The Impact of String Interning on Java Performance
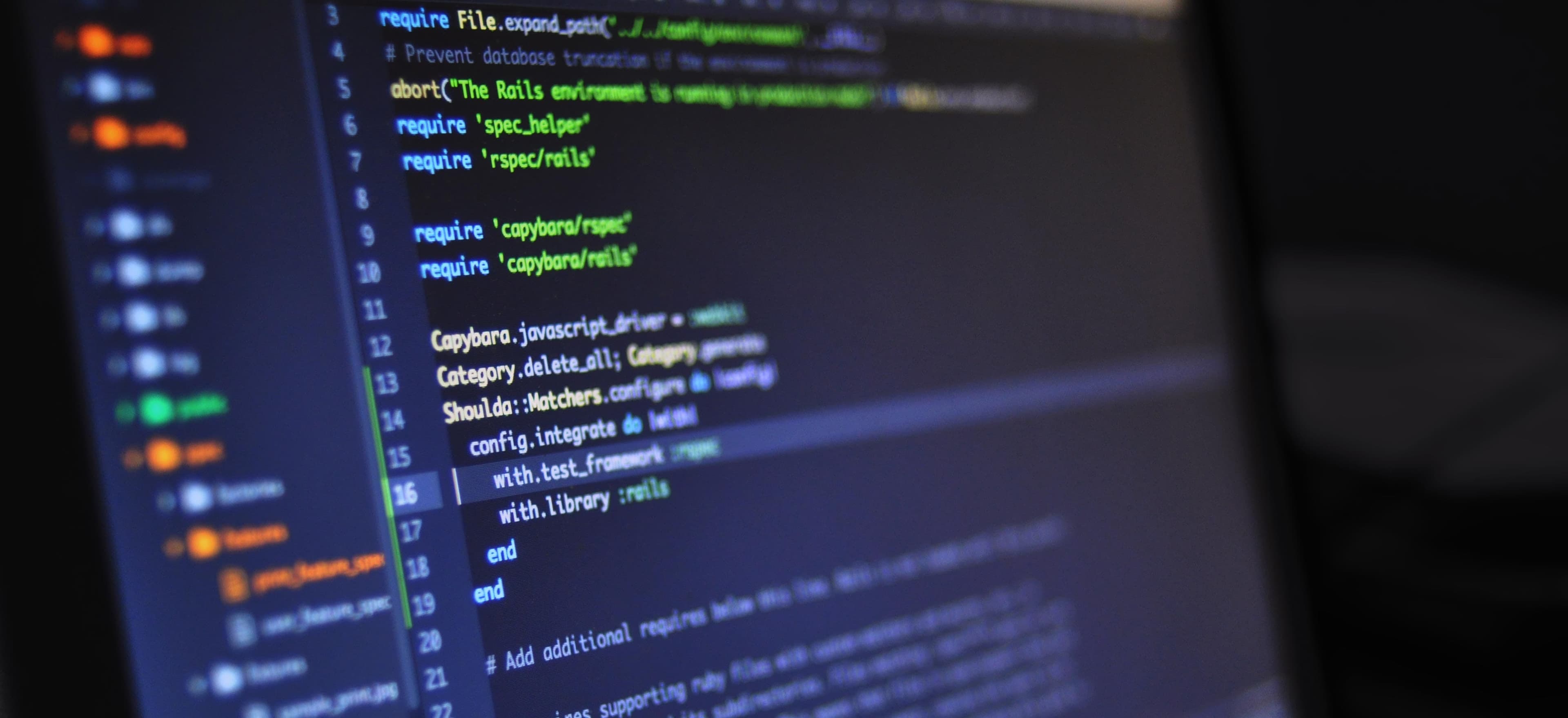
- Published on
The Impact of String Interning on Java Performance
String internment is a concept in Java that can have a significant impact on the performance of your code. When dealing with a lot of string manipulation and comparisons, understanding how string internment works and its influence on memory and performance can be crucial. In this article, we will delve into the concept of string internment, its benefits, potential drawbacks, and how it affects the performance of Java applications.
