Optimizing Large MQ Deployments for Efficiency
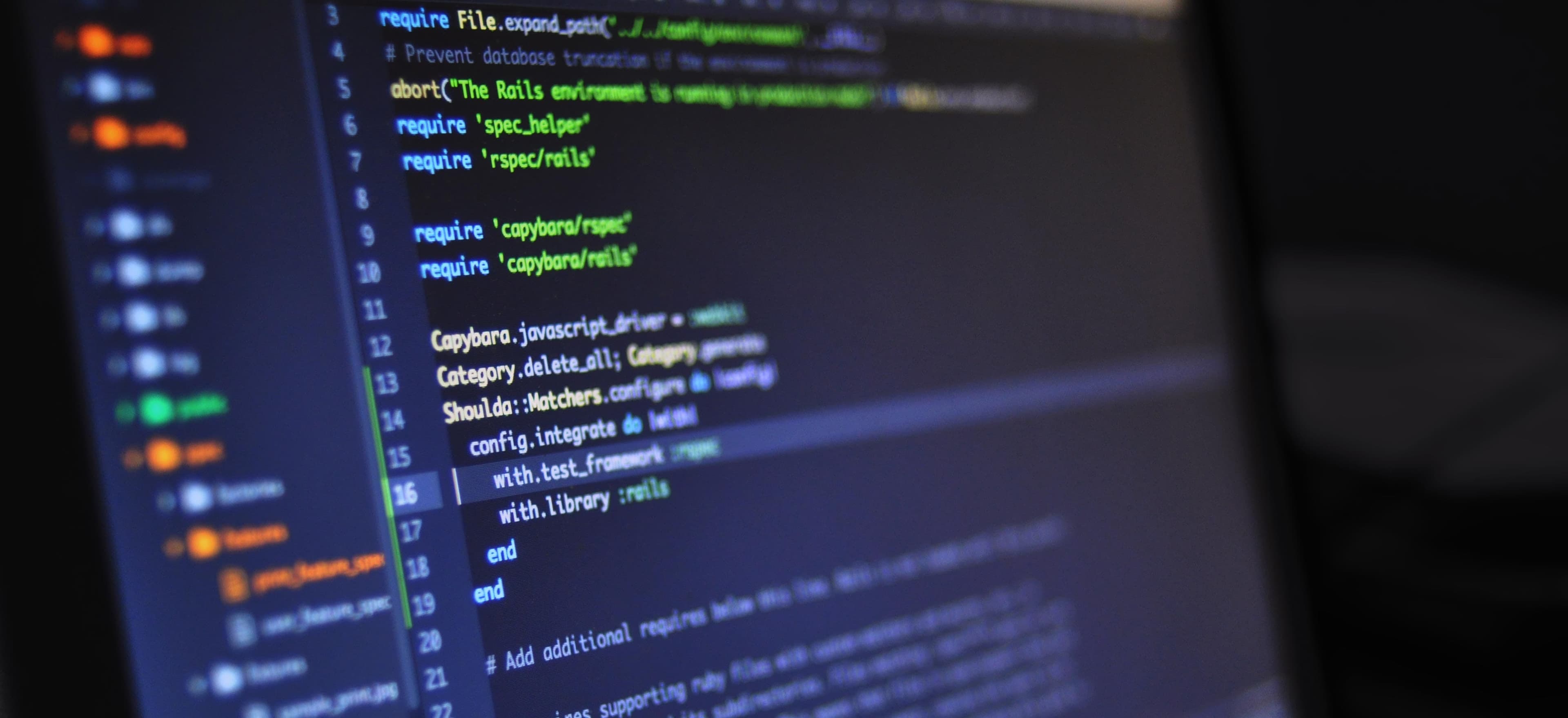
- Published on
Optimizing Large MQ Deployments for Efficiency
When it comes to handling large volumes of messages in a Java application, optimizing the use of IBM MQ (formerly known as WebSphere MQ) becomes crucial to ensure efficiency and high performance. In this article, we will explore some best practices and techniques for optimizing large MQ deployments in Java.
1. Use Asynchronous Message Delivery
One of the key principles for optimizing MQ deployments is to leverage asynchronous message delivery. By using asynchronous message consumption, a Java application can continue its processing while waiting for MQ messages. This approach prevents the application from getting blocked, leading to better resource utilization and improved overall performance.
