"Handling Exception in Apache Camel with Groovy"
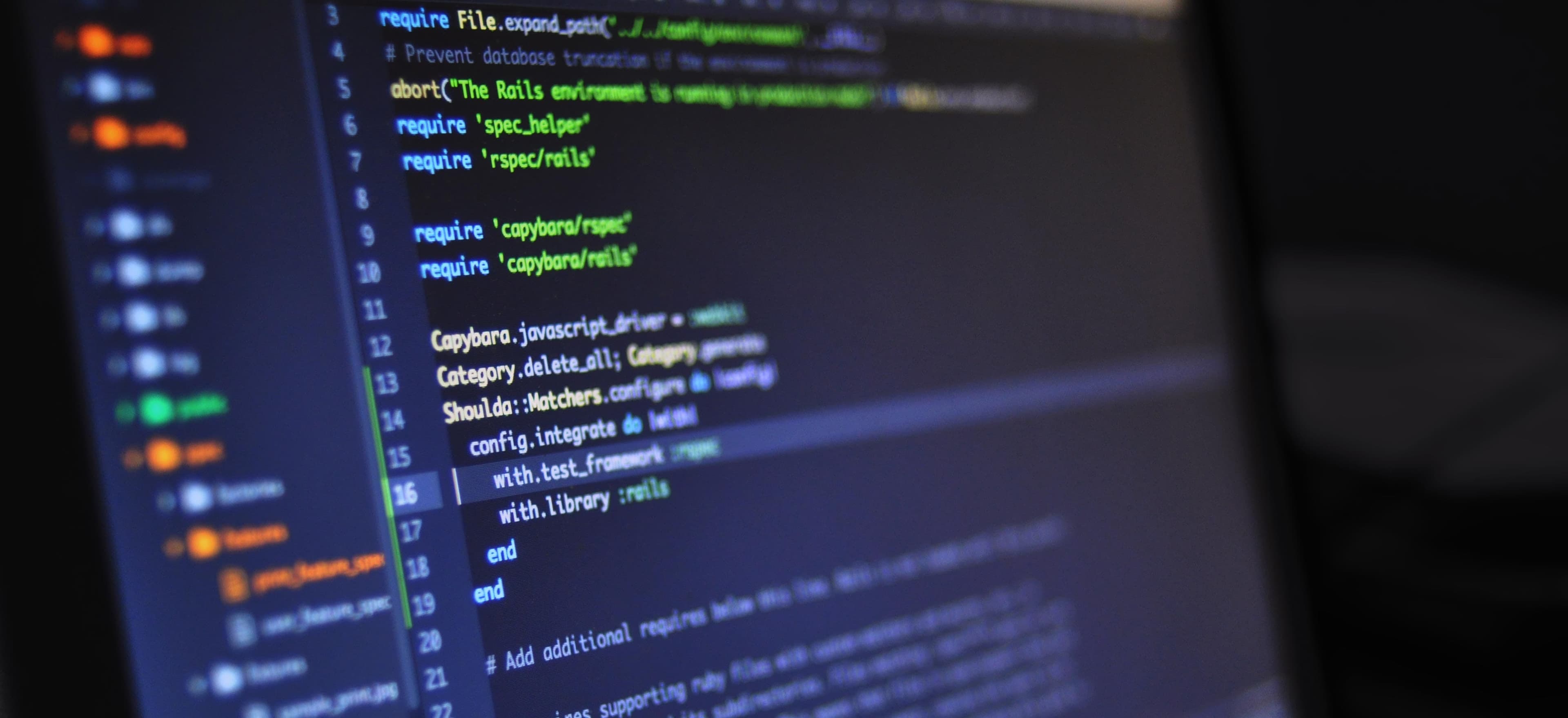
- Published on
Handling Exception in Apache Camel with Groovy
Exception handling is a critical aspect of any software development process. In the context of Apache Camel, which is a powerful open-source integration framework based on known Enterprise Integration Patterns, handling exceptions effectively becomes even more important. In this blog post, we will explore how to handle exceptions in Apache Camel routes using Groovy, a dynamic language that runs on the Java Virtual Machine (JVM). We will discuss various techniques and best practices for handling exceptions in Apache Camel routes using Groovy.
