Optimizing Relational Database Performance for JMeter Load Testing
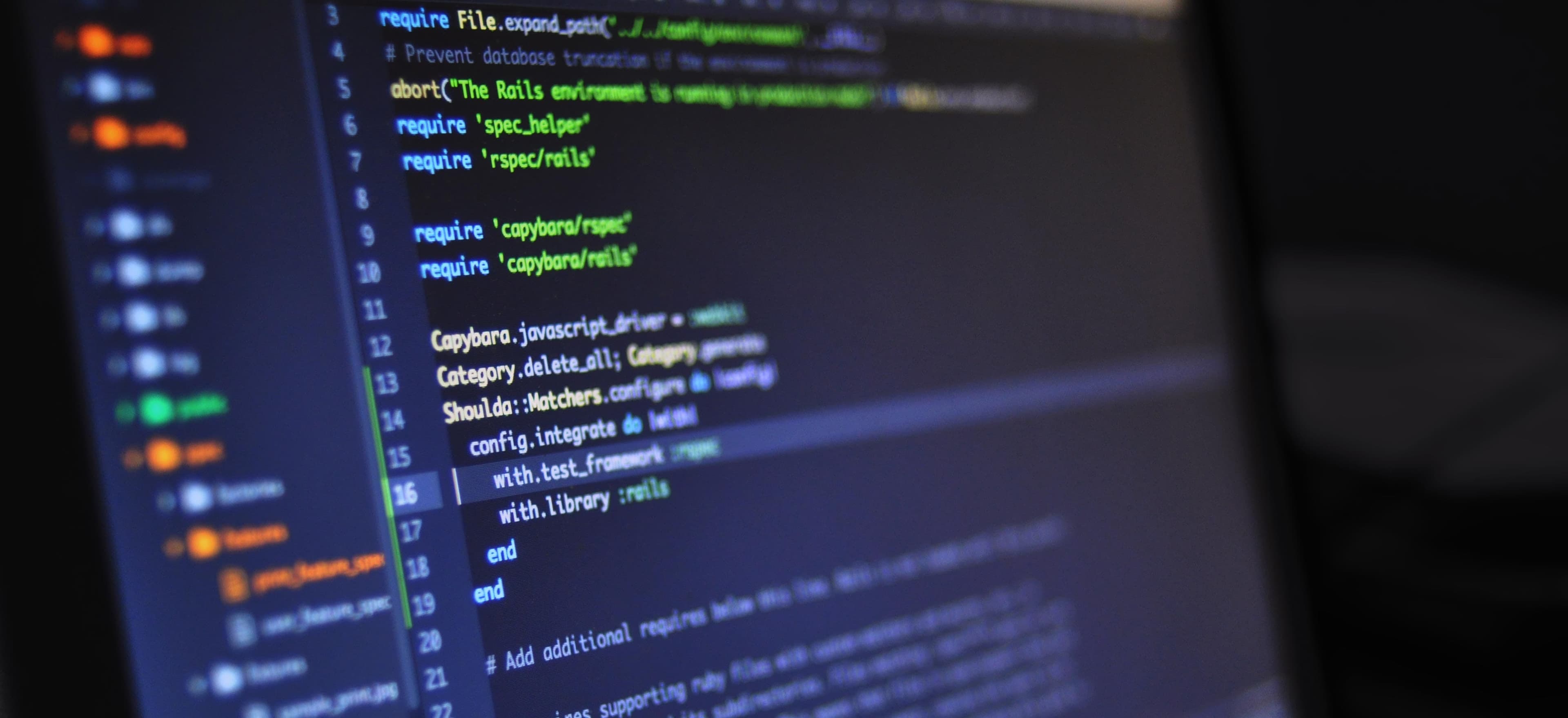
- Published on
Understanding the Importance of Optimizing Relational Database Performance for JMeter Load Testing
When it comes to evaluating the performance of a system, load testing is an indispensable tool. Apache JMeter is a popular open-source tool used for load testing, and it provides robust support for testing the performance of databases. However, when conducting load testing with JMeter, it’s crucial to pay special attention to the performance of the underlying database to ensure accurate and reliable test results. In this article, we will delve into the process of optimizing relational database performance specifically for JMeter load testing, focusing on Java-based applications.
