Optimizing Enterprise Testing Workflows for Better Code Quality
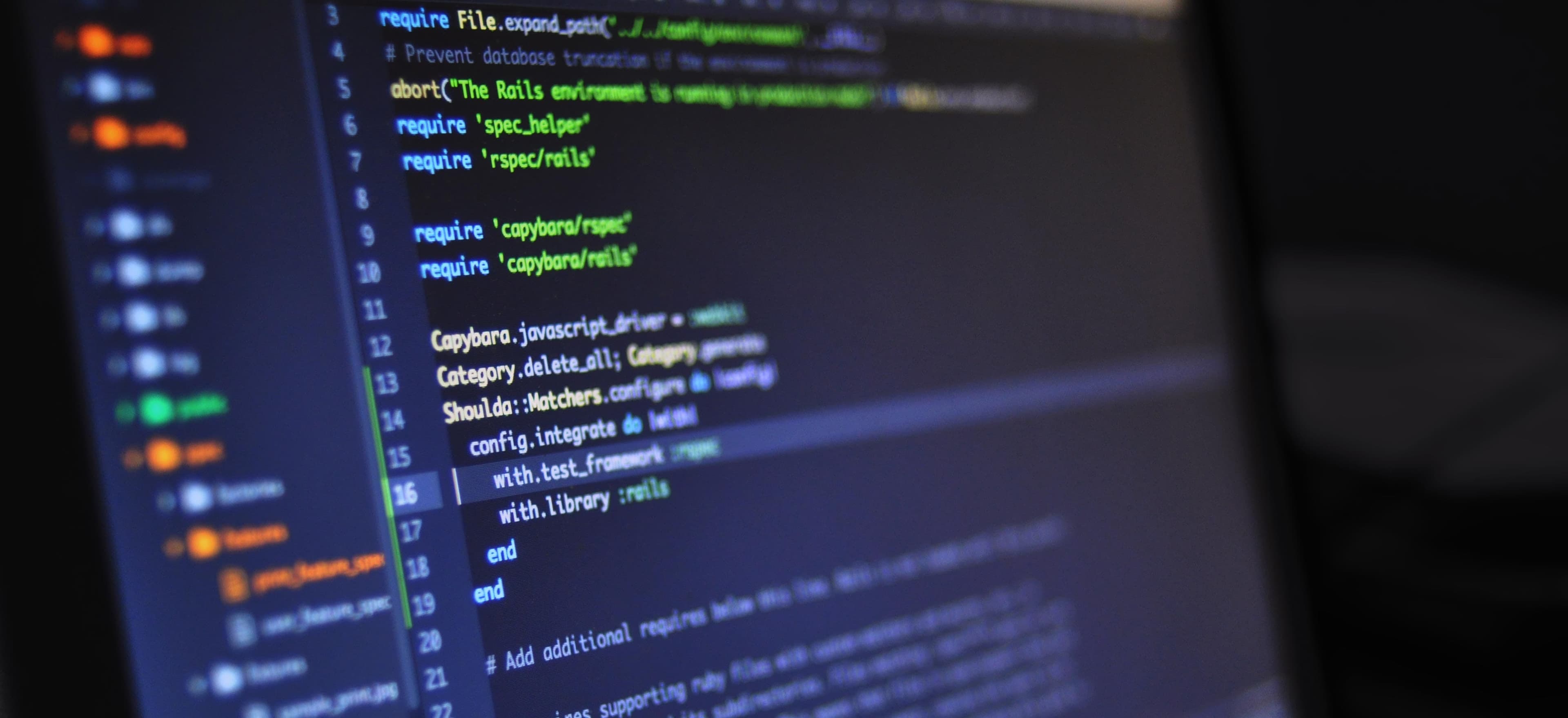
- Published on
Optimizing Enterprise Testing Workflows for Better Code Quality
As the demands for faster, more frequent software releases increase, the importance of efficient testing workflows cannot be overstated. In the enterprise environment, where complex systems and large codebases are the norm, optimizing testing workflows becomes crucial for maintaining high code quality while meeting tight release deadlines.
