Handling InterruptedException in Java Multithreading
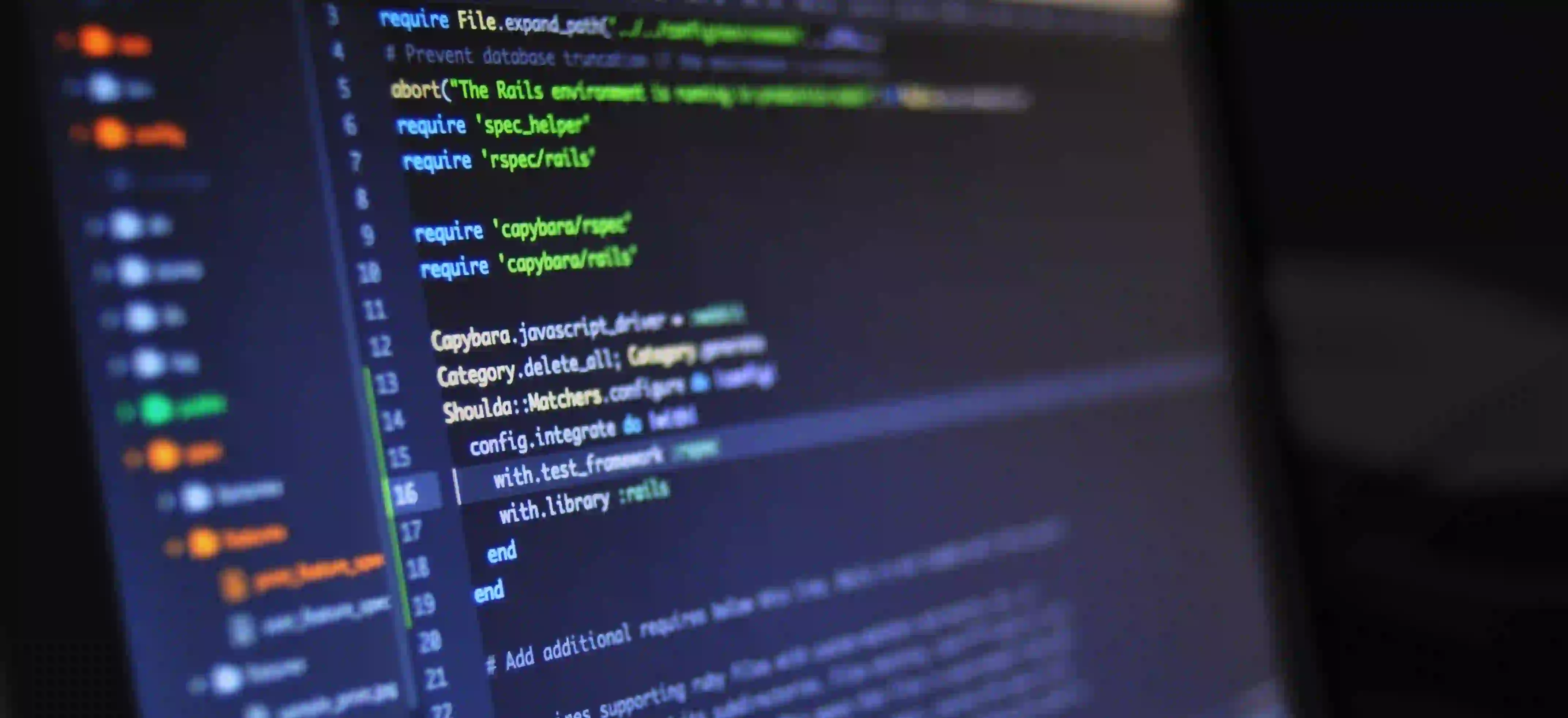
Understanding InterruptedException in Java Multithreading
When working with concurrent programming in Java, it's crucial to comprehend how to handle InterruptedException
. This exception is commonly encountered when dealing with multithreading, and mastering its handling is vital for creating stable and responsive applications.
What is InterruptedException?
In Java, InterruptedException
is a checked exception that is thrown when a thread is interrupted by another thread while it's in a waiting, sleeping, or blocking state. The interruption serves as a way to communicate between threads, allowing one thread to request that another thread should halt its current operation.
Why is InterruptedException important?
Handling InterruptedException
is crucial to ensure that our multithreaded applications can gracefully respond to interrupt requests and maintain stability. Ignoring or mishandling this exception can lead to unpredictable behavior and might even cause our application to become unresponsive.
Handling InterruptedException in Java
Let's discuss some best practices for handling InterruptedException
in Java multithreading.
1. Handling InterruptedException with an Interrupted Flag
public class ThreadExample implements Runnable {
private volatile boolean isInterrupted = false;
@Override
public void run() {
while (!isInterrupted) {
// Perform thread's task
}
}
public void stopThread() {
isInterrupted = true;
}
}
In this example, we are using a volatile boolean flag isInterrupted
to signal the thread when it should stop its execution. When another thread wants to interrupt this thread, it can call the stopThread
method. This approach ensures that the thread can gracefully exit its operation.
2. Handling InterruptedException by Rethrowing
@Override
public void run() {
try {
while (!Thread.currentThread().isInterrupted()) {
// Perform thread's task
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Preserve the interrupt status
// Re-assert the interrupt by rethrowing the exception
throw new RuntimeException("Thread interrupted", e);
}
}
In this example, if the thread is interrupted, we rethrow the exception after preserving the interrupt status. This allows the interruption to propagate up the call stack, ensuring that the higher levels of the application are aware of the interruption and can take appropriate action.
3. Handling InterruptedException by Clean-up and Graceful Exit
@Override
public void run() {
while (!Thread.currentThread().isInterrupted()) {
try {
// Perform thread's task
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Preserve the interrupt status
// Clean up resources and gracefully exit
// e.g., close open connections, release locks, etc.
break;
}
}
}
This approach handles the InterruptedException
by performing necessary clean-up operations and then gracefully exiting the thread's execution. It ensures that resources are properly released and the thread terminates in a controlled manner.
Key Takeaways
In Java multithreading, handling InterruptedException
is essential for building robust and responsive applications. By understanding the best practices for handling this exception, we can create multithreaded programs that gracefully respond to interrupt requests and maintain stability.
Remember, ignoring InterruptedException
can lead to thread leaks, resource contention, and unpredictable behavior. Therefore, it's crucial to handle this exception appropriately to ensure the reliability of your multithreaded applications.
By applying the discussed techniques, you can write Java multithreaded code that handles InterruptedException
effectively, promoting application stability and responsiveness.
To deepen your understanding, consider exploring the official Java documentation on InterruptedException and experiment with the provided code examples.