Overcoming Communication Challenges in Microservices
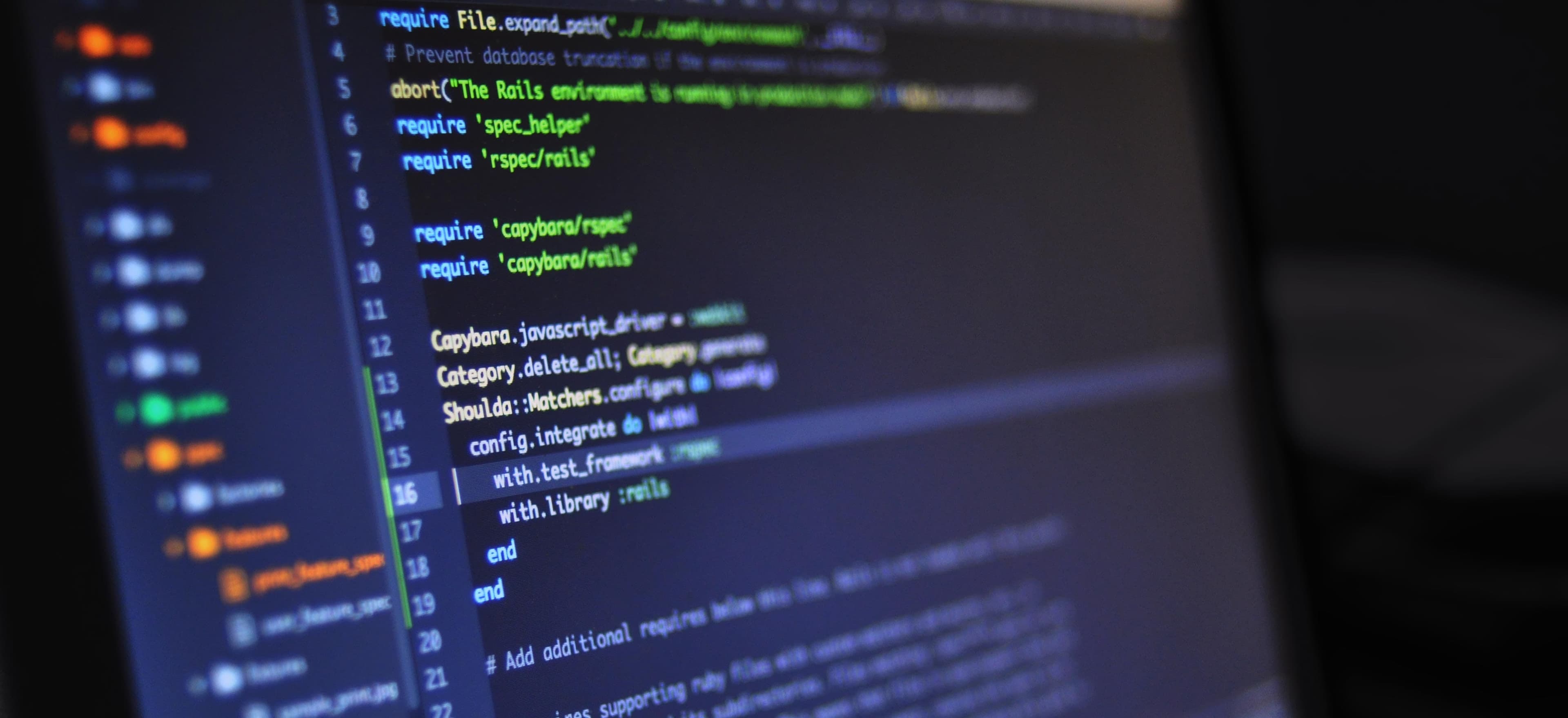
- Published on
Overcoming Communication Challenges in Microservices
Microservices architecture has gained popularity due to its ability to break down large, complex applications into smaller, more manageable services. However, as the number of services grows, so do the communication challenges. In this article, we will explore some common communication challenges in microservices and discuss strategies to overcome them using Java.
Challenge 1: Service Discovery
In a microservices architecture, services need to discover and communicate with each other. Traditionally, this was done using a static configuration or a centralized service registry. However, as the number of services grows, managing this becomes complex.
Solution: Use Service Discovery with Eureka
Eureka is a service discovery solution that provides a REST-based service that is primarily used in the AWS cloud for locating services for the purpose of load balancing and failover of middle-tier servers.
Example Code:
@SpringBootApplication
@EnableEurekaServer
public class EurekaApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaApplication.class, args);
}
}
In this example, we utilize Spring Boot and @EnableEurekaServer
to create a Eureka server, allowing services to register and discover each other dynamically.
Challenge 2: Asynchronous Communication
Microservices often need to communicate with each other asynchronously to improve performance and scalability. This introduces challenges in ensuring message reliability and handling communication failures.
Solution: Use Apache Kafka for Event-Driven Communication
Apache Kafka is a distributed streaming platform that can be used for building real-time data pipelines and streaming applications. It provides features like fault tolerance, high throughput, and horizontal scalability.
Example Code:
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
producer.send(new ProducerRecord<>("topic1", "key1", "value1"));
In this example, we configure a Kafka producer to send messages to a topic, enabling asynchronous communication between microservices.
Challenge 3: Circuit Breaker and Fault Tolerance
In microservices architecture, services are prone to failures. When a service fails or becomes unresponsive, it can cause cascading failures across dependent services.
Solution: Use Hystrix for Circuit Breaker and Fault Tolerance
Hystrix is a latency and fault tolerance library designed to isolate points of access to remote systems, services, and 3rd party libraries, stop cascading failure, and enable resilience in complex distributed systems where failure is inevitable.
Example Code:
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String getRemoteData() {
// Make remote service call
}
public String fallbackMethod() {
// Fallback logic
}
In this example, we use the @HystrixCommand
annotation to create a circuit breaker around the getRemoteData
method, providing a fallback mechanism if the remote service call fails.
To Wrap Things Up
In conclusion, communication challenges in microservices can be overcome by leveraging modern technologies and frameworks. It's important to carefully design the communication between microservices to ensure reliability, fault tolerance, and scalability. By using tools such as Eureka for service discovery, Apache Kafka for asynchronous communication, and Hystrix for fault tolerance, Java developers can build robust microservices architectures that can handle the complexities of communication in a distributed system.
By implementing these solutions, Java developers can effectively address the communication challenges in microservices and build resilient and scalable systems.
Checkout our other articles