Effective Time Management for Early Project Delivery
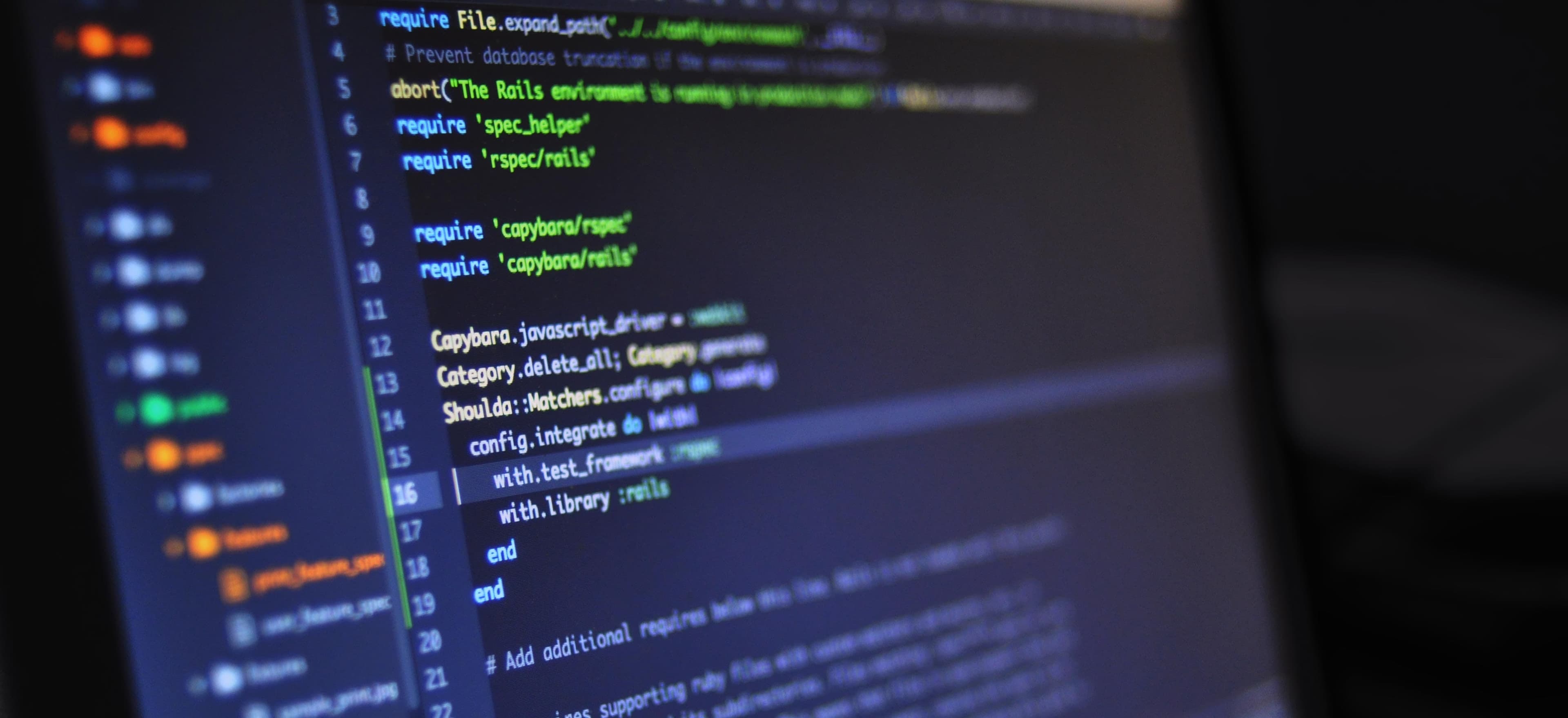
- Published on
Boosting Your Java Project Delivery with Effective Time Management
When it comes to delivering Java projects on time, effective time management is crucial. As a Java developer, it's essential to optimize your workflow to ensure that you meet project deadlines without compromising the quality of your code. In this article, we'll explore some practical tips and techniques to help you manage your time efficiently and deliver Java projects early.
Setting Clear Goals and Priorities
Before diving into writing code, it's essential to have a clear understanding of the project requirements and objectives. Take the time to clarify the goals with your team and stakeholders to ensure alignment. By establishing clear goals and priorities, you can focus your efforts on the most critical aspects of the project, thereby optimizing your time.
Agile Methodologies and Sprint Planning
Embracing Agile methodologies, such as Scrum or Kanban, can significantly improve time management in Java development. By breaking the project into manageable sprints, you can maintain a steady pace of development while regularly reassessing priorities. Sprint planning allows you to allocate specific tasks to each sprint, ensuring that you make steady progress towards your project goals.
Example Sprint Planning in Scrum
public class Sprint {
private String goal;
private List<Task> tasks;
public Sprint(String goal, List<Task> tasks) {
this.goal = goal;
this.tasks = tasks;
}
// Getters and setters
}
public class Task {
private String description;
private int storyPoints;
public Task(String description, int storyPoints) {
this.description = description;
this.storyPoints = storyPoints;
}
// Getters and setters
}
In the above example, a simple representation of a Sprint and Task classes demonstrates how you can encapsulate sprint goals and individual tasks, facilitating organized sprint planning.
Utilizing Integrated Development Environments (IDEs)
Investing time in mastering a powerful Integrated Development Environment (IDE) can significantly boost your productivity as a Java developer. IDEs like IntelliJ IDEA, Eclipse, and NetBeans provide a wide range of features such as code completion, refactoring tools, and debugging capabilities, allowing you to write code more efficiently and with fewer errors.
Test-Driven Development (TDD)
Adopting Test-Driven Development (TDD) practices can help you manage your time effectively by reducing the time spent on debugging and fixing issues later in the development cycle. By writing tests before implementing the actual code, you can ensure that your code meets the specified requirements while catching potential issues early on.
Example of Test-Driven Development in Java
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
The code snippet demonstrates a simple test case for a Calculator
class following the TDD approach, where the test is written before the actual implementation.
Code Reviews and Pair Programming
Engaging in code reviews and pair programming can be a valuable time management practice. By having your code reviewed by peers and collaborating with other developers in pair programming sessions, you can identify potential issues early, learn new techniques, and ultimately write better code in less time.
Automation with Build Tools and Continuous Integration
Integrating build tools like Apache Maven or Gradle into your workflow can automate repetitive tasks such as compilation, testing, and packaging, thereby saving you valuable time. Additionally, setting up a continuous integration pipeline using tools like Jenkins or Travis CI can further streamline the development process by automatically building, testing, and deploying your Java code.
Effective Communication and Collaboration
Clear and efficient communication within your development team is essential for effective time management. By maintaining open channels for communication, leveraging collaboration tools like Slack or Microsoft Teams, and conducting regular stand-up meetings, you can ensure that everyone is aligned, aware of their tasks, and can promptly address any potential roadblocks.
Final Considerations
Time management is a critical skill for Java developers aiming to deliver high-quality projects on time. By setting clear goals, leveraging Agile methodologies, utilizing powerful IDEs, embracing TDD, engaging in code reviews and pair programming, automating repetitive tasks, and fostering effective communication, you can optimize your workflow and ensure early project delivery without compromising code quality. Mastering these time management techniques will not only benefit your current projects but also set the foundation for your future successes as a Java developer.
By implementing these strategies, you can cultivate an efficient and productive development environment, allowing you to consistently deliver high-quality Java projects ahead of schedule.