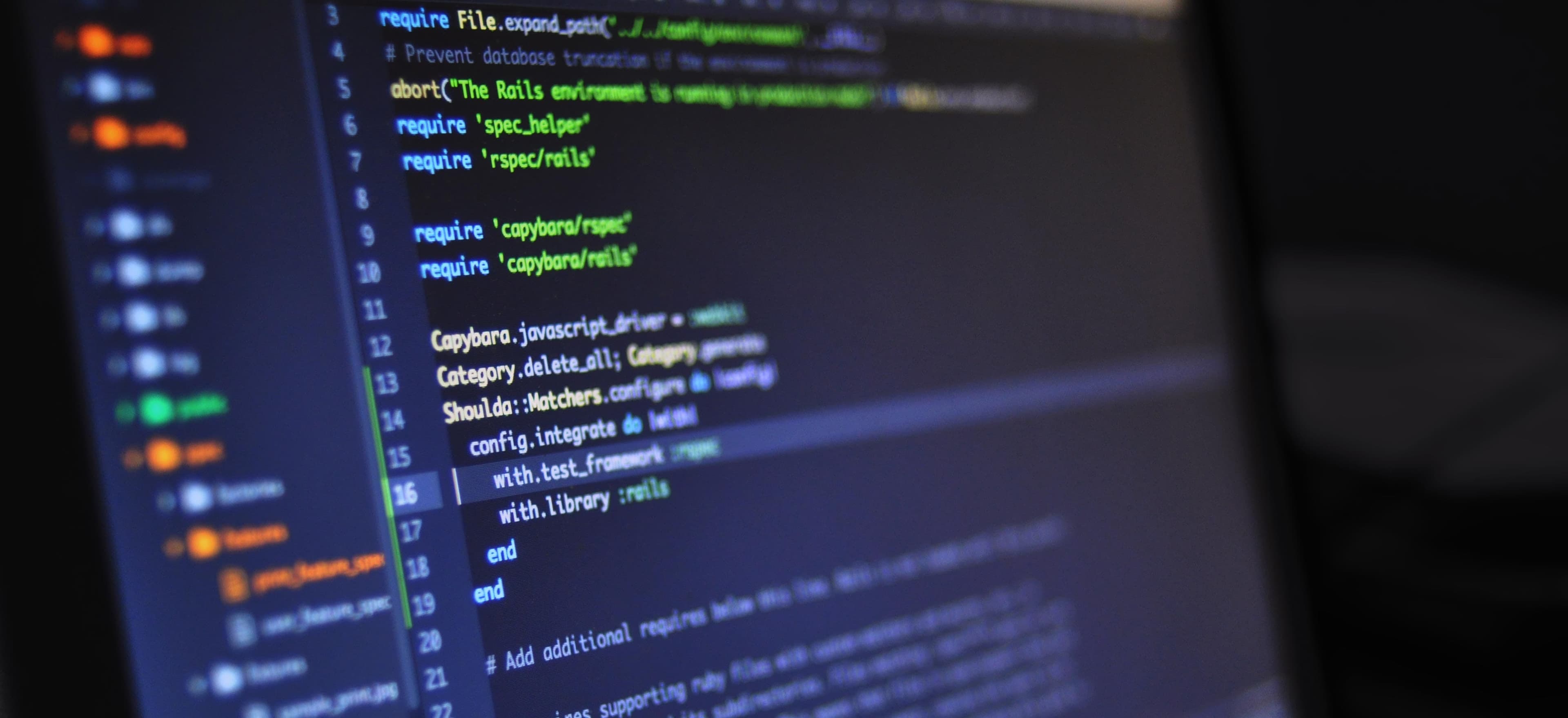
- Published on
Understanding Microservices Scalability and Overcoming Dependencies
Microservices architecture has gained popularity in recent years due to its ability to improve modularity, flexibility, and scalability in software development. However, as your microservices ecosystem grows, you may encounter challenges related to dependencies, which can hinder the scalability of your system. In this article, we will discuss strategies for overcoming dependencies and achieving scalability in a microservices environment using Java.
The Challenge of Dependencies in Microservices
In a microservices architecture, each service is designed to be independently deployable and scalable. However, services often rely on each other to fulfill complex business requirements, leading to dependencies. As the number of interacting services increases, managing these dependencies becomes crucial for achieving scalability. Here are some common challenges associated with dependencies in a microservices environment:
- Cascading Failures: A failure in one service can propagate to dependent services, causing cascading failures and impacting the overall system's scalability and reliability.
- Performance Bottlenecks: Tight dependencies between services can lead to performance bottlenecks, as a slowdown in one service can affect the response times of dependent services.
- Resource Management: Coordinating resources across dependent services, such as database connections and external service calls, can become complex and impact scalability.
- Scaling Constraints: Scaling dependent services independently becomes challenging when tight coupling exists, limiting the ability to efficiently allocate resources based on demand.