In this article, we will explore how Java, as one of the most popular programming languages in enterprise development, can be leveraged to optimize testing workflows, leading to better code quality and more reliable software releases.
The Role of Testing in Enterprise Software Development
In enterprise software development, testing plays a pivotal role in ensuring that the code meets the functional and non-functional requirements set by the business. Testing workflows encompass unit testing, integration testing, system testing, and acceptance testing, each serving a unique purpose in the software development lifecycle. These tests not only validate the correctness of the code but also ensure that it performs reliably under varying conditions and scales effectively with the increasing workload.
Challenges in Enterprise Testing Workflows
Despite the obvious benefits of testing, enterprise testing workflows face several challenges. These challenges include:
- Scalability: As the codebase grows, the number of tests increases, leading to longer test execution times and resource consumption.
- Complexity: Enterprise systems often have intricate dependencies and interactions, making it challenging to set up and maintain testing environments.
- Fragmented Tools: Different teams may use different testing tools, leading to fragmentation and inconsistency in the testing process.
- Integration: Coordinating testing efforts across multiple teams and systems introduces integration challenges, often resulting in delays and communication overhead.
- Feedback Loop: Delayed feedback on test results impedes the development cycle, slowing down the overall release process.
Leveraging Java for Optimizing Testing Workflows
Java provides a robust foundation for improving testing workflows in enterprise environments. Leveraging key features of the language, frameworks, and tools can address the challenges mentioned earlier, leading to more efficient and reliable testing processes.
1. Parallel Test Execution Using JUnit 5
To address the scalability challenge, JUnit 5, the latest version of the popular Java testing framework, introduces native support for parallel test execution. By parallelizing tests, enterprises can significantly reduce the overall test execution time, especially beneficial in large codebases with extensive test suites.
@Execution(ExecutionMode.CONCURRENT)
class MyTestClass {
@Test
void testMethod1() {
// Test method implementation
}
@Test
void testMethod2() {
// Test method implementation
}
}
By annotating test classes or methods with @Execution(ExecutionMode.CONCURRENT)
, JUnit 5 ensures that the tests run concurrently, utilizing multi-core processors for efficient execution.
2. Containerization for Simplified Test Environment Setup
Enterprise systems often comprise multiple interconnected components, making setting up testing environments a daunting task. Docker, a popular containerization platform, can streamline this process by encapsulating each component and its dependencies into lightweight containers.
By using Docker, teams can create consistent testing environments that mirror production setups, reducing the discrepancies between development, testing, and production environments. This approach leads to a more reliable testing process and fewer environment-related issues.
3. Unified Testing Tools with Maven
To tackle the fragmented tools challenge, Maven, a powerful build automation tool predominantly used for Java projects, can serve as a unifying force for testing. Maven provides a standardized way to define and execute tests, irrespective of the testing framework used (e.g., JUnit, TestNG).
By configuring Maven to execute tests as part of the build lifecycle, teams can ensure that testing is an integral part of the development process, promoting consistency and uniformity across teams and projects.
4. Continuous Integration with Jenkins
Jenkins, an open-source automation server, plays a vital role in addressing the integration challenge by facilitating continuous integration and delivery. By orchestrating the build, test, and deployment processes, Jenkins enables seamless integration of code changes, automated testing, and feedback generation.
Integrating Jenkins with version control systems such as Git allows for automated triggering of test runs upon code commits, thus providing immediate feedback to developers and reducing the integration overhead.
5. Feedback Loop Improvement with Test Reports
Delayed feedback can significantly impede the release process. Java testing frameworks like JUnit and TestNG provide comprehensive test reporting capabilities, including test results, code coverage, and failure analysis.
By leveraging these capabilities and integrating them with build pipelines, teams can ensure that actionable feedback on test results is readily available, empowering developers to address issues promptly, and fostering a shorter feedback loop.
In conclusion, optimizing testing workflows in enterprise Java development is vital for maintaining high code quality, meeting release deadlines, and delivering reliable software. By leveraging Java's features, along with complementary tools and frameworks, enterprises can address scalability, complexity, tool fragmentation, integration, and feedback challenges, leading to more efficient testing processes and improved code quality.