Improving SMS Notification Systems for Efficient Communication
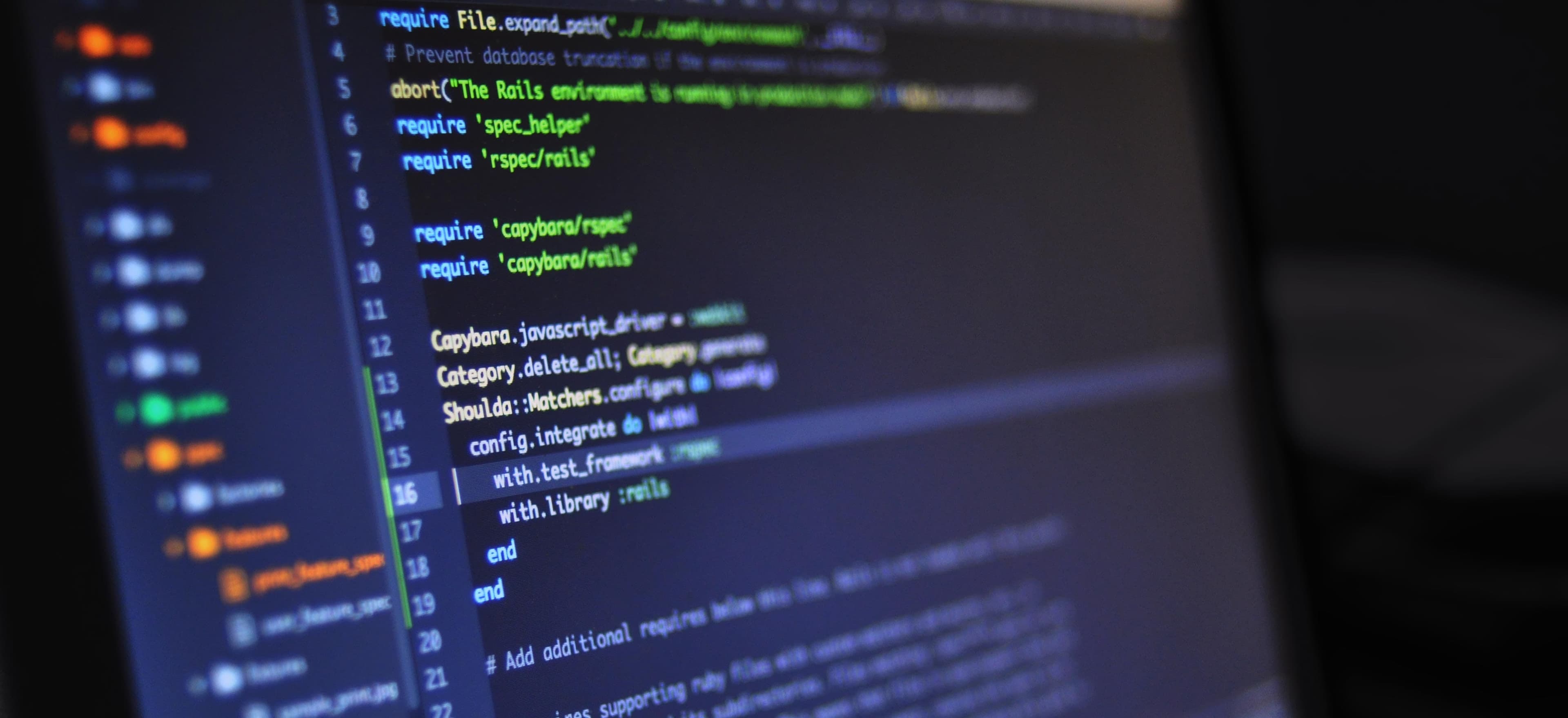
- Published on
Enhancing SMS Notification Systems with Java
In today's fast-paced world, effective communication is essential for businesses and organizations to thrive. Short Message Service (SMS) notifications have become a vital tool for reaching customers, employees, and stakeholders. However, as the volume of SMS notifications increases, so does the need for efficient and reliable notification systems.
Java, with its robust features and flexibility, offers a solid foundation for building and improving SMS notification systems. In this article, we will explore how Java can be leveraged to enhance SMS notification systems, ensuring seamless and reliable communication.
Understanding the Importance of SMS Notification Systems
Before delving into the technical aspects, it's crucial to understand the significance of SMS notification systems in today's business landscape. SMS notifications provide a direct and immediate channel for delivering important information, such as order confirmations, appointment reminders, security alerts, and more. This directness makes SMS a powerful tool for engaging and communicating with customers and stakeholders.
