Optimizing AWT Robot with Custom DSL
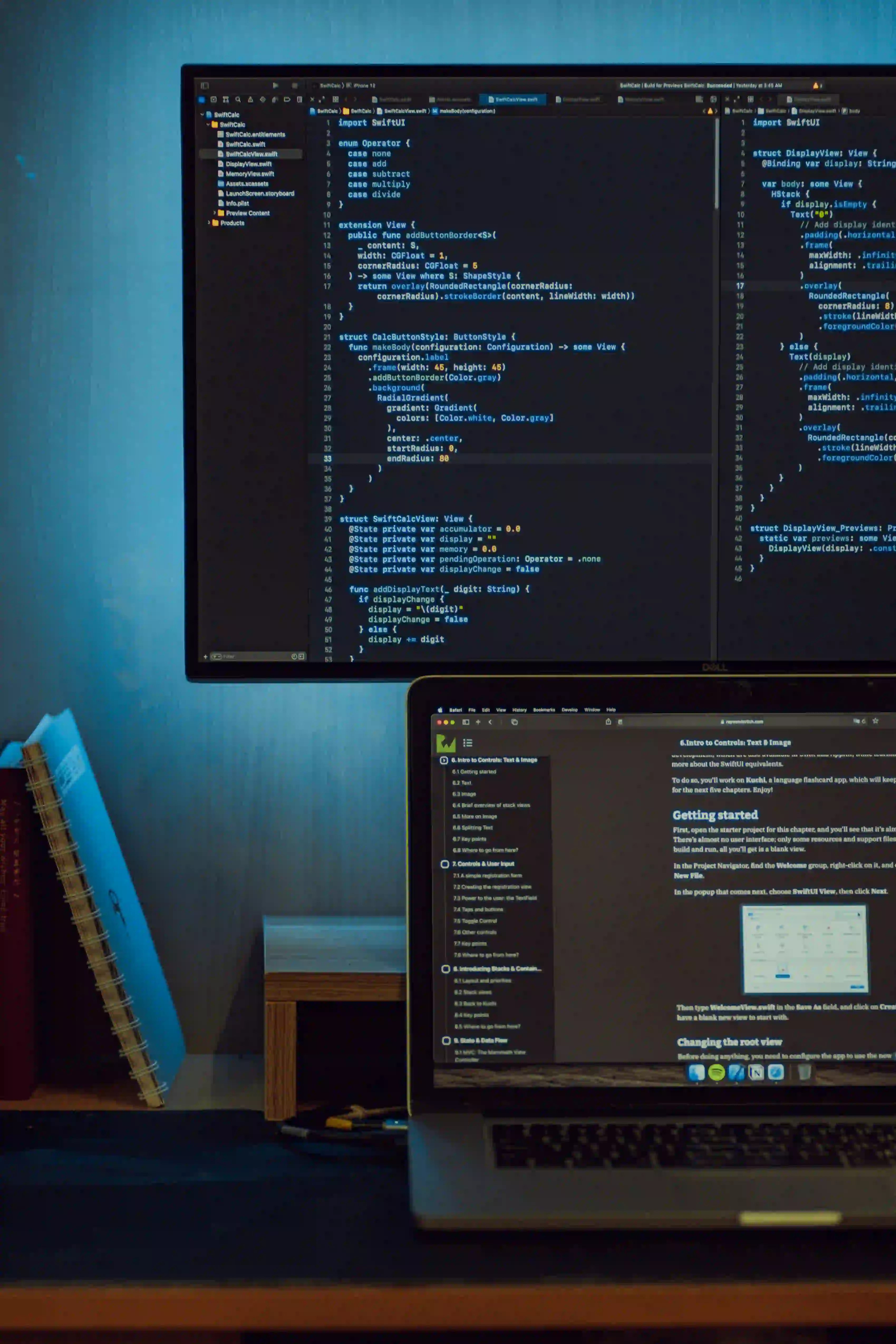
Optimizing AWT Robot with Custom DSL
In this article, we'll explore how to optimize AWT Robot with a custom DSL (Domain Specific Language) in Java. AWT (Abstract Window Toolkit) is a package used to develop graphical user interfaces (GUIs) in Java. The Robot class in AWT provides low-level control over mouse and keyboard inputs, which can be useful for automating tasks, testing GUI applications, and other scenarios where programmatic control over input devices is required.
However, working directly with the AWT Robot class can be verbose and error-prone, especially when performing complex sequences of input actions. To address these issues, we can create a custom DSL that encapsulates common input patterns and provides a more expressive and readable API for interacting with the Robot class.