What is String Interning?
String internment is a process in Java where the runtime keeps only one copy of each distinct string value, which is then shared across the application. This means that if you have multiple strings with the same value, they all point to the same memory location. This can be particularly useful in scenarios where you have a large number of strings with duplicate values, as it can help conserve memory and improve performance by reducing the memory footprint and speeding up string comparisons.
How String Interning Works in Java
In Java, string internment is primarily handled by the String.intern()
method. When this method is called on a string, the JVM checks the string pool to see if an equivalent string is already present. If the string is found, the existing reference is returned. If not, the string is added to the pool and its reference is returned. This ensures that only a single copy of each distinct string value exists in memory.
String s1 = "Hello";
String s2 = new String("Hello").intern();
System.out.println(s1 == s2); // Outputs: true
In this example, s1
and s2
both point to the same memory location, demonstrating how string internment works to conserve memory by reusing existing string instances.
Benefits of String Interning
Memory Efficiency
String internment can lead to significant memory savings, especially in scenarios where there are a large number of duplicate string values. By ensuring that only one copy of each distinct string value is retained in memory, interned strings contribute to reducing the overall memory footprint of the application.
Improved Performance
Interned strings can lead to faster string comparisons due to their unique memory addresses. Since interned strings share the same memory location if they have the same value, comparing interned strings for equality becomes a simple reference comparison (==
) instead of a character-by-character evaluation. This can result in faster performance, particularly in applications that heavily rely on string comparisons.
Drawbacks of String Interning
Memory Overhead
While string internment can be beneficial in terms of memory efficiency, it can also lead to potential memory overhead if not managed carefully. Since interned strings persist in the string pool for the duration of the application, failing to release unnecessary interned strings can lead to increased memory consumption.
Impact on Garbage Collection
The use of string internment can affect garbage collection, as the retained interned strings may prevent the garbage collector from freeing up memory in the string pool. This can potentially lead to longer garbage collection cycles and impact the overall application performance.
Best Practices for Using String Interning
While string internment can offer performance benefits, it's essential to use it judiciously and adhere to best practices to avoid potential drawbacks.
Limit String Internment to Known Cases
It's crucial to identify and intern strings only when there is a clear benefit in terms of memory and performance. Interning all strings indiscriminately can lead to unnecessary memory overhead and potential performance implications.
Consider String Pool Management
Regularly monitor and manage the string pool to ensure that unnecessary interned strings are released when they are no longer needed. This can help mitigate the potential memory overhead associated with string internment.
Be Cautious with External Inputs
When interning strings derived from external inputs, such as user-provided data, it's important to carefully evaluate the potential impact on memory and performance. Interning large volumes of arbitrary strings can lead to unforeseen memory consumption.
Performance Considerations
String Concatenation
String internment can also impact the performance of string concatenation operations. Since the +
operator is frequently used for string concatenation, interned strings can optimize the concatenation process, leading to improved performance in some cases.
String str1 = "Hello";
String str2 = "World";
String result = (str1 + str2).intern();
In this example, the interned result of the concatenation operation can lead to improved performance by reusing existing string instances.
Comparison Operations
String internment is particularly beneficial for comparison operations, as it allows for efficient comparison of string values through reference equality rather than character comparison.
String s1 = "Hello";
String s2 = "Hello";
System.out.println(s1 == s2); // Outputs: true
In this comparison, the interned strings s1
and s2
are efficiently compared using the reference equality (==
) operator, resulting in improved performance.
A Final Look
String internment in Java can have a significant impact on memory efficiency and application performance. By understanding how string internment works and its potential benefits and drawbacks, developers can make informed decisions regarding its usage in their applications. When used judiciously and in accordance with best practices, string internment can contribute to improved memory utilization and performance optimization in Java applications.
In conclusion, while string internment is a valuable tool for optimizing memory usage and improving performance, it should be applied thoughtfully and carefully managed to avoid potential drawbacks. When used effectively, string internment can be a powerful ally in enhancing the performance of Java applications.