The Downsides of Monolithic Architecture
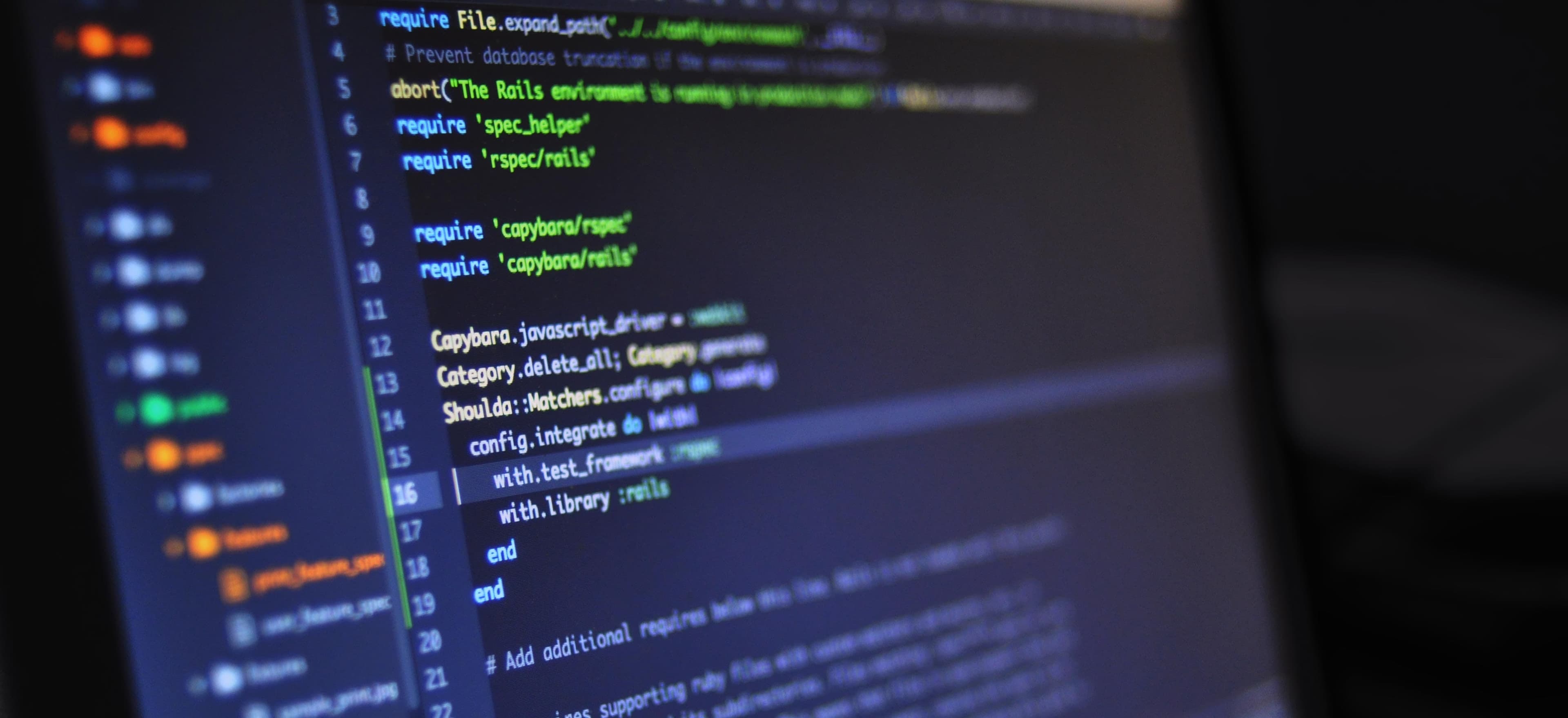
- Published on
In the realm of software development, architecture plays a pivotal role in determining the success and scalability of an application. When it comes to choosing an appropriate architecture for a Java application, developers often find themselves deliberating between monolithic and microservices architectures. While monolithic architecture has its own merits, it also comes with several downsides that need to be carefully considered.
The Drawbacks of Monolithic Architecture
Complexity and Scalability
One of the primary downsides of monolithic architecture lies in its inherent complexity. As an application grows, the codebase becomes larger and more convoluted, making it increasingly challenging to understand, maintain, and scale. In a monolithic architecture, all components are tightly coupled, and any changes made to a specific part of the application can have unforeseen effects on other parts, leading to a higher risk of introducing bugs and making it harder to scale the application independently.
Technology Stack Limitations
In a monolithic architecture, the entire application is built using a single technology stack. This limitation can be restrictive, especially when newer technologies and frameworks emerge that could potentially benefit specific components of the application. With a monolithic architecture, integrating new technologies becomes more cumbersome, as any change to the technology stack affects the entire application.
