Handling Complex State Management with AngularJS
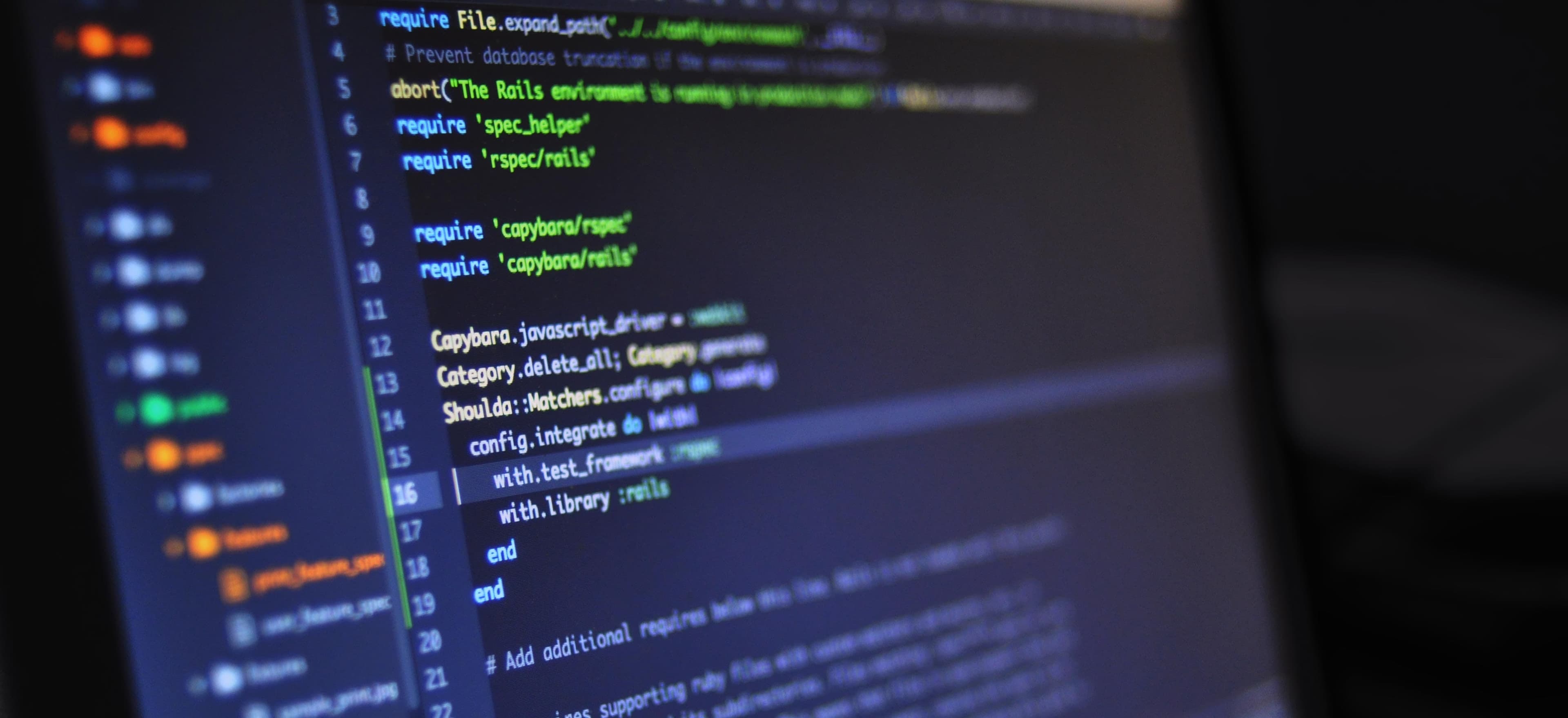
- Published on
Handling Complex State Management with AngularJS
AngularJS is a powerful JavaScript framework that provides a wide range of tools for building web applications. One of the key challenges in front-end development is managing the state of the application. As applications grow in complexity, managing state becomes increasingly challenging. In this article, we will explore how AngularJS can help handle complex state management and provide some best practices to follow.
Understanding State Management
Before diving into how to manage state in AngularJS, it's important to understand what state management entails. State refers to the data that represents the condition of the application at a specific point in time. This can include user input, server responses, and other dynamic information. Effective state management involves keeping track of this data and updating it appropriately as the application evolves.
AngularJS Services for State Management
AngularJS provides several services that are particularly useful for managing state within an application. Two key services that play a significant role in state management are $rootScope
and $stateParams
.
$rootScope
The $rootScope
is the highest level scope in an AngularJS application and serves as the parent scope for all other scopes. It is often used to store and manage global application state. While it can be tempting to use $rootScope
for all state management, it is best practice to use it sparingly, as overusing it can lead to a cluttered and hard-to-maintain codebase.
$stateParams
The $stateParams
service is part of the UI-Router module, which is commonly used for managing application states in AngularJS. It extracts parameters from the URL and makes them available to the application. This is particularly useful for managing the state of different views within the application.
app.controller('ProductDetailController', function($scope, $stateParams) {
$scope.productId = $stateParams.id;
});
In this example, the $stateParams
service is used to retrieve the product ID from the URL and assign it to the $scope
for use within the controller.
Using AngularJS Components for Stateful UI
AngularJS components provide a modular way to encapsulate and manage the state of UI elements within an application. By creating custom components, complex UI elements can be broken down into smaller, manageable pieces, each with their own state. This promotes reusability and maintainability.
Example of a Stateful Component
app.component('taskList', {
templateUrl: 'task-list.html',
controller: function TaskListController() {
this.tasks = [
{ name: 'Task 1', completed: false },
{ name: 'Task 2', completed: true },
{ name: 'Task 3', completed: false }
];
this.markAsCompleted = function(task) {
task.completed = true;
};
}
});
In this example, the taskList
component encapsulates a list of tasks and provides a method to mark a task as completed. Each instance of the taskList
component maintains its own state, isolating it from other parts of the application.
Best Practices for State Management
To effectively manage state in an AngularJS application, consider the following best practices:
Single Source of Truth
Adopt the principle of having a single source of truth for the state of your application. This means centralizing the state into a well-defined structure, such as using services or a state management library like Redux. This approach reduces complexity and makes it easier to maintain and reason about the application's state.
Immutability
Prefer immutable data structures when updating state. By avoiding direct mutation of state, you can prevent unexpected side effects and simplify debugging. Libraries like Immutable.js can be used to work with immutable data structures in JavaScript.
Use AngularJS Events Sparingly
While AngularJS events ($broadcast
, $emit
, and $on
) can be used to communicate changes in state between components, they can also lead to coupling and make it hard to track state changes. Consider using services or a state management library for more controlled and predictable state management.
Utilize $q for Asynchronous State Management
When dealing with asynchronous operations that affect state, use the $q
service for handling promises. This ensures that the state is updated consistently and robustly in response to asynchronous events.
Closing Remarks
Managing state in a complex AngularJS application can be challenging, but by leveraging AngularJS services, components, and following best practices, it is possible to maintain a clean and maintainable codebase. As always, understanding the principles of effective state management and applying them judiciously is key to building robust and responsive applications with AngularJS.
By incorporating the discussed best practices and leveraging AngularJS's powerful features, developers can ensure that their applications effectively manage complex state, leading to improved performance and maintainability.
To delve deeper into AngularJS state management, consider exploring the official documentation for comprehensive insights and examples.
Remember, effective state management is crucial for delivering a seamless user experience and building scalable applications. With AngularJS, developers have a powerful set of tools at their disposal to tackle even the most intricate state management challenges.
Checkout our other articles