Handling JSON Serialization with MOXy in JAX-RS
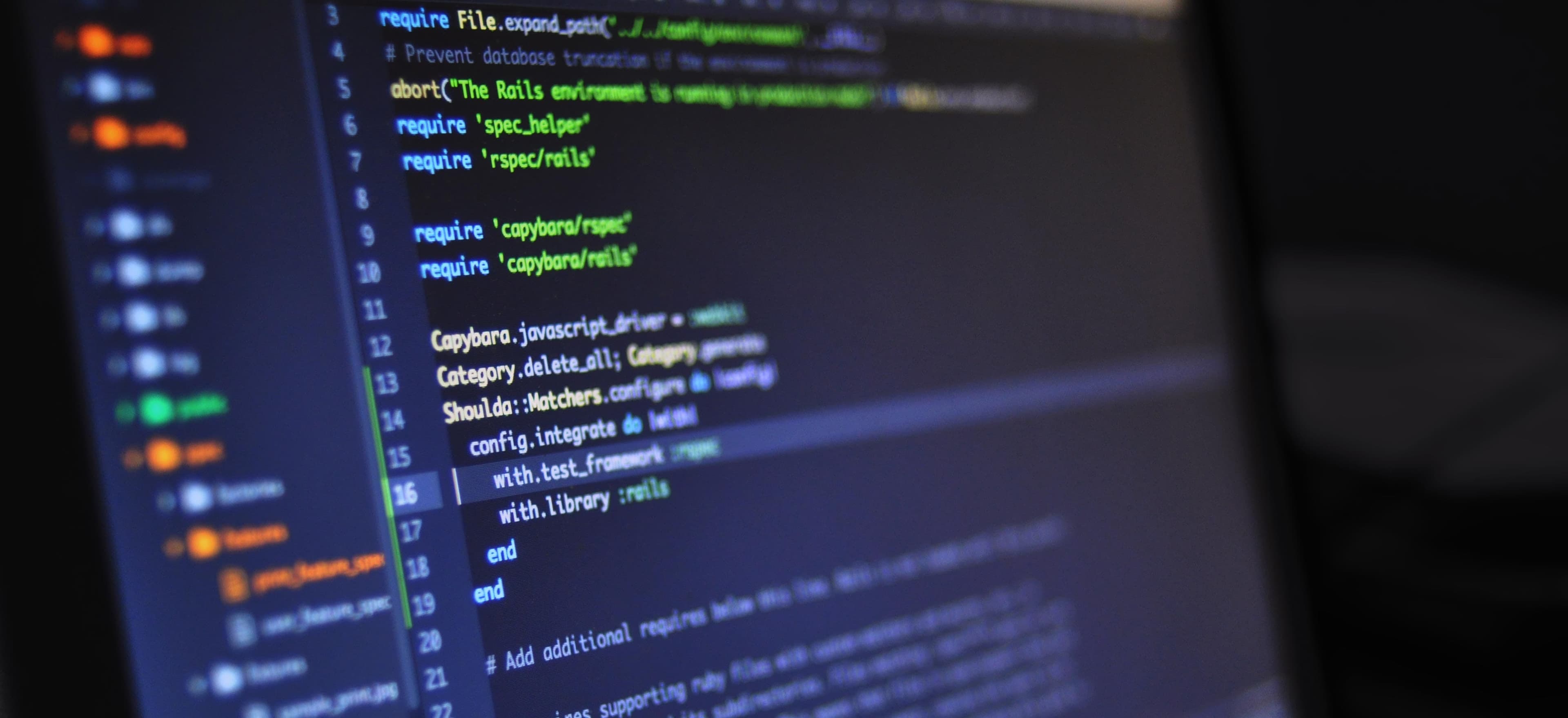
- Published on
Unleashing the Power of MOXy for JSON Serialization in JAX-RS
In the world of Java web development, Java API for RESTful Web Services (JAX-RS) is a powerful tool for building web services. When it comes to handling JSON serialization, MOXy (EclipseLink MOXy) provides a convenient and efficient solution. In this article, we will explore how to leverage MOXy for JSON serialization in JAX-RS, understanding its benefits and how to implement it effectively.
