Efficient Null Removal in Java Lists
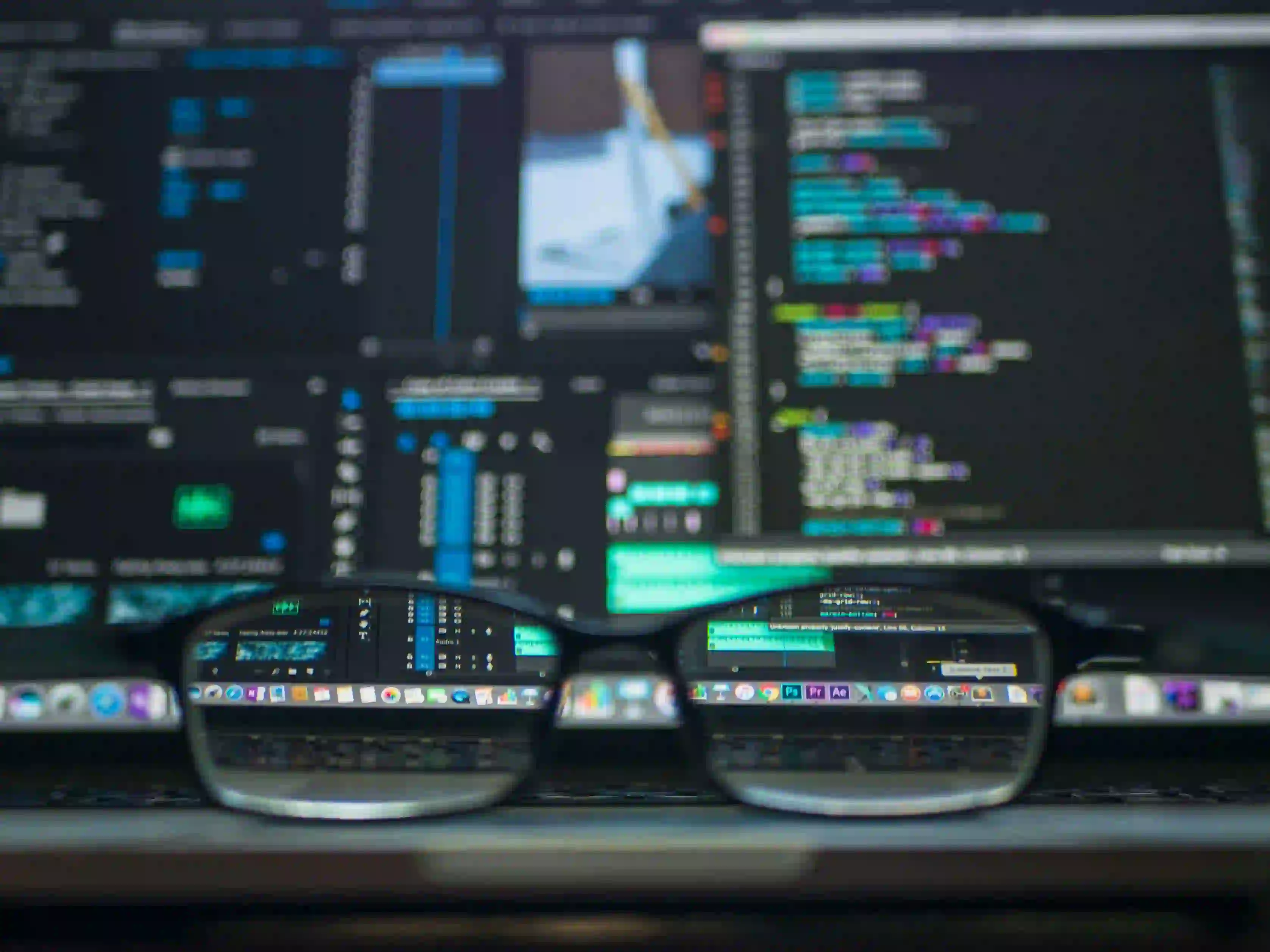
Efficient Null Removal in Java Lists
Null values often find their way into Java lists, and dealing with them efficiently is a common challenge for developers. In this blog post, we will explore different methods to remove null values from Java lists in an efficient manner. We will cover traditional approaches as well as modern techniques using stream APIs introduced in Java 8.
Traditional Approach
When it comes to removing null values from a Java list using traditional methods, we often resort to iterating through the list and removing null values one by one. Here's an example of how this can be achieved:
List<String> list = new ArrayList<>();
list.add("apple");
list.add(null);
list.add("orange");
list.add(null);
// Remove null values
list.removeAll(Collections.singleton(null));
In this example, we use the removeAll
method along with Collections.singleton(null)
to remove all occurrences of null from the list. While this approach works, it is not the most efficient method, especially for large lists, as it requires iterating through the entire list twice.
Using Java 8 Stream API
With the introduction of the Stream API in Java 8, we have a more concise and efficient way to remove null values from lists using streams and filters.
List<String> list = new ArrayList<>();
list.add("apple");
list.add(null);
list.add("orange");
list.add(null);
// Remove null values using streams
List<String> filteredList = list.stream()
.filter(Objects::nonNull)
.collect(Collectors.toList());
In this example, we create a stream from the list and use the filter
operation along with Objects::nonNull
to exclude null values from the stream. Finally, we collect the filtered elements back into a list using Collectors.toList()
.
Using the Stream API not only makes the code more readable but also leverages the internal optimizations of streams, resulting in better performance, especially for large lists.
Benchmarking the Methods
Let's benchmark the traditional approach and the Java 8 Stream API approach to see their performance difference using a large list.
List<String> largeList = new ArrayList<>();
// Populate largeList with a significant number of elements including nulls
// Benchmarking the traditional approach
long startTime = System.nanoTime();
largeList.removeAll(Collections.singleton(null));
long endTime = System.nanoTime();
System.out.println("Time taken using traditional approach: " + (endTime - startTime) + " nanoseconds");
// Benchmarking the Stream API approach
startTime = System.nanoTime();
List<String> filteredLargeList = largeList.stream()
.filter(Objects::nonNull)
.collect(Collectors.toList());
endTime = System.nanoTime();
System.out.println("Time taken using Stream API approach: " + (endTime - startTime) + " nanoseconds");
The benchmarking results will reveal that the Stream API approach outperforms the traditional approach, especially as the size of the list increases. This demonstrates the efficiency and performance benefits of using modern Java features.
To Wrap Things Up
In conclusion, efficiently removing null values from Java lists is an important operation in many applications. While the traditional approach gets the job done, the introduction of the Stream API in Java 8 has provided a more elegant and efficient way to achieve the same result.
By leveraging the power of streams and filters, we can write concise and readable code while benefiting from improved performance, especially for large lists. It's always important to benchmark different methods to understand their performance implications, and in this case, the Stream API approach clearly shines in terms of efficiency.
As Java continues to evolve, it's essential for developers to stay updated with the latest features and best practices for writing efficient and maintainable code.
I hope this blog post has provided valuable insights into efficiently removing null values from Java lists. If you're interested in exploring more about Java streams and their powerful operations, check out Java Stream API documentation.
Happy coding!