Here's how you can consume messages asynchronously using the IBM MQ Java client:
// Create a new instance of MQQueueManager
MQQueueManager queueManager = new MQQueueManager("QMGR");
// Access a queue for reading
MQQueue queue = queueManager.accessQueue("QUEUE_NAME", MQC.MQOO_INPUT_SHARED);
// Set up the message options
MQGetMessageOptions messageOptions = new MQGetMessageOptions();
messageOptions.options = MQC.MQGMO_NO_WAIT | MQC.MQGMO_ASYNC_CONSUME;
// Create a new MQMessage to hold the incoming message
MQMessage incomingMessage = new MQMessage();
// Get the message from the queue asynchronously
queue.get(incomingMessage, messageOptions);
// Process the incoming message
// ...
// Close the queue and queue manager
queue.close();
queueManager.disconnect();
In the above code snippet, MQC.MQGMO_ASYNC_CONSUME
enables asynchronous message consumption, allowing the application to continue its processing without getting blocked.
2. Batch Processing of Messages
When dealing with large volumes of messages, it's beneficial to implement batch processing to reduce the overhead of individual message handling. By fetching and processing messages in batches, the application can maximize throughput and minimize the impact of network latency and processing overhead.
Here's an example of batch processing using the IBM MQ Java client:
// Create a new instance of MQQueueManager
MQQueueManager queueManager = new MQQueueManager("QMGR");
// Access a queue for reading
MQQueue queue = queueManager.accessQueue("QUEUE_NAME", MQC.MQOO_INPUT_SHARED);
// Set up the message options
MQGetMessageOptions messageOptions = new MQGetMessageOptions();
messageOptions.options = MQC.MQGMO_NO_WAIT;
// Create an array to hold the incoming messages
MQMessage[] batchMessages = new MQMessage[BATCH_SIZE];
for (int i = 0; i < BATCH_SIZE; i++) {
// Create a new MQMessage to hold the incoming message
batchMessages[i] = new MQMessage();
// Get the message from the queue
queue.get(batchMessages[i], messageOptions);
// Process the incoming message
// ...
}
// Close the queue and queue manager
queue.close();
queueManager.disconnect();
In this code snippet, BATCH_SIZE
determines the number of messages to be processed in a batch. By fetching multiple messages in one go, the application can minimize the overhead of individual message retrievals and processing.
3. Connection Pooling
Efficient management of MQ connections is essential for optimizing performance, especially in large deployments with high message throughput. Connection pooling helps reduce the overhead of establishing and tearing down connections, leading to better resource utilization and improved scalability.
Using the MQConnectionFactory
provided by IBM MQ, you can easily set up a connection pool in your Java application.
Here's an example of setting up an MQ connection pool:
// Create a connection factory
MQConnectionFactory connectionFactory = new MQConnectionFactory();
// Set the necessary attributes (host, port, queue manager, etc.)
connectionFactory.setHostName("HOST_NAME");
connectionFactory.setPort(1414);
connectionFactory.setQueueManager("QMGR");
// ...
// Set the maximum number of connections in the pool
connectionFactory.setMaxConnections(MAX_CONNECTIONS);
// Get a connection from the pool
MQConnection connection = (MQConnection) connectionFactory.createConnection();
// Use the connection for sending or receiving messages
// ...
// Close the connection (returns it to the pool)
connection.close();
In this example, MAX_CONNECTIONS
determines the maximum number of connections allowed in the pool. By reusing connections from the pool, the application can avoid the overhead of creating new connections for each message operation.
Bringing It All Together
Optimizing large MQ deployments for efficiency in Java applications is essential for achieving high performance and scalability. By leveraging asynchronous message delivery, implementing batch processing, and utilizing connection pooling, developers can effectively manage high message volumes while minimizing resource utilization. Incorporating these best practices will result in a well-optimized and efficient MQ deployment for Java applications.
In conclusion, optimizing large MQ deployments in Java requires a combination of asynchronous message delivery, batch processing, and connection pooling to achieve high performance and scalability.
By adopting these best practices, Java developers can effectively manage high message volumes while minimizing resource utilization, resulting in a well-optimized and efficient MQ deployment.
Optimizing large MQ deployments is a critical aspect of developing high-performance Java applications, and by following these best practices, developers can take full advantage of IBM MQ's capabilities while ensuring efficient message processing and delivery.
Checkout our other articles