Choosing the Right Compression Method
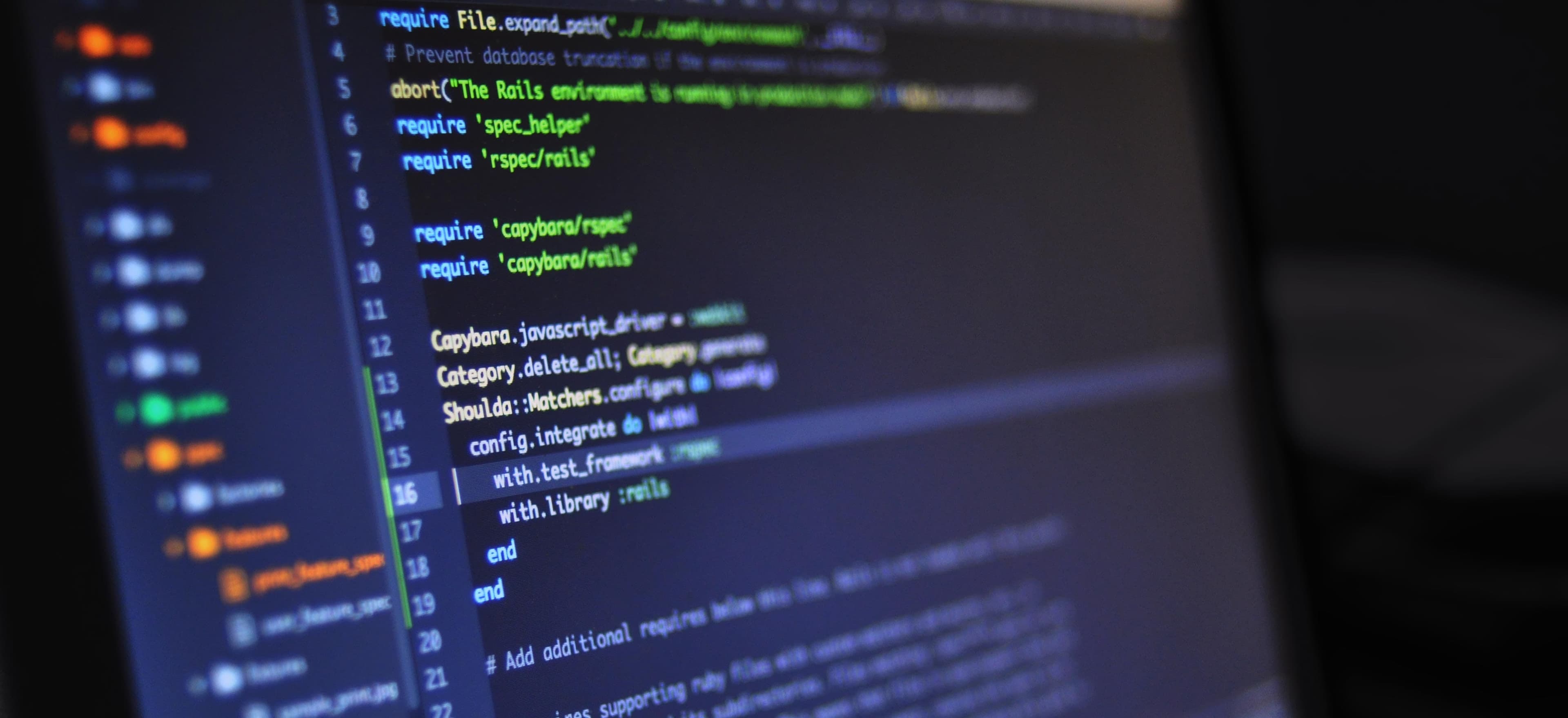
- Published on
When dealing with Java applications, optimizing performance is crucial to ensure smooth user experience and efficient use of resources. One common approach to achieving this is through data compression. By reducing the size of data, we can improve transfer speeds, decrease storage requirements, and overall enhance the application's performance. However, with the multitude of compression methods available, it's important to choose the right one to best suit the specific requirements of your Java application.
Why Data Compression Matters
Data compression plays a significant role in reducing the amount of data that needs to be transmitted over a network or stored in a database. This is particularly important in scenarios where large volumes of data are involved, such as in web applications, file processing, or data streaming.
