Managing Asynchronous Operations in Vert.x 2.0 with RxJava
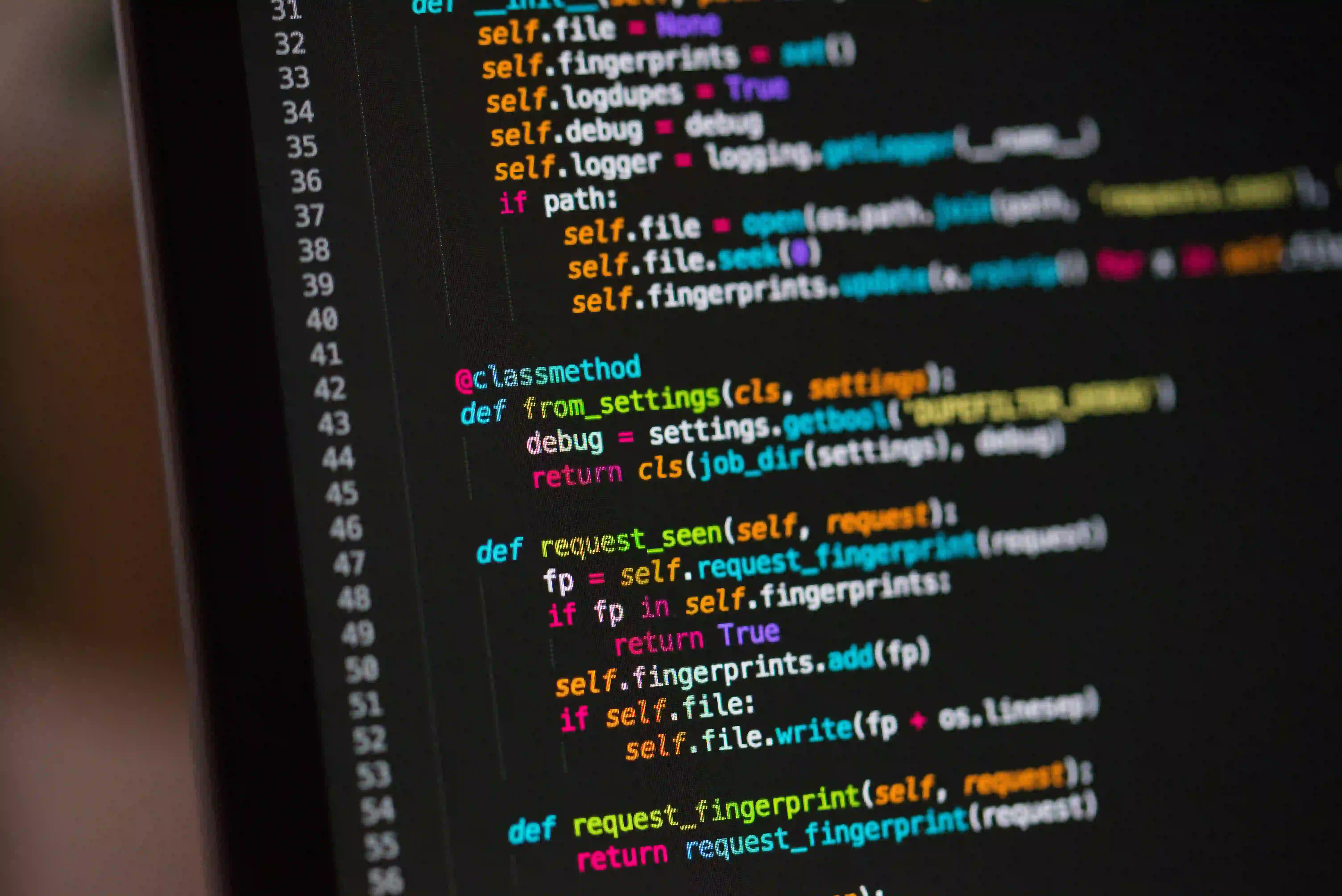
Managing Asynchronous Operations in Vert.x 2.0 with RxJava
Asynchronous programming is an essential part of developing scalable and efficient applications. Vert.x is a powerful and lightweight framework for building reactive applications on the Java Virtual Machine (JVM). With the release of Vert.x 2.0, developers can take advantage of reactive programming with RxJava to manage asynchronous operations in a more concise and efficient manner.
