Handling JSON Parsing Errors in Dropwizard
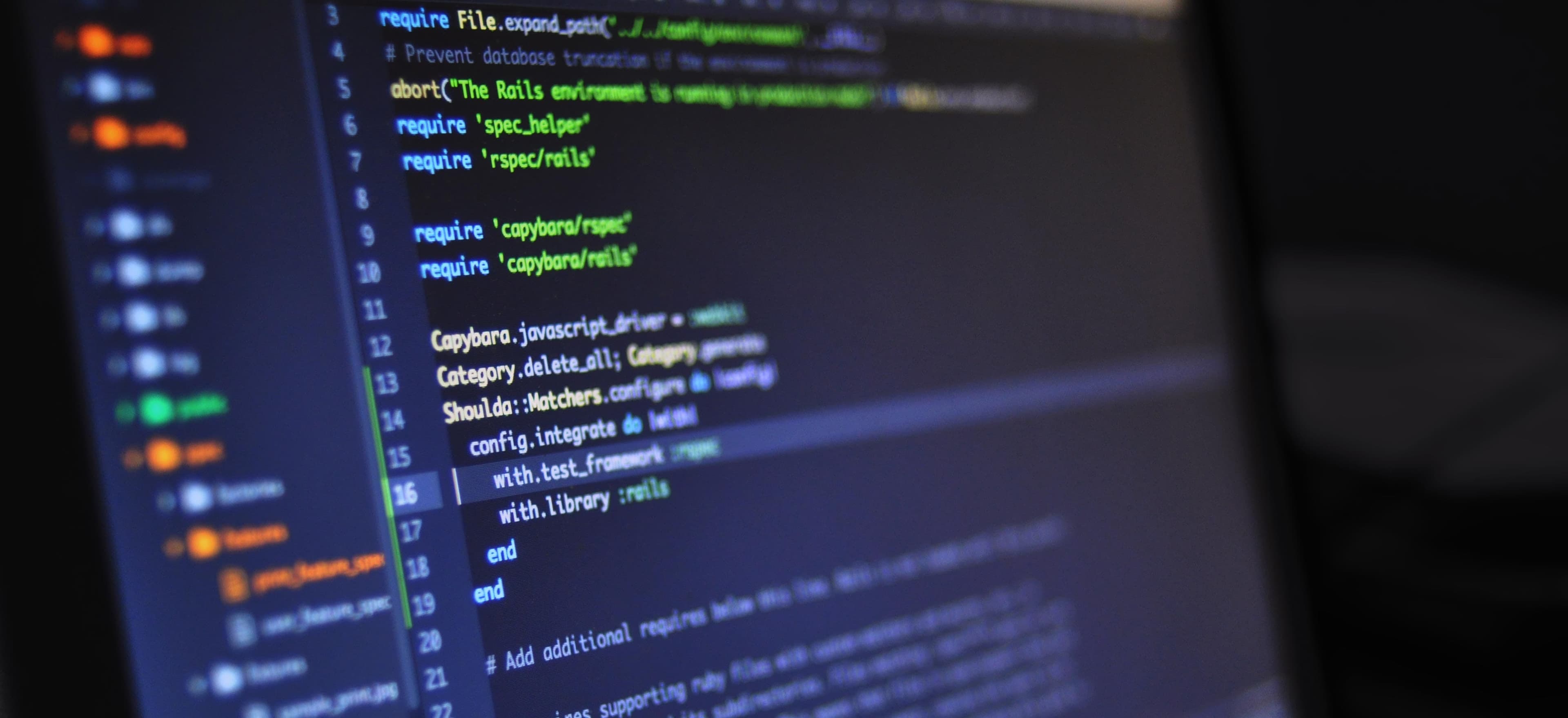
- Published on
Handling JSON Parsing Errors in Dropwizard
When working with APIs in Java, parsing and handling JSON data is a crucial part of the development process. However, dealing with errors during this process can be challenging, especially when using frameworks like Dropwizard. In this article, we'll explore how to handle JSON parsing errors in Dropwizard effectively.