Understanding AWT Robot
Before diving into the custom DSL, let's briefly review the basics of AWT Robot. The Robot class allows us to generate native system input events for the keyboard and mouse, such as key presses, mouse movements, and mouse clicks. Here's a simple example of using AWT Robot to move the mouse to a specific screen coordinate:
Robot robot = new Robot();
robot.mouseMove(100, 100);
In this example, we create a new Robot instance and then use it to move the mouse to the (100, 100) screen coordinates. While this code snippet is straightforward, more complex interactions, such as simulating keyboard input or performing multi-step mouse actions, can quickly become cumbersome and hard to maintain.
Creating a Custom DSL
To simplify the usage of AWT Robot, we can create a custom DSL tailored to our specific needs. A DSL is a small, domain-specific language designed for a particular task or problem domain. In our case, the DSL will provide a higher-level, more expressive interface for interacting with the AWT Robot.
Let's create a simplified example of a DSL for mouse actions. We'll define a fluent API for chaining mouse-related operations and abstracting away the lower-level details of the Robot class. We will use method chaining to build a sequence of actions, making the code more readable and concise.
public class CustomRobot {
private Robot robot;
public CustomRobot() throws AWTException {
this.robot = new Robot();
}
public CustomRobot moveMouseTo(int x, int y) {
robot.mouseMove(x, y);
return this;
}
public CustomRobot click() {
robot.mousePress(InputEvent.BUTTON1_DOWN_MASK);
robot.mouseRelease(InputEvent.BUTTON1_DOWN_MASK);
return this;
}
// Additional DSL methods for other mouse actions can be added here
}
In this simplified example, we've created a CustomRobot class that encapsulates the AWT Robot instance and provides methods for common mouse actions. The moveMouseTo method moves the mouse to the specified coordinates, and the click method simulates a left mouse button click. By chaining these methods together, we can create a readable sequence of mouse actions.
Using the Custom DSL
Now that we have our custom DSL defined, let's see how we can use it to interact with AWT Robot in a more expressive manner.
public class CustomRobotExample {
public static void main(String[] args) {
try {
CustomRobot customRobot = new CustomRobot();
customRobot.moveMouseTo(200, 200).click().moveMouseTo(300, 300).click();
} catch (AWTException e) {
e.printStackTrace();
}
}
}
In this example, we create an instance of CustomRobot and then use its DSL methods to move the mouse to (200, 200), click, move the mouse to (300, 300), and click again. The resulting code reads almost like a series of English sentences, making it much easier to understand and maintain compared to the low-level Robot API.
Benefits of Using a Custom DSL
Using a custom DSL for interacting with AWT Robot offers several benefits:
-
Readability: The DSL provides a more readable and expressive interface, making it easier to understand the sequence of input actions without delving into the implementation details of AWT Robot.
-
Maintainability: By encapsulating the low-level Robot interactions within the DSL, we can isolate changes to the AWT Robot API and modify the DSL implementation without affecting the rest of the codebase.
-
Abstraction: The DSL allows us to abstract away the complexities of AWT Robot, providing a higher-level interface that focuses on the specific domain of input actions.
Considerations and Limitations
While a custom DSL can greatly improve the usability of AWT Robot, there are a few considerations and limitations to keep in mind:
-
Learning Curve: Introducing a custom DSL may involve a learning curve for developers who are unfamiliar with its syntax and semantics. Proper documentation and examples are essential for onboarding new users.
-
DSL Flexibility: The design of the custom DSL should strike a balance between expressiveness and simplicity. Overly complex DSLs can introduce cognitive overhead, while overly simplistic DSLs may not cover all use cases.
-
Performance Overhead: Depending on the complexity of the DSL implementation, there may be a slight performance overhead compared to directly using the AWT Robot API. However, the tradeoff in developer productivity and code readability often outweighs this concern.
Wrapping Up
In this article, we've explored how to optimize AWT Robot with a custom DSL in Java. By creating a domain-specific language tailored to interacting with AWT Robot, we can significantly improve the readability, maintainability, and abstraction of input actions in GUI automation and testing scenarios. While there are considerations and limitations to be aware of, the benefits of using a custom DSL make it a compelling approach for working with AWT Robot in Java.
By leveraging the power of custom DSLs, developers can streamline their interaction with low-level APIs, such as AWT Robot, and create more expressive and maintainable code. This approach contributes to improved developer productivity and a better overall user experience.
For further reading on AWT and Robot class, consider the official Java documentation on AWT and Robot.
Remember, when working with a custom DSL, prioritize clarity and simplicity to ensure that the DSL enhances, rather than complicates, the codebase.