Optimizing Docker Containers for Linux Performance
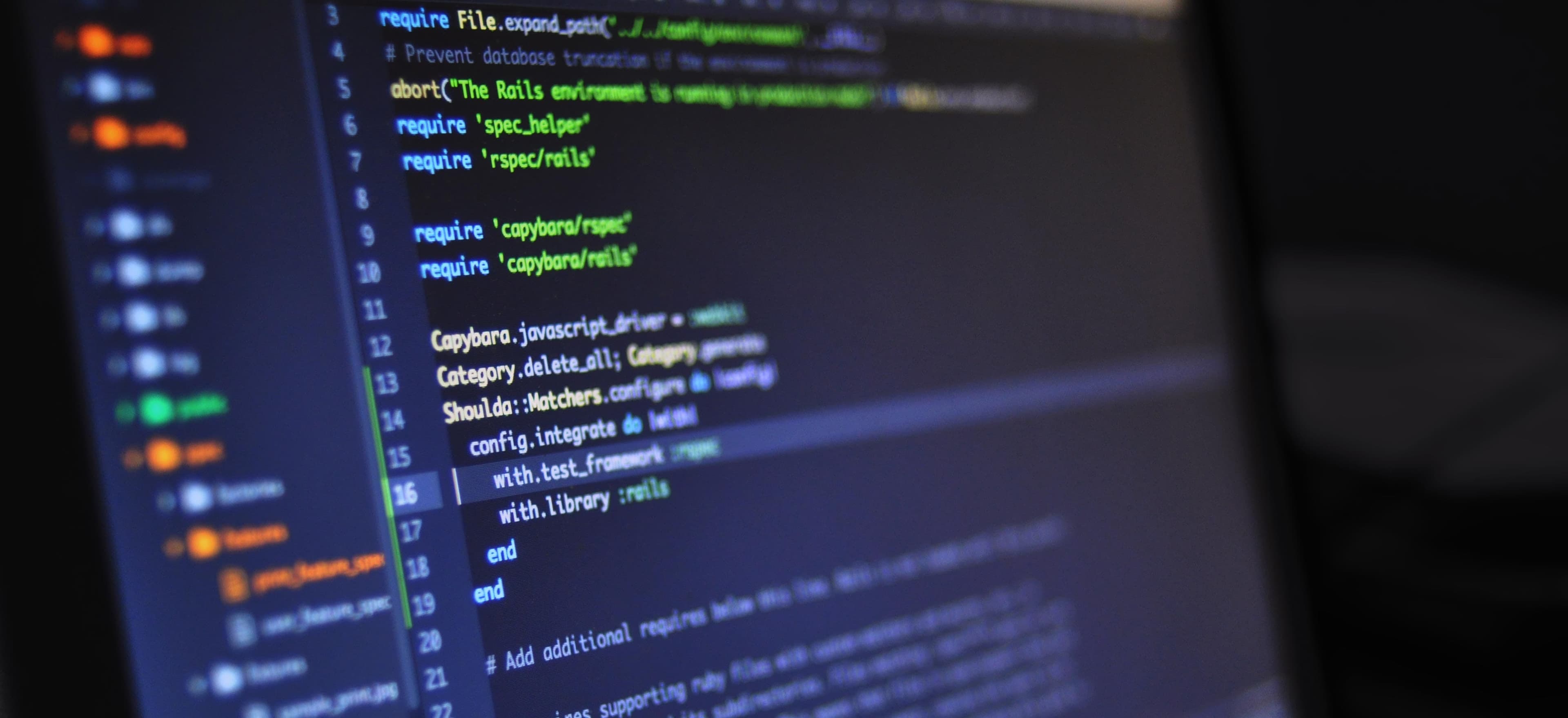
- Published on
Optimizing Docker Containers for Improved Java Application Performance on Linux
In today's technology-driven world, the use of containers has become a standard practice for deploying applications. Docker, in particular, has gained widespread popularity for its ability to package applications and their dependencies into containers that can be easily moved between environments. However, while Docker offers many advantages, it also introduces potential performance challenges, especially when running Java applications. In this article, we'll explore strategies for optimizing Docker containers to achieve improved performance for Java applications on the Linux platform.
