Creating a Slot Machine Game in Java: Handling User Input
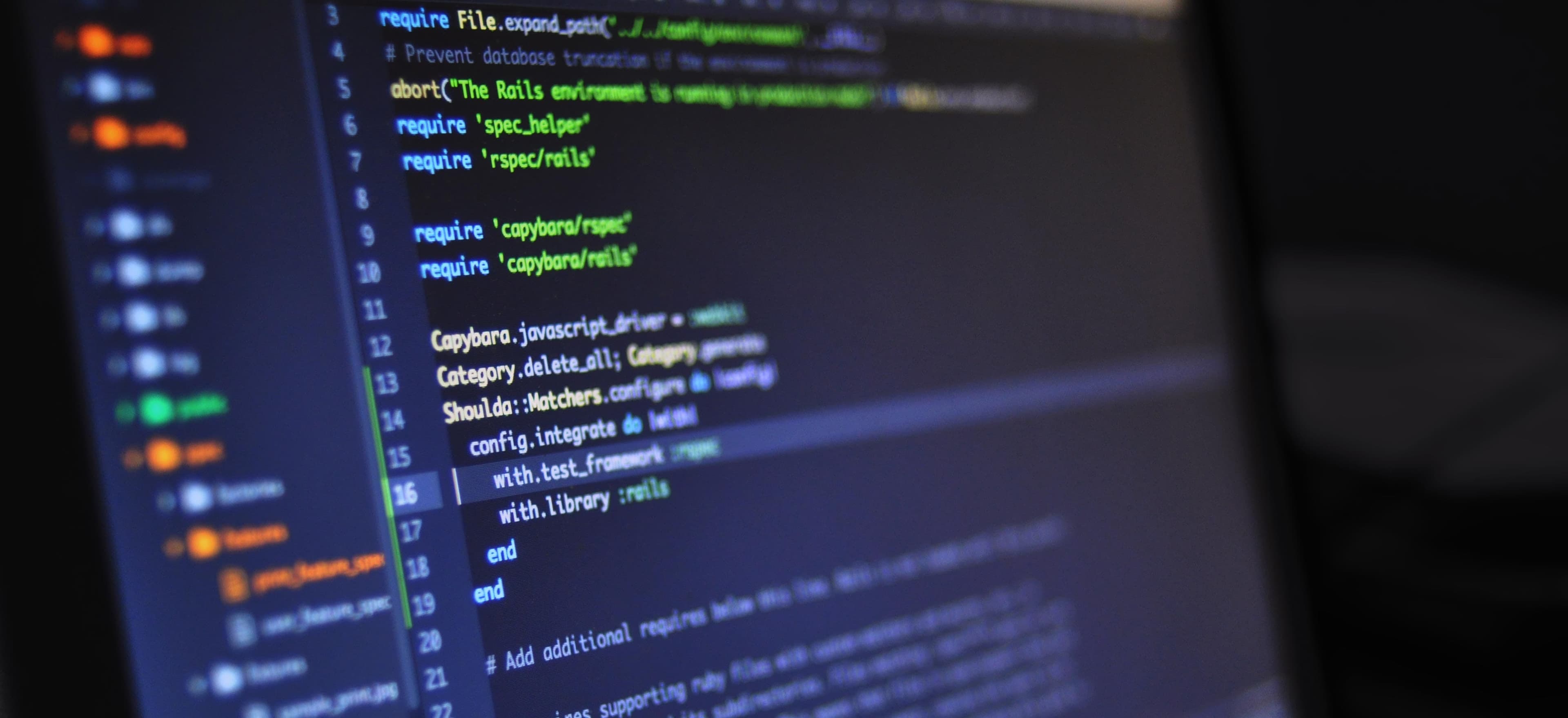
- Published on
In the previous post, we laid the foundation for our slot machine game by creating the main structure and visual components. Now, it's time to handle user input and implement the core functionality of the game.
Handling User Input
1. Capturing User Input
We'll begin by capturing the user's input to spin the slot machine. We can achieve this by adding a button to our game GUI. Let's create a JButton
and add an ActionListener
to capture the user's click.
JButton spinButton = new JButton("Spin");
spinButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Handle spin logic here
}
});
Here, we create a "Spin" button and attach an ActionListener
to it. When the button is clicked, the actionPerformed
method will be invoked, allowing us to handle the spin logic.
2. Handling Spin Logic
Now, let's dive into the spin logic. When the user clicks the "Spin" button, we want the slot machine to display a random combination of symbols. We can achieve this by generating random numbers for each slot and updating the display accordingly.
// Inside actionPerformed method
int[] result = new int[3]; // Represents the symbols on the slot machine
Random random = new Random();
for (int i = 0; i < 3; i++) {
result[i] = random.nextInt(symbols.length); // Assuming symbols is an array of possible symbols
}
// Update the display with the result array
In this snippet, we use the Random
class to generate random numbers, representing the symbols on each slot. We then update the display to show the result of the spin.
3. Validating User Input
In a real slot machine, you wouldn't want users to be able to spin the slots continuously without placing a bet. Similarly, in our game, we should validate whether the user has enough credits to spin the machine.
// Inside actionPerformed method
if (credits >= betAmount) {
// Deduct the bet amount from user's credits
// Perform the spin logic
} else {
// Inform the user that they don't have enough credits
}
Here, we check if the user has enough credits to place a bet. If they do, we deduct the bet amount from their credits and allow the spin. Otherwise, we notify the user that they don't have enough credits to play.
Handling user input is crucial for creating an engaging and interactive game experience. By capturing the user's actions and validating their input, we can ensure that the game runs smoothly and according to the rules.
In the next post, we will explore adding animations to the slot machine to enhance the visual appeal of the game.
Stay tuned for the next update!
We've covered the crucial aspect of handling user input in the slot machine game, ensuring a seamless and enjoyable user experience. Stay tuned for the next post, where we will further enhance the game's visual appeal by adding animations. If you're interested in learning more about Java game development, check out this comprehensive guide.