Understanding Exception Handling in Apache Camel
Exception handling in Apache Camel is essential to ensure the reliability and robustness of integration routes. When developing integration solutions with Apache Camel, it's crucial to anticipate and handle exceptions that may occur during message processing. Apache Camel provides a comprehensive set of features and mechanisms for handling exceptions, such as the onException
DSL, which allows route-specific exception handling, and the try-catch-finally
block, which provides more granular exception handling within a route.
Using onException DSL for Route-Specific Exception Handling
One of the primary ways to handle exceptions in Apache Camel routes is by using the onException
DSL. This mechanism allows you to define specific exception handling behavior for individual routes. Let's consider an example where we have a route that reads data from an input source, processes the data, and then writes it to an output destination. We want to handle any exceptions that may occur during the data processing step.
import org.apache.camel.builder.RouteBuilder
class ExceptionHandlingRoute extends RouteBuilder {
void configure() {
onException(Exception.class)
.handled(true)
.maximumRedeliveries(3)
.to("log:errorLog")
from("direct:dataInput")
.process {
// Data processing logic here
// Potential exception may occur
}
.to("direct:output")
from("direct:output")
.to("mock:result")
}
}
In the above example, we create an Apache Camel route using Groovy that defines exception handling for a specific type of exception (Exception.class
). We use the handled(true)
method to indicate that the exception has been handled and prevent it from being propagated further up the route. Additionally, we set maximumRedeliveries(3)
to specify the maximum number of redelivery attempts in case of an exception. Finally, we redirect the exception to an error log for further analysis.
Implementing Granular Exception Handling with try-catch-finally Block
While the onException
DSL allows for route-specific exception handling, there are cases where you may need more granular control over exception handling within a route. This is where the try-catch-finally
block comes into play. You can use the try-catch-finally
block within a route to catch and handle exceptions at a more detailed level.
from("direct:dataInput")
.process {
try {
// Data processing logic here
// Potential exception may occur
} catch(Exception e) {
// Exception handling logic here
} finally {
// Clean-up logic here
}
}
.to("direct:output")
In the above example, we use the try-catch-finally
block within an Apache Camel route to encapsulate the data processing logic. Any exceptions that occur during the data processing step are caught and handled within the catch
block. The finally
block ensures that any necessary clean-up logic is executed, regardless of whether an exception occurred.
Best Practices for Exception Handling in Apache Camel with Groovy
When handling exceptions in Apache Camel routes using Groovy, it's essential to follow best practices to ensure robust and reliable integration solutions. Here are some best practices to consider:
-
Use Specific Exception Types: Whenever possible, handle specific types of exceptions rather than catching general
Exception
types. This allows for more precise and targeted exception handling. -
Logging and Monitoring: Always log exceptions and any relevant information for monitoring and troubleshooting purposes. Apache Camel provides extensive logging capabilities that can be leveraged for this purpose.
-
Retry Mechanisms: Implement retry mechanisms for recoverable exceptions to provide resiliency in case of transient issues. This can be achieved using the
maximumRedeliveries
andredeliveryDelay
options. -
Centralized Error Handling: Consider implementing a centralized error handling mechanism to handle exceptions that are not explicitly handled within individual routes.
-
Unit Testing Exception Handling: Write unit tests to validate the exception handling behavior of your Apache Camel routes. This helps ensure that the exception handling logic behaves as expected.
Final Considerations
Handling exceptions effectively in Apache Camel routes using Groovy is crucial for building robust and reliable integration solutions. By leveraging the onException
DSL for route-specific exception handling and utilizing the try-catch-finally
block for granular exception handling within routes, developers can ensure that their integration solutions can gracefully handle exceptions and recover from errors. By following best practices such as using specific exception types, logging and monitoring, implementing retry mechanisms, and unit testing exception handling, developers can build resilient and fault-tolerant integration solutions with Apache Camel and Groovy.
