Improving Web Application Performance with Asynchronous Servlets
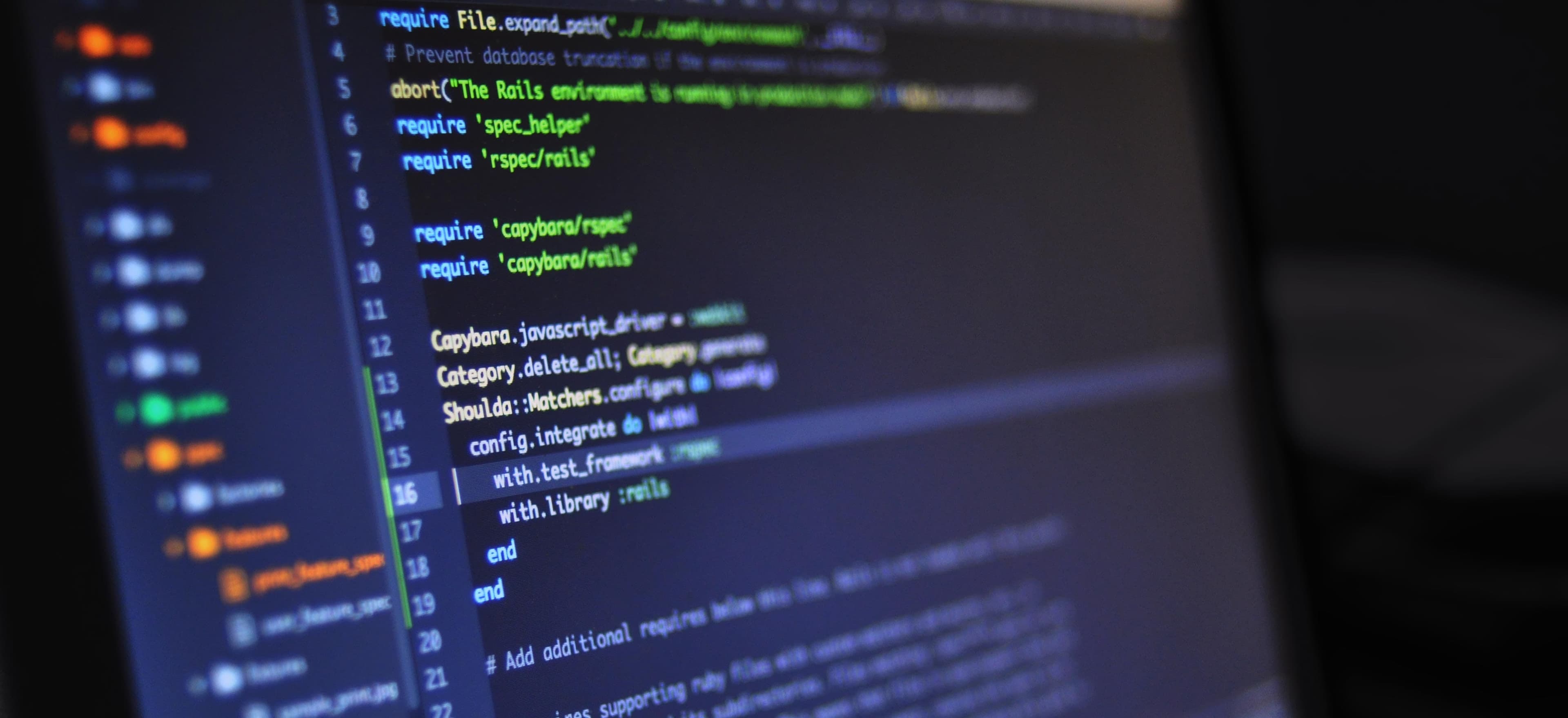
- Published on
Improving Web Application Performance with Asynchronous Servlets
In the world of web development, optimizing performance is critical. Users expect websites and web applications to load quickly, and any delays can result in a poor user experience. Asynchronous Servlets in Java offer a powerful way to improve the performance of web applications by handling requests more efficiently.