Strategies to Overcome Dependencies and Improve Scalability
1. Asynchronous Communication
One way to address dependencies between services is to implement asynchronous communication using message brokers such as Apache Kafka or RabbitMQ. By decoupling interactions between services, asynchronous communication reduces the impact of failures, isolates performance bottlenecks, and provides better resource management.
2. Circuit Breaker Pattern
Applying the circuit breaker pattern, popularized by libraries like Netflix Hystrix, can help prevent cascading failures by providing fallback mechanisms when dependent services are unavailable. In Java, frameworks like Resilience4j offer comprehensive support for implementing the circuit breaker pattern and can be seamlessly integrated into microservices.
3. Event-Driven Architecture
Embracing an event-driven architecture enables services to communicate through events, promoting loose coupling and improving scalability. Java frameworks like Spring Cloud Stream provide abstractions for building event-driven microservices and integrating with message brokers, facilitating the adoption of event-driven patterns.
4. Service Mesh
Introducing a service mesh, such as Istio or Linkerd, can centralize the management of service-to-service communication, resilience, and observability. Service meshes provide features like load balancing, circuit breaking, and distributed tracing, reducing the impact of dependencies on scalability and overall system resilience.
5. Domain-Driven Design (DDD)
Adopting domain-driven design principles can help in identifying bounded contexts and reducing dependencies between microservices. By aligning service boundaries with the domain, DDD promotes autonomous and scalable microservices. The use of DDD concepts, such as aggregates and domain events, can further decouple services and improve scalability.
Implementing Scalability Strategies in Java Microservices
Now, let's explore how to implement these scalability strategies in a Java microservices ecosystem.
Asynchronous Communication with Apache Kafka
// Example of producing a message to Apache Kafka topic
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
public class KafkaProducerExample {
public static void main(String[] args) {
Properties props = new Properties();
props.put("bootstrap.servers", "kafka-broker1:9092,kafka-broker2:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
ProducerRecord<String, String> record = new ProducerRecord<>("my-topic", "key", "value");
producer.send(record);
producer.close();
}
}
In this example, we use the Apache Kafka Java client to produce a message to a Kafka topic asynchronously, demonstrating how to decouple service interactions using a message broker.
Integrating Resilience4j for Circuit Breaking
// Example of using Resilience4j for circuit breaking
import io.github.resilience4j.circuitbreaker.CircuitBreaker;
import io.github.resilience4j.circuitbreaker.CircuitBreakerConfig;
public class Resilience4jExample {
public static void main(String[] args) {
CircuitBreakerConfig config = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.ringBufferSizeInClosedState(5)
.build();
CircuitBreaker circuitBreaker = CircuitBreaker.of("myCircuitBreaker", config);
circuitBreaker.getEventPublisher()
.onSuccess(event -> System.out.println("Success"))
.onError(event -> System.out.println("Error"));
circuitBreaker.executeRunnable(() -> {
// Call to dependent service
});
}
}
In this snippet, we configure and use Resilience4j's circuit breaker to prevent cascading failures when calling dependent services, enhancing the resilience of our microservices.
Building Event-Driven Microservices with Spring Cloud Stream
// Example of event-driven microservice using Spring Cloud Stream
import org.springframework.cloud.stream.annotation.EnableBinding;
import org.springframework.cloud.stream.annotation.StreamListener;
import org.springframework.cloud.stream.messaging.Sink;
@EnableBinding(Sink.class)
public class EventListener {
@StreamListener(Sink.INPUT)
public void handleEvent(String event) {
// Process the incoming event
}
}
Here, we leverage Spring Cloud Stream to create an event listener that handles incoming events asynchronously, promoting loose coupling and scalability in our microservices ecosystem.
Utilizing Istio for Service Mesh Capabilities
Integrating Istio into your Java microservices environment allows you to leverage powerful service mesh capabilities, such as traffic management, load balancing, and fault injection, thereby reducing the impact of dependencies on scalability and resilience.
A Final Look
In conclusion, overcoming dependencies is essential for achieving scalability in a microservices architecture. By embracing asynchronous communication, resilience patterns, and event-driven design, coupled with the adoption of Java frameworks and tools, you can effectively mitigate the challenges posed by dependencies and create a scalable microservices ecosystem. As your microservices ecosystem continues to evolve, addressing dependencies and promoting scalability will remain fundamental to the success of your architecture.
To further explore these concepts and enhance your understanding of microservices scalability, consider diving deeper into the official documentation of Apache Kafka and Resilience4j's user guide. These resources will provide valuable insights into implementing scalable and resilient microservices with Java.
Now armed with these strategies and practical examples, you are well-equipped to navigate the complexities of dependencies and scalability in your Java microservices architecture.
Remember, overcoming dependencies and achieving scalability is an ongoing journey, and continuous refinement of your architecture will be key to sustaining a robust and resilient microservices ecosystem.