In Java, the java.util.zip
package provides the functionality to work with standard ZIP files. The java.util.zip
package is suitable for general-purpose compression and decompression. However, there are other third-party libraries and algorithms that can be more efficient for certain types of data.
Understanding Compression Algorithms
Different compression algorithms have varying characteristics in terms of compression ratio, speed, and memory usage. It's essential to understand these factors to choose the most suitable algorithm for a specific use case.
Compression Ratio
The compression ratio refers to the size reduction achieved by a compression algorithm. A higher compression ratio means more significant savings in storage or bandwidth, but often at the cost of increased computational requirements.
Compression Speed
The speed of compression and decompression varies among algorithms. Some algorithms prioritize speed over achieving the highest compression ratios. In situations where data needs to be processed in real-time, a faster compression algorithm might be more suitable.
Memory Usage
Compression algorithms differ in their memory requirements. Some algorithms may consume more memory during the compression process, which can be a crucial factor to consider in memory-constrained environments.
Choosing the Right Compression Method in Java
Let's delve into some of the popular compression methods and how to choose the right one in the context of Java applications.
Deflate Algorithm
The Deflate algorithm, which is widely used in the ZIP and gzip formats, provides a good balance between compression ratio and speed. It's suitable for scenarios where a compromise between compression effectiveness and computational resources is needed. In Java, the Deflate algorithm is implemented in the Deflater
and Inflater
classes in the java.util.zip
package.
Here's an example of using the Deflate algorithm in Java:
import java.util.zip.Deflater;
import java.util.zip.Inflater;
public class DeflateCompressionExample {
public static void main(String[] args) {
byte[] input = "Hello, world!".getBytes();
Deflater deflater = new Deflater();
deflater.setInput(input);
deflater.finish();
byte[] compressedData = new byte[100];
int compressedSize = deflater.deflate(compressedData);
Inflater inflater = new Inflater();
inflater.setInput(compressedData, 0, compressedSize);
byte[] decompressedData = new byte[100];
int decompressedSize = inflater.inflate(decompressedData);
inflater.end();
System.out.println("Original: " + new String(input));
System.out.println("Decompressed: " + new String(decompressedData, 0, decompressedSize));
}
}
In this example, we use the Deflater
and Inflater
classes to compress and decompress a simple string.
LZ4 Algorithm
The LZ4 algorithm is known for its high-speed compression and decompression. It excels in scenarios where fast data processing is a priority. Although it may not achieve the highest compression ratios, its speed makes it suitable for use cases such as real-time data processing, network communication, and in-memory compression.
The LZ4 Java library provides efficient implementations of the LZ4 algorithm for Java applications. Integrating this library into your project can significantly improve the performance of data compression in Java.
Snappy Compression
Snappy is another compression algorithm designed for high-speed compression and decompression. It offers a balance between speed and compression ratio, making it suitable for scenarios where fast data processing is essential without a significant compromise on compression efficiency.
Google's Snappy Java library provides a Java binding for the Snappy compression/decompression library. It offers a simple and efficient way to incorporate Snappy compression into Java applications.
Evaluation of Use Case
When evaluating which compression method to use, consider the specific requirements and constraints of the use case. Some key considerations include:
- Type of Data: Understand the nature of the data being compressed. Is it textual, binary, or a mix of both? Different compression algorithms perform better on specific types of data.
- Resource Constraints: Consider the available computational resources, memory limitations, and the desired trade-off between compression ratio and speed.
- Performance Requirements: Determine whether the priority is on achieving the highest compression ratio, the fastest processing speed, or a balance between the two.
Closing Remarks
Choosing the right compression method is essential for optimizing the performance of Java applications. Understanding the characteristics of different compression algorithms, such as compression ratio, speed, and memory usage, is crucial in making an informed decision. By evaluating the specific requirements of the use case, and considering factors such as the type of data, resource constraints, and performance requirements, you can select the most suitable compression method to enhance the efficiency of your Java application. Whether it's the balance of the Deflate algorithm, the speed of LZ4, or the efficiency of Snappy, the right compression method can significantly impact the overall performance of your Java application.
In the ever-evolving landscape of software development, the choice of a suitable compression method can be a game-changer in enhancing the overall user experience and ensuring the optimal utilization of resources within Java applications.