What is MOXy?
MOXy is a component of EclipseLink, which is an open-source persistence framework that is being used for JPA (Java Persistence API) implementations. EclipseLink MOXy, however, provides support for JSON binding alongside its existing XML and JSON bindings. This allows developers to easily serialize and deserialize Java objects to and from JSON.
Setting up MOXy in JAX-RS
To start using MOXy for JSON serialization in a JAX-RS application, you will first need to add the required dependencies to your project. Gradle or Maven can be used to include the necessary dependencies, such as eclipselink
and jersey-media-moxy
for the MOXy integration with JAX-RS.
<dependency>
<groupId>org.eclipse.persistence</groupId>
<artifactId>eclipselink</artifactId>
<version>{version}</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-moxy</artifactId>
<version>{version}</version>
</dependency>
After adding the dependencies to your project, you can configure MOXy as the preferred JSON provider for JAX-RS by registering the MoxyJsonFeature
in your JAX-RS Application
subclass or as a Feature
if you are using a programmatic approach.
import org.glassfish.jersey.moxy.json.MoxyJsonFeature;
import javax.ws.rs.core.Application;
import java.util.HashSet;
import java.util.Set;
public class MyApplication extends Application {
@Override
public Set<Class<?>> getClasses() {
Set<Class<?>> classes = new HashSet<>();
classes.add(MoxyJsonFeature.class);
return classes;
}
}
Benefits of Using MOXy for JSON Serialization
Customization of JSON Output
MOXy provides a flexible and powerful way to customize the JSON output of Java objects. By utilizing annotations such as @XmlRootElement
, @XmlElement
, and @XmlTransient
, developers can control how the JSON representation of their Java objects should look like. This level of customization is invaluable when dealing with complex object structures and when specific JSON output formatting is required.
Support for Inheritance and Polymorphism
When working with inheritance and polymorphic relationships in Java, MOXy simplifies the process of serializing and deserializing objects with different types. By using annotations such as @XmlDiscriminatorNode
and @XmlDiscriminatorValue
, developers can ensure that the JSON output contains the necessary information to accurately represent the hierarchy of object types.
Integration with JPA Entities
For developers using JPA entities in their JAX-RS applications, MOXy seamlessly integrates with JPA annotations to provide JSON serialization and deserialization for entity objects. This allows for consistent handling of data objects across the application, whether they are being persisted in a database or serialized as JSON for web service responses.
Best Practices for Using MOXy in JAX-RS
Utilize @XmlTransient for Excluding Fields
In scenarios where certain fields of a Java object should not be included in the JSON output, the @XmlTransient
annotation can be used to exclude those fields from serialization. This can be beneficial when there are internal state variables or sensitive information that should not be exposed in the JSON representation.
Leverage @XmlSeeAlso for Polymorphic Types
When dealing with polymorphic relationships in Java, the @XmlSeeAlso
annotation can be used to specify the subtypes that are expected in the JSON representation. This ensures that MOXy understands the inheritance hierarchy and can handle instances of different subtypes accordingly during serialization and deserialization.
Use @XmlAccessorType for Controlling Field and Property Access
The @XmlAccessorType
annotation allows developers to control how fields and properties are accessed during JSON serialization. By specifying FIELD
or PROPERTY
as the access type, developers can fine-tune the behavior of MOXy when working with object properties, providing greater control over the JSON output.
Closing Remarks
Incorporating MOXy for JSON serialization in JAX-RS applications provides developers with a powerful and versatile tool for handling the transformation of Java objects to and from JSON. Its customizable features, seamless integration with JPA entities, and support for inheritance and polymorphism make it a preferred choice for many Java web developers. By following best practices and understanding the benefits of using MOXy, developers can effectively leverage its capabilities for building robust and efficient web services.
With MOXy, the process of handling JSON serialization in JAX-RS becomes a straightforward and seamless aspect of Java web development, empowering developers to focus on building high-quality, feature-rich web services with ease.
For further exploration, refer to the MOXy documentation and the Jersey documentation.
Start harnessing the power of MOXy for JSON serialization in your JAX-RS applications today!