Understanding JSON Parsing Errors
JSON parsing errors occur when there are issues with the structure or formatting of the JSON data being processed. These errors can be caused by malformed JSON syntax, unexpected data types, missing fields, or other similar issues. When building APIs, it's essential to handle these errors gracefully to provide meaningful feedback to clients and maintain the stability of the application.
Dropwizard and JSON Parsing
Dropwizard is a popular Java framework for building RESTful web services. It provides seamless integration with JSON for serializing and deserializing data. Dropwizard uses Jackson, a high-performance JSON processor, for handling JSON data.
Handling JSON Parsing Errors in Dropwizard
Configuring Dropwizard to Customize Error Handling
To customize error handling for JSON parsing in Dropwizard, we can leverage its Jackson
configuration. By customizing the ObjectMapper
used by Dropwizard, we can define how JSON parsing errors are handled.
public class CustomJsonMapper extends ObjectMapper {
public CustomJsonMapper() {
this.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
this.configure(DeserializationFeature.FAIL_ON_IGNORED_PROPERTIES, false);
}
}
In this code snippet, we create a custom ObjectMapper
by extending the ObjectMapper
class provided by Jackson. We then configure the behavior of the ObjectMapper to not fail on unknown or ignored properties during deserialization. This customization allows us to handle JSON parsing errors more gracefully, especially when dealing with evolving APIs and backward compatibility.
Implementing Exception Mappers
In Dropwizard, we can use Exception Mappers to handle specific types of exceptions, including JSON parsing errors. Exception Mappers are used to transform an exception into a relevant response, providing a consistent and standardized way to deal with errors.
@Provider
public class JsonParsingExceptionMapper implements ExceptionMapper<JsonParseException> {
@Override
public Response toResponse(JsonParseException exception) {
ErrorMessage errorMessage = new ErrorMessage("Invalid JSON format", 400);
return Response.status(Response.Status.BAD_REQUEST)
.entity(errorMessage)
.build();
}
}
In the above example, we create a JsonParsingExceptionMapper
that handles JsonParseException
specifically. When a JSON parsing error occurs, this mapper transforms the exception into a 400 Bad Request
response with a custom error message, providing clear feedback to the client about the nature of the error.
Providing Descriptive Error Messages
When handling JSON parsing errors, it's crucial to provide descriptive error messages that convey useful information to the API client. This helps the client understand the nature of the error and take appropriate action.
public class ErrorMessage {
private String message;
private int status;
public ErrorMessage(String message, int status) {
this.message = message;
this.status = status;
}
// Getters and setters
}
In this example, we define a simple ErrorMessage
class to encapsulate the error message and status code. This allows us to consistently return meaningful error messages in the API responses, enhancing the overall developer experience.
Unit Testing for Error Scenarios
As with any critical functionality, it's essential to have robust unit tests for handling JSON parsing errors. By writing tests that simulate various error scenarios, we can ensure that our error handling mechanisms are effective and provide the expected outcomes.
public class JsonParsingErrorTest {
@Test
public void testJsonParsingErrorHandling() {
// Simulate a JSON parsing error and assert the response
// ...
}
@Test
public void testCustomJsonMapperConfiguration() {
// Test the custom ObjectMapper configuration for handling JSON parsing errors
// ...
}
}
Unit testing allows us to verify that the error handling logic behaves as intended and remains resilient to changes in the codebase.
Key Takeaways
In this article, we've delved into the importance of handling JSON parsing errors in Dropwizard, a popular Java framework for building RESTful web services. By customizing the ObjectMapper
, implementing Exception Mappers, providing descriptive error messages, and conducting thorough unit testing, we can ensure that our APIs handle JSON parsing errors gracefully and provide meaningful feedback to clients. Effective error handling not only improves the reliability of our APIs but also enhances the overall developer and end-user experience.
By following the guidelines outlined in this article, developers can build robust, resilient APIs that handle JSON parsing errors with finesse, ensuring smooth interactions with clients and maintaining the integrity of their applications.
For further reading on Dropwizard and JSON error handling, you may find this guide on Dropwizard and this article on Exception Handling in Java useful to expand your knowledge and strengthen your error handling skills.