Managing Dependency Ambiguity in Java EE CDI
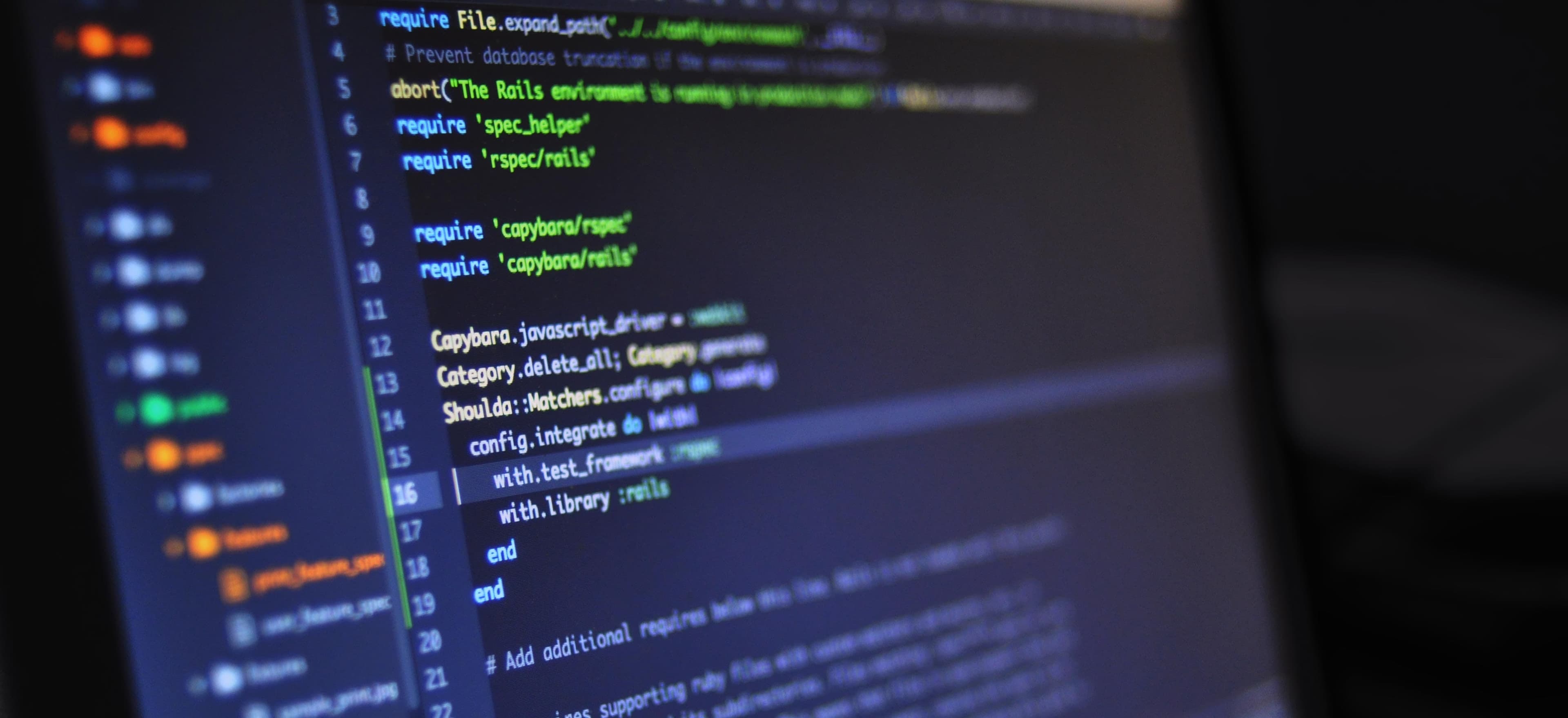
- Published on
Managing Dependency Ambiguity in Java EE CDI
In Java EE development, Contexts and Dependency Injection (CDI) is a powerful and widely used technology for managing dependencies and enabling loose coupling between components. However, as applications grow in complexity, managing dependency ambiguity becomes a critical challenge. This article will explore how to deal with dependency ambiguity in Java EE CDI and provide practical solutions for resolving conflicts.