Understanding the Performance Challenges
When running Java applications within Docker containers on Linux, several performance challenges may arise. These include:
-
Memory Overhead: Docker introduces overhead for running containers, which can impact the amount of memory available for Java applications. This can lead to increased latency and decreased throughput.
-
CPU Utilization: Docker adds a layer of abstraction to the underlying operating system, which can impact CPU utilization and cause performance overhead for Java applications.
-
Networking Overhead: Docker networking introduces additional overhead, which can impact the network performance of Java applications, especially in microservices-based architectures.
Optimizing Docker Containers for Java Applications
To address these challenges and optimize Docker containers for running Java applications on Linux, consider the following best practices:
1. Use a Base Image Optimized for Java
When building Docker images for Java applications, start with a base image that is optimized for running Java. For example, you can use the official OpenJDK or Oracle JDK images as your base image. These images are specifically designed for running Java applications and provide a good starting point for optimizing Java performance within Docker containers.
FROM openjdk:11-jre-slim
COPY your-app.jar /app.jar
CMD ["java", "-jar", "/app.jar"]
2. Configure JVM Options
Optimizing the Java Virtual Machine (JVM) options can significantly impact the performance of Java applications running in Docker containers. Consider setting appropriate JVM options such as heap size, garbage collection settings, and thread settings to match the container's resources and characteristics.
ENV JAVA_OPTS="-Xms512m -Xmx1024m -XX:+UseG1GC"
3. Limit Memory and CPU Resources
Docker provides the ability to limit the memory and CPU resources available to containers using flags such as --memory
and --cpus
. By properly restricting resources, you can prevent containers from consuming excessive memory and CPU, thereby improving overall performance and resource utilization.
docker run --memory=2g --cpus=2 your-java-app
4. Optimize Networking
In a microservices architecture, Docker networking plays a crucial role in the performance of Java applications. Consider using Docker's built-in networking capabilities, such as user-defined bridge networks or overlay networks, to optimize communication between containers and minimize networking overhead.
5. Use Multi-Stage Builds
Utilize multi-stage Docker builds to reduce the size of the final image and exclude unnecessary build dependencies from the runtime image. This can lead to a smaller footprint and faster startup times for Java applications within Docker containers.
# Build Stage
FROM maven:3.6.3-openjdk-11 AS builder
WORKDIR /app
COPY pom.xml .
RUN mvn dependency:go-offline
# Copy source code and build application
COPY src /app/src
RUN mvn package
# Production Stage
FROM openjdk:11-jre-slim
COPY --from=builder /app/target/your-app.jar /app.jar
CMD ["java", "-jar", "/app.jar"]
6. Utilize Volume Mounts for Caches
When dealing with build tools and dependencies, consider using volume mounts to cache dependencies and build artifacts between container runs. This can significantly improve build times and reduce the overhead of downloading dependencies repeatedly.
docker run -v ~/.m2:/root/.m2 your-java-build-container
7. Monitor and Tune Overhead
Utilize monitoring tools such as Prometheus and Grafana to track and analyze the performance of Java applications within Docker containers. Use the insights gathered to fine-tune JVM options, memory allocation, and CPU utilization for optimal performance.
To Wrap Things Up
Optimizing Docker containers for running Java applications on Linux involves considering a range of factors, from base image selection to fine-tuning JVM options and resource allocation. By following the best practices outlined in this article, you can effectively mitigate performance challenges and achieve improved performance for Java applications within Docker containers on the Linux platform.
For further reading on Docker optimization and Java application performance tuning, refer to the following resources:
- Docker Best Practices for Java Applications
- Understanding JVM Memory Management
- Optimizing Java Applications for Docker
Remember, optimizing Docker containers for Java applications is an ongoing process, and it requires continuous monitoring, testing, and refinement to achieve the best performance outcomes.