Understanding the Problem
When a web server receives a request, it traditionally processes it synchronously. This means that the server dedicates a thread to handle the request from start to finish. If the request involves time-consuming operations such as accessing a database, making external API calls, or performing complex computations, the thread remains blocked until the operation completes. This approach can lead to thread starvation, where all threads are occupied, and new requests have to wait, resulting in poor application performance.
Introducing Asynchronous Servlets
Asynchronous Servlets, introduced in the Servlet 3.0 specification, provide a solution to the problems associated with synchronous request processing. They allow a web application to handle a large number of concurrent connections without consuming a thread per connection. Instead, the server can use a limited number of threads to process a large number of requests concurrently.
Implementing Asynchronous Servlets
Let's take a look at a simple example to illustrate the implementation of an asynchronous servlet.
Setting up the Servlet
@WebServlet(urlPatterns = "/asyncServlet", asyncSupported = true)
public class AsyncServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
AsyncContext asyncContext = request.startAsync(request, response);
asyncContext.start(() -> {
// Asynchronous task implementation
asyncContext.complete();
});
}
}
In this example, we define a servlet AsyncServlet
and annotate it with @WebServlet
to specify the URL pattern for which the servlet should be invoked. Additionally, we set asyncSupported
to true to indicate that this servlet supports asynchronous processing.
Handling the Request Asynchronously
The doGet
method of the servlet obtains an AsyncContext
from the request, which allows the request to be processed asynchronously. Inside the start
method of the AsyncContext
, we define the implementation of the asynchronous task. This might involve performing I/O operations, invoking external services, or executing time-consuming computations.
Once the asynchronous task is completed, we call asyncContext.complete()
to indicate that the processing is finished.
Benefits of Using Asynchronous Servlets
-
Improved Scalability: Asynchronous servlets allow the web server to handle a larger number of concurrent connections without requiring a thread per connection. This results in improved scalability and resource utilization.
-
Reduced Thread Blocking: With asynchronous processing, threads are not blocked while waiting for time-consuming operations to complete. This prevents thread starvation and allows the server to handle more requests concurrently.
-
Enhanced Responsiveness: By offloading time-consuming tasks to be executed asynchronously, the web application can remain responsive and handle other requests without being affected by long-running operations.
-
Optimized Resource Utilization: Asynchronous servlets optimize resource utilization by using a smaller number of threads to handle a large number of concurrent requests.
Best Practices for Using Asynchronous Servlets
While asynchronous servlets offer significant performance benefits, it's important to follow best practices to ensure their effective usage.
Proper Resource Management
When performing asynchronous I/O or using external resources, it's essential to manage resources properly. This includes closing database connections, releasing file handles, and cleaning up resources after the asynchronous task completes.
Timeout Management
Implement timeout mechanisms to handle long-running asynchronous tasks. Setting reasonable timeouts ensures that resources are not tied up indefinitely and helps prevent potential resource leaks.
Error and Exception Handling
Properly handle errors and exceptions that may occur during asynchronous processing. This includes logging exceptions, notifying clients of failures, and implementing fallback mechanisms when applicable.
Testing and Monitoring
Thoroughly test asynchronous servlets under load conditions to ensure they perform as expected. Additionally, implement monitoring and logging to track the performance and behavior of asynchronous tasks in production environments.
The Bottom Line
In conclusion, asynchronous servlets provide a valuable tool for improving the performance and scalability of web applications. By embracing asynchronous processing, developers can create more responsive and efficient web applications that can handle a large number of concurrent connections without sacrificing performance.
When used effectively and in accordance with best practices, asynchronous servlets can elevate the overall user experience and contribute to the success of web applications in a highly competitive online landscape.
By understanding the benefits and implementation practices associated with asynchronous servlets, developers can leverage this powerful technology to build high-performance web applications that meet the demands of modern users.
Are you excited to implement asynchronous servlets in your Java web applications? Share your thoughts and experiences with asynchronous processing in the comments below!
Checkout our other articles