What is Dependency Ambiguity?
Dependency ambiguity occurs when the CDI container encounters multiple beans that are eligible to inject into a specific injection point, and it is unable to determine which bean to use. This situation often arises when multiple beans implement the same interface or extend the same class, leading to ambiguity in the dependency resolution process.
Qualifiers to the Rescue
CDI provides a solution to dependency ambiguity through qualifiers. Qualifiers allow you to create custom annotations that can be applied to beans, enabling the CDI container to differentiate between multiple beans with the same type. By using qualifiers, you can specify the exact bean that should be injected at a particular injection point.
Let's consider an example where we have two implementations of a PaymentProcessor
interface, namely CreditCardPaymentProcessor
and PaypalPaymentProcessor
. To resolve the ambiguity, we can create custom qualifiers @CreditCard
and @Paypal
, and apply them to their respective implementations.
@Qualifier
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD, ElementType.TYPE, ElementType.METHOD})
public @interface CreditCard {}
@Qualifier
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD, ElementType.TYPE, ElementType.METHOD})
public @interface Paypal {}
Now, we can annotate each implementation with its corresponding qualifier:
@CreditCard
public class CreditCardPaymentProcessor implements PaymentProcessor {
// Implementation
}
@Paypal
public class PaypalPaymentProcessor implements PaymentProcessor {
// Implementation
}
And in the injection point, we can use the qualifiers to specify which implementation to inject:
@Inject
@CreditCard
PaymentProcessor paymentProcessor;
By using qualifiers, we have resolved the ambiguity and provided clear instructions to the CDI container on which bean to inject.
Ordering Beans with @Priority
In some cases, you may have multiple beans that are qualified for the same injection point, and you want to define an order of precedence among them. CDI provides the @Priority
annotation from the javax.annotation.Priority
package to address this scenario.
By applying the @Priority
annotation to the bean classes, you can define their priority order, where a lower value indicates higher priority. The CDI container will then use the priority to resolve the ambiguity and inject the bean with the highest priority.
Let's consider a scenario where we have multiple implementations of a NotificationService
interface, and we want to ensure that the EmailNotificationService
takes precedence over the SMSNotificationService
:
@Priority(1)
public class EmailNotificationService implements NotificationService {
// Implementation
}
@Priority(2)
public class SMSNotificationService implements NotificationService {
// Implementation
}
By using the @Priority
annotation, we have explicitly defined the order in which the beans should be considered for injection, effectively resolving any ambiguity.
Alternatives and @Alternative
In some cases, you may want to provide a set of alternative beans that can be used in place of the default bean for a particular injection point. CDI allows you to mark a bean as an alternative using the @Alternative
annotation, and then enable it at the deployment level using the beans.xml
configuration file.
Let's consider a scenario where we have an interface StorageService
with two implementations, LocalStorageService
and CloudStorageService
. We want to make CloudStorageService
an alternative to the default LocalStorageService
:
@Alternative
public class CloudStorageService implements StorageService {
// Implementation
}
In the beans.xml
file, we can enable the CloudStorageService
as an alternative:
<beans xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/beans_1_1.xsd"
bean-discovery-mode="all">
<alternatives>
<class>com.example.CloudStorageService</class>
</alternatives>
</beans>
By marking CloudStorageService
as an alternative and enabling it in the beans.xml
file, we have provided a clear way to handle the ambiguity, allowing the CDI container to use CloudStorageService
when it is enabled as an alternative.
Wrapping Up
In Java EE CDI, managing dependency ambiguity is crucial to ensure the reliable and predictable behavior of your application. By using qualifiers, @Priority
, and @Alternative
, you can effectively resolve ambiguity and provide clear instructions to the CDI container for dependency injection.
Understanding how to manage dependency ambiguity in CDI not only improves the robustness of your applications but also promotes better design practices by explicitly defining the relationships between beans and their injection points.
By applying these techniques, you can confidently navigate and resolve dependency ambiguity in your Java EE CDI applications, leading to more maintainable and scalable codebases.
For more in-depth information and best practices regarding CDI, consult the official Java EE documentation and the Contexts and Dependency Injection for Java EE tutorial.