Why Optimizing Database Performance is Crucial for Load Testing
During load testing, JMeter simulates multiple users interacting with a system simultaneously. This places a significant load on the application and its associated database. As a result, any inefficiencies in the database performance can have a cascading effect, impacting the overall test results and potentially leading to inaccurate conclusions about the system’s performance under real-world conditions.
Optimizing the database performance for load testing is essential to ensure that the database can handle the expected load efficiently, without becoming a bottleneck that skews the test results. By addressing database performance optimization, you can gain more accurate insights into how the system would perform under concurrent user interactions in a production environment.
Leveraging the Power of Java for JMeter Load Testing
Java is widely used for developing enterprise applications, and its compatibility with JMeter makes it an ideal choice for load testing applications that rely on relational databases. When optimizing database performance for JMeter load testing, leveraging Java’s capabilities can provide a solid foundation for efficient and effective testing.
Additionally, Java offers a multitude of libraries and frameworks that can be utilized to connect to relational databases, execute queries, and process the retrieved data. Leveraging these features allows for the seamless integration of database operations within JMeter test plans, ensuring that the database is appropriately stressed during load testing.
Tips for Optimizing Relational Database Performance
1. Indexing Strategy
Proper indexing of database tables is crucial for efficient query processing, especially when dealing with large datasets. By analyzing the queries executed during load testing, you can identify the frequently accessed columns and design appropriate indexes to expedite query execution. Utilizing tools like the Hibernate Query Analyzer can aid in identifying potential areas for indexing optimization.
// Example of creating an index on a database table using JDBC
String createIndexQuery = "CREATE INDEX idx_username ON users(username)";
statement.execute(createIndexQuery);
2. Connection Pooling
Establishing a connection to the database can be a resource-intensive task. Utilizing connection pooling mechanisms such as HikariCP or Apache DBCP can significantly reduce the overhead of creating new database connections for each user thread in JMeter, leading to improved performance and scalability.
// Example of configuring HikariCP connection pool
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("username");
config.setPassword("password");
config.setMaximumPoolSize(20);
HikariDataSource dataSource = new HikariDataSource(config);
3. Query Optimization
Analyze the SQL queries executed by the application and ensure they are optimized for performance. Strategies such as utilizing proper join techniques, limiting the result set with pagination, and avoiding unnecessary database roundtrips can significantly enhance the efficiency of query execution during load testing.
// Example of optimizing a query using pagination
String query = "SELECT * FROM products LIMIT 50 OFFSET 100";
statement.execute(query);
4. Data Caching
Introduce caching mechanisms to reduce the frequency of database reads, especially for static or infrequently updated data. Libraries like Ehcache or Redisson can be employed to cache query results or frequently accessed data, reducing the load on the database and improving overall system performance.
// Example of caching using Ehcache
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withCache("myCache",
NewCacheConfigurationBuilder.newCacheConfigurationBuilder(Long.class, String.class, ResourcePoolsBuilder.heap(10)))
.build(true);
Cache<Long, String> myCache = cacheManager.getCache("myCache", Long.class, String.class);
myCache.put(1L, "example");
String value = myCache.get(1L);
Final Thoughts
In conclusion, optimizing relational database performance is paramount when conducting load testing with JMeter, especially for Java-based applications. By focusing on areas such as indexing, connection pooling, query optimization, and data caching, you can ensure that the database can efficiently handle the simulated load, providing accurate insights into the system’s performance under real-world conditions. Leveraging Java’s capabilities and integrating best practices for database performance optimization will contribute to more reliable and informative load test results.
By following these optimization strategies and leveraging the power of Java, you can ensure that your load testing efforts with JMeter yield accurate and actionable insights, enabling you to fine-tune your applications for optimal performance in production environments.
Remember, a robust and well-optimized database is the backbone of accurate load testing with JMeter. Happy load testing!
Related links for further reading:
- Apache JMeter
- Hibernate Query Analyzer
- HikariCP
- Apache DBCP
- Ehcache
- Redisson