In this article, we will explore how to leverage RxJava to handle asynchronous operations in Vert.x 2.0, covering the basics of reactive programming, integrating RxJava with Vert.x, and demonstrating how to write asynchronous code using its powerful features.
Understanding Reactive Programming and RxJava
Reactive programming is a paradigm that focuses on asynchronous data streams and the propagation of change. It provides a more declarative approach to working with asynchronous data by allowing developers to compose and manipulate streams of events. RxJava is a popular implementation of reactive programming for the JVM, offering a rich set of operators for working with asynchronous data streams.
By using RxJava with Vert.x, developers can take advantage of its powerful features, such as Observable and Single, to handle asynchronous operations in a more concise and readable way. Additionally, RxJava provides operators for handling concurrency, error handling, and more, making it a perfect fit for managing asynchronous tasks in Vert.x 2.0.
Integrating RxJava with Vert.x
To start using RxJava with Vert.x, you will need to include the RxJava dependency in your project. You can add the RxJava dependency to your Maven project by adding the following dependency to your pom.xml
file:
<dependency>
<groupId>io.reactivex.rxjava2</groupId>
<artifactId>rxjava</artifactId>
<version>2.2.19</version>
</dependency>
After adding the dependency, you can use RxJava's powerful features in your Vert.x application.
Writing Asynchronous Code with RxJava in Vert.x
Let's dive into some examples to understand how to write asynchronous code using RxJava in a Vert.x application.
Example 1: Handling Asynchronous Tasks with Observables
import io.vertx.core.Vertx;
import io.reactivex.Observable;
public class RxJavaVertxExample {
public static void main(String[] args) {
Vertx vertx = Vertx.vertx();
Observable<String> observable = Observable.create(emitter -> {
vertx.setTimer(1000, id -> {
emitter.onNext("Hello, RxJava in Vert.x!");
emitter.onComplete();
});
});
observable.subscribe(System.out::println, Throwable::printStackTrace);
}
}
In this example, we create an Observable that emits a "Hello, RxJava in Vert.x!" message after a 1000-millisecond delay using vertx.setTimer()
. We then subscribe to the Observable to receive the emitted value and handle any errors.
Example 2: Managing Asynchronous Operations with Singles
import io.vertx.core.Vertx;
import io.reactivex.Single;
public class RxJavaSingleVertxExample {
public static void main(String[] args) {
Vertx vertx = Vertx.vertx();
Single<String> single = Single.create(emitter -> {
vertx.setTimer(1000, id -> {
emitter.onSuccess("Hello, RxJava Single in Vert.x!");
});
});
single.subscribe(System.out::println, Throwable::printStackTrace);
}
}
In this example, we use a Single to handle a single asynchronous result, emitting a "Hello, RxJava Single in Vert.x!" message after a 1000-millisecond delay. We then subscribe to the Single to handle the emitted value or any errors.
Why Use RxJava for Asynchronous Operations in Vert.x?
-
Declarative and Readable Code: RxJava provides a more declarative and readable way to handle asynchronous operations, making the code easier to understand and maintain.
-
Rich Set of Operators: RxJava offers a rich set of operators for working with asynchronous data streams, including operators for mapping, filtering, transforming, and combining data, as well as handling concurrency and error conditions.
-
Back Pressure Support: RxJava provides built-in support for back pressure, allowing for better control over the flow of data and preventing overflow in scenarios where the producer is faster than the consumer.
-
Interoperability: RxJava seamlessly integrates with Vert.x, allowing developers to leverage its features while building reactive applications with Vert.x 2.0.
Key Takeaways
In this article, we've explored how to manage asynchronous operations in Vert.x 2.0 with RxJava, covering the basics of reactive programming, integrating RxJava with Vert.x, and writing asynchronous code using its powerful features. By leveraging RxJava, developers can build more efficient and scalable applications by handling asynchronous tasks in a more concise and readable manner.
Reactive programming with RxJava in Vert.x opens up new possibilities for building reactive, event-driven applications, offering a paradigm shift in handling asynchronous operations on the JVM. Incorporating RxJava into your Vert.x projects can lead to more maintainable, scalable, and performant applications, providing a competitive edge in the modern software development landscape.
By embracing reactive programming and the power of RxJava in Vert.x, developers can unlock the potential for building high-performance, responsive, and resilient applications that meet the demands of today's distributed and event-driven architectures.