In the context of business operations, SMS notifications can streamline communication processes, leading to improved customer satisfaction, operational efficiency, and overall business performance. However, to fully unlock the potential of SMS notifications, a well-structured and efficient system is imperative.
Leveraging Java for Building Robust SMS Notification Systems
Java, as a versatile and widely-used programming language, offers a multitude of tools and libraries for developing SMS notification systems. From handling API integrations to managing SMS templates, Java provides the necessary capabilities to create reliable and scalable notification systems.
Integrating SMS Gateways with Java
One of the fundamental aspects of enhancing SMS notification systems is integrating with SMS gateways. SMS gateways serve as intermediaries between the application and the mobile network, facilitating the delivery of SMS messages. With Java, developers can seamlessly integrate various SMS gateway APIs, such as Twilio, Nexmo, or Plivo, into their applications.
Let's take a look at how Java can be used to integrate with the Twilio API for sending SMS notifications:
import com.twilio.Twilio;
import com.twilio.rest.api.v2010.account.Message;
import com.twilio.type.PhoneNumber;
public class TwilioSMS {
// Twilio account credentials
public static final String ACCOUNT_SID = "your_account_sid";
public static final String AUTH_TOKEN = "your_auth_token";
public static void sendSMS(String from, String to, String body) {
Twilio.init(ACCOUNT_SID, AUTH_TOKEN);
Message message = Message.creator(
new PhoneNumber(to),
new PhoneNumber(from),
body)
.create();
System.out.println("SMS sent: " + message.getSid());
}
}
In the above code snippet, we are leveraging the Twilio API to send an SMS message. By providing the required credentials and utilizing the Twilio library, Java enables seamless integration with the Twilio SMS gateway.
Implementing SMS Templates and Personalization
Another key aspect of an effective SMS notification system is the ability to use templates and personalize messages for different recipients. Java provides the flexibility to implement and manage SMS templates, allowing for consistent messaging across various notification types.
Let's consider a scenario where we want to send personalized appointment reminders to customers using Java:
public class AppointmentReminder {
public static void sendAppointmentReminder(String recipient, Date appointmentDate) {
String messageTemplate = "Hi %s! This is a friendly reminder of your appointment on %s. We look forward to seeing you.";
String personalizedMessage = String.format(messageTemplate, recipient, appointmentDate.toString());
// Call SMS gateway integration to send the personalized message
TwilioSMS.sendSMS("your_twilio_number", recipient, personalizedMessage);
}
}
In this example, we are leveraging Java's string formatting capabilities to personalize the appointment reminder message before sending it via the integrated SMS gateway. This level of customization enhances the effectiveness of the notification system, leading to improved customer engagement.
Ensuring Reliability and Error Handling
Reliability is paramount when it comes to SMS notification systems. Java offers robust error handling mechanisms, allowing developers to implement failover strategies and handle network issues effectively.
By incorporating retry mechanisms and error logging, Java-based SMS notification systems can mitigate potential delivery failures and provide insights into any issues that arise during the notification process.
Closing the Chapter
In conclusion, Java serves as a powerful platform for enhancing SMS notification systems, enabling developers to integrate with SMS gateways, implement personalized messaging, and ensure the reliability of communication channels. By leveraging Java's capabilities, businesses and organizations can establish efficient and effective SMS notification systems that contribute to improved communication and operational excellence.
As the demand for streamlined and reliable communication continues to grow, the role of Java in optimizing SMS notification systems becomes increasingly significant. Embracing Java's strengths in building robust and scalable notification systems can undoubtedly elevate the communication strategies of modern businesses.
In the relentless pursuit of efficient communication, Java emerges as a formidable ally in the realm of SMS notification systems. As businesses strive to connect with their audience in a meaningful and timely manner, Java remains a steadfast companion in the journey towards seamless communication.
In the realm of SMS notification systems, the fusion of Java's versatility and the imperative need for effective communication propels us towards an era of unparalleled connectivity and engagement.
By harnessing the power of Java, businesses can transcend communication barriers and orchestrate harmonious dialogues with their audience, setting the stage for a symphony of seamless interactions and enriched relationships.
In the symphony of communication, Java stands as a conductor, orchestrating the seamless flow of SMS notifications with precision and finesse.
Remember, the effectiveness of SMS notification systems lies not only in their technical adeptness but also in their ability to forge meaningful connections and deliver value to recipients.
So, as we continue to navigate the ever-evolving landscape of communication, let us harness the capabilities of Java to craft SMS notification systems that resonate with clarity and purpose.