Exception handling is a critical aspect of any software development process. In the context of Apache Camel, which is a powerful open-source integration framework based on known Enterprise Integration Patterns, handling exceptions effectively becomes even more important. In this blog post, we will explore how to handle exceptions in Apache Camel routes using Groovy, a dynamic language that runs on the Java Virtual Machine (JVM). We will discuss various techniques and best practices for handling exceptions in Apache Camel routes using Groovy.
Understanding Exception Handling in Apache Camel
Exception handling in Apache Camel is essential to ensure the reliability and robustness of integration routes. When developing integration solutions with Apache Camel, it's crucial to anticipate and handle exceptions that may occur during message processing. Apache Camel provides a comprehensive set of features and mechanisms for handling exceptions, such as the onException
DSL, which allows route-specific exception handling, and the try-catch-finally
block, which provides more granular exception handling within a route.
Using onException DSL for Route-Specific Exception Handling
One of the primary ways to handle exceptions in Apache Camel routes is by using the onException
DSL. This mechanism allows you to define specific exception handling behavior for individual routes. Let's consider an example where we have a route that reads data from an input source, processes the data, and then writes it to an output destination. We want to handle any exceptions that may occur during the data processing step.
import org.apache.camel.builder.RouteBuilder
class ExceptionHandlingRoute extends RouteBuilder {
void configure() {
onException(Exception.class)
.handled(true)
.maximumRedeliveries(3)
.to("log:errorLog")
from("direct:dataInput")
.process {
// Data processing logic here
// Potential exception may occur
}
.to("direct:output")
from("direct:output")
.to("mock:result")
}
}
In the above example, we create an Apache Camel route using Groovy that defines exception handling for a specific type of exception (Exception.class
). We use the handled(true)
method to indicate that the exception has been handled and prevent it from being propagated further up the route. Additionally, we set maximumRedeliveries(3)
to specify the maximum number of redelivery attempts in case of an exception. Finally, we redirect the exception to an error log for further analysis.
Implementing Granular Exception Handling with try-catch-finally Block
While the onException
DSL allows for route-specific exception handling, there are cases where you may need more granular control over exception handling within a route. This is where the try-catch-finally
block comes into play. You can use the try-catch-finally
block within a route to catch and handle exceptions at a more detailed level.
from("direct:dataInput")
.process {
try {
// Data processing logic here
// Potential exception may occur
} catch(Exception e) {
// Exception handling logic here
} finally {
// Clean-up logic here
}
}
.to("direct:output")
In the above example, we use the try-catch-finally
block within an Apache Camel route to encapsulate the data processing logic. Any exceptions that occur during the data processing step are caught and handled within the catch
block. The finally
block ensures that any necessary clean-up logic is executed, regardless of whether an exception occurred.
Best Practices for Exception Handling in Apache Camel with Groovy
When handling exceptions in Apache Camel routes using Groovy, it's essential to follow best practices to ensure robust and reliable integration solutions. Here are some best practices to consider:
-
Use Specific Exception Types: Whenever possible, handle specific types of exceptions rather than catching general
Exception
types. This allows for more precise and targeted exception handling. -
Logging and Monitoring: Always log exceptions and any relevant information for monitoring and troubleshooting purposes. Apache Camel provides extensive logging capabilities that can be leveraged for this purpose.
-
Retry Mechanisms: Implement retry mechanisms for recoverable exceptions to provide resiliency in case of transient issues. This can be achieved using the
maximumRedeliveries
andredeliveryDelay
options. -
Centralized Error Handling: Consider implementing a centralized error handling mechanism to handle exceptions that are not explicitly handled within individual routes.
-
Unit Testing Exception Handling: Write unit tests to validate the exception handling behavior of your Apache Camel routes. This helps ensure that the exception handling logic behaves as expected.
Final Considerations
Handling exceptions effectively in Apache Camel routes using Groovy is crucial for building robust and reliable integration solutions. By leveraging the onException
DSL for route-specific exception handling and utilizing the try-catch-finally
block for granular exception handling within routes, developers can ensure that their integration solutions can gracefully handle exceptions and recover from errors. By following best practices such as using specific exception types, logging and monitoring, implementing retry mechanisms, and unit testing exception handling, developers can build resilient and fault-tolerant integration solutions with Apache Camel and Groovy.