Continuous Deployment and CI/CD Challenges
In a monolithic architecture, the entire application is deployed as a single unit. This can pose challenges in adopting continuous deployment and continuous integration/continuous delivery (CI/CD) practices. Since any change to the application requires deploying the entire monolith, the deployment process becomes slower and riskier, making it difficult to achieve the agility and efficiency offered by CI/CD pipelines.
Team Collaboration and Autonomy
Monolithic architectures can hinder team collaboration and autonomy. Since the entire codebase is within a single code repository, different teams working on disparate parts of the application may inadvertently impact each other's code, leading to coordination challenges and potential conflicts. Moreover, with a monolithic architecture, it is harder to assign ownership and autonomy to individual teams for specific components, as they are all part of the same monolith.
Mitigating the Downsides with Java and Microservices
Java and Microservices
Java, with its robust ecosystem and extensive support for microservices development, provides a compelling solution to address the downsides of monolithic architecture. By embracing microservices architecture in Java development, developers can effectively mitigate the complexities and limitations associated with monolithic architectures.
Flexibility and Independence
Microservices architecture enables developers to decouple different components of the application, allowing each microservice to be developed, deployed, and scaled independently. This independence grants teams the freedom to choose the most suitable technology stack for each microservice, promoting flexibility and innovation without being constrained by the choices made for other parts of the application.
Scalability and Performance
With microservices, scalability becomes more granular, as individual microservices can be scaled based on their specific demands. This contrasts with monolithic architectures, where the entire application must be scaled as a single unit. The ability to scale only the necessary parts of the system can lead to significant cost savings and improved performance.
CI/CD Readiness
Microservices are well-aligned with CI/CD practices, as each microservice can be developed, tested, and deployed independently. This granular approach to deployment significantly reduces the risk and time associated with releasing new features or updates. Additionally, microservices allow for faster rollbacks in case of issues, as only the affected microservice needs to be addressed, minimizing the impact on the rest of the system.
Team Autonomy and Collaboration
Microservices promote team autonomy by allowing different teams to take ownership of specific microservices. This ownership fosters a sense of responsibility and accountability, empowering teams to make independent decisions regarding technology choices, development practices, and deployment strategies. Furthermore, the decoupled nature of microservices encourages better collaboration, as teams can work on their respective microservices without impacting other parts of the system.
Implementing Microservices in Java
Creating Microservices with Spring Boot
Java developers can leverage Spring Boot, a popular framework for building microservices, to create scalable, resilient, and independently deployable microservices. With its extensive support for building RESTful services and its robust dependency injection capabilities, Spring Boot simplifies the process of developing microservices in Java.
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
// Other CRUD operations for user management
}
In the example above, a UserController is defined to handle requests related to user management. By leveraging Spring Boot's annotations and dependency injection, the UserController can seamlessly interact with the UserService to perform CRUD operations, showcasing the simplicity and elegance of building microservices with Java and Spring Boot.
Communication Between Microservices
Communication between microservices is a critical aspect of microservices architecture. Java developers can utilize frameworks like Spring Cloud to facilitate inter-service communication, service discovery, load balancing, and fault tolerance. By incorporating Spring Cloud's features, such as Feign for declarative REST client and Eureka for service discovery, Java-based microservices can effectively communicate with each other while remaining resilient in the face of network failures.
@FeignClient(name = "user-service")
public interface UserServiceClient {
@GetMapping("/users/{id}")
UserDTO getUserById(@PathVariable Long id);
}
The code snippet demonstrates the usage of Feign to define a declarative REST client for communicating with the user service. By abstracting the communication details, Java developers can focus on building resilient and loosely coupled microservices that seamlessly interact with one another.
Final Considerations
While monolithic architecture has been a dominant force in the realm of software development, its inherent complexities and limitations have led many organizations to embrace microservices architecture as a more viable alternative. By leveraging Java's robust ecosystem and the capabilities of microservices, developers can address the downsides of monolithic architectures, fostering agility, scalability, and autonomy in their applications. With Java's extensive support for building microservices, the transition from monolithic to microservices architecture becomes an attainable and rewarding endeavor for development teams seeking to adapt to the evolving landscape of modern software architecture.
In conclusion, while monolithic architecture has its place, the drawbacks associated with it have paved the way for the ascension of microservices, especially in the context of Java development. As technology continues to evolve, embracing microservices architecture in Java stands as a compelling approach to building resilient, scalable, and maintainable applications, effectively mitigating the downsides that have long plagued monolithic architectures.