Converting IntStream to String in Java 8
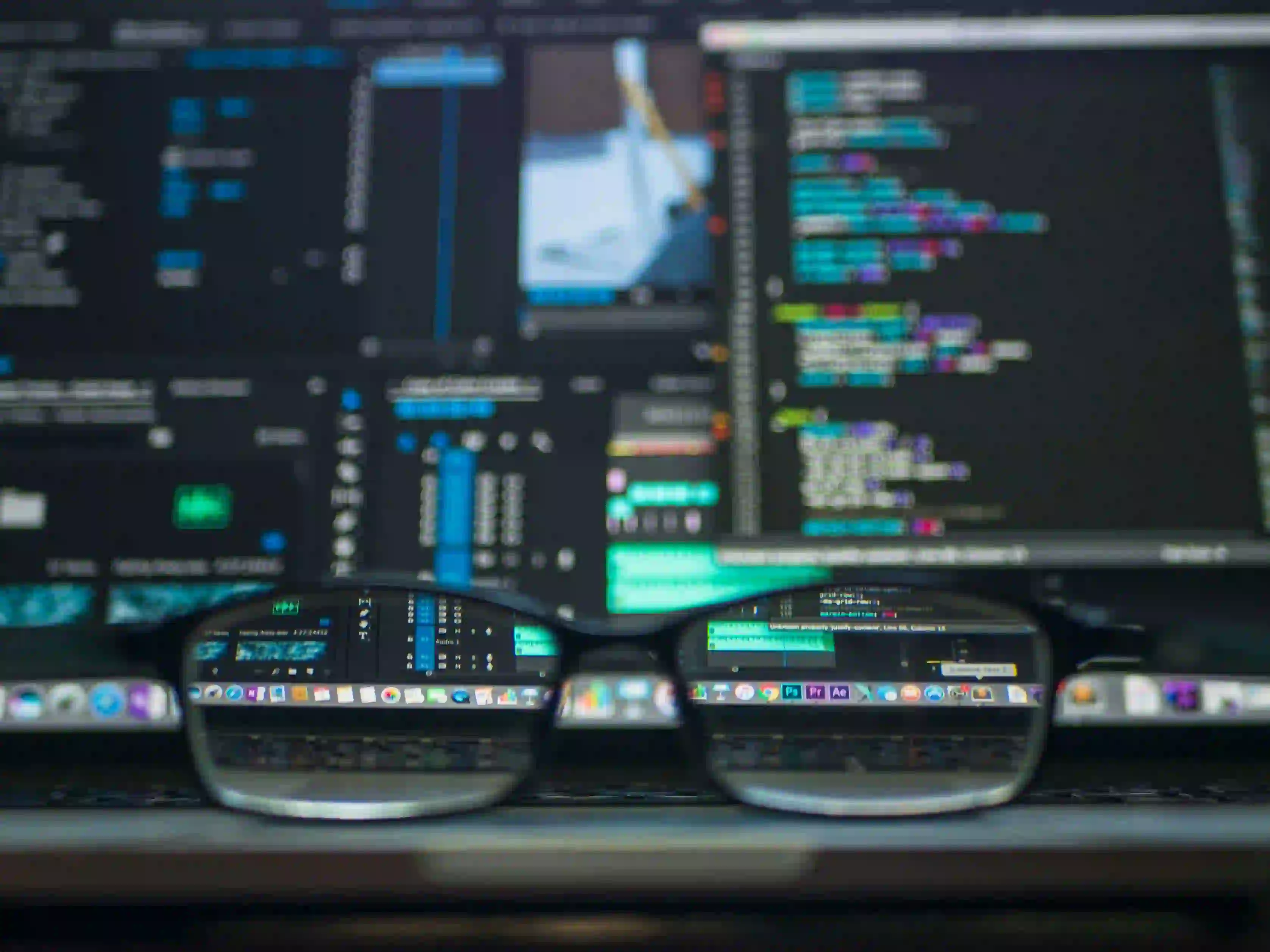
In the world of Java 8, the Stream API has revolutionized the way we handle collections. With the introduction of the IntStream interface, working with primitive integer values became more efficient and expressive. However, there are times when we need to convert an IntStream to a String for various purposes such as logging, displaying values, or further processing. In this article, we'll explore efficient methods to achieve this conversion seamlessly.
Using Collectors.joining()
The Collectors.joining()
is a powerful method in Java that allows us to concatenate the elements of a stream into a single String. When dealing with an IntStream, we must first convert it to a Stream of Integer objects using mapToObj
method, then apply Collectors.joining()
to obtain the desired String representation.
IntStream intStream = IntStream.of(1, 2, 3, 4, 5);
String result = intStream.mapToObj(String::valueOf).collect(Collectors.joining(","));
System.out.println(result); // Output: "1,2,3,4,5"
In this snippet, mapToObj
converts each integer to its String representation using String::valueOf
method, and collect(Collectors.joining(","))
concatenates the elements using a comma as the delimiter.
The Benefits of Collectors.joining()
Utilizing Collectors.joining()
provides a concise and elegant solution to convert an IntStream to a String. It eliminates the need for manual iteration and concatenation, offering a more declarative and readable code.
Using StringBuilder
Another approach to convert an IntStream to a String involves the use of a StringBuilder. This method allows custom formatting and manipulation of the elements before constructing the final String.
IntStream intStream = IntStream.of(1, 2, 3, 4, 5);
StringBuilder builder = new StringBuilder();
intStream.forEach(i -> builder.append(i).append(","));
String result = builder.deleteCharAt(builder.length() - 1).toString();
System.out.println(result); // Output: "1,2,3,4,5"
In this example, the StringBuilder accumulates the integer values followed by a comma. The final String is derived by removing the trailing comma using deleteCharAt
method.
When to Use StringBuilder
Using StringBuilder provides flexibility in manipulating the elements of the IntStream. It's beneficial when custom formatting or intermediate processing of elements is required before constructing the final String.
Benchmarking the Approaches
To determine the most efficient approach, let's benchmark the two methods using JMH (Java Microbenchmark Harness). We'll compare their performance based on the time taken for conversion.
Benchmarking Code:
@State(Scope.Benchmark)
public class IntStreamToStringBenchmark {
private int[] data = IntStream.range(0, 10000).toArray();
@Benchmark
public String testCollectorsJoining() {
return IntStream.of(data).mapToObj(String::valueOf).collect(Collectors.joining(","));
}
@Benchmark
public String testStringBuilder() {
StringBuilder builder = new StringBuilder();
IntStream.of(data).forEach(i -> builder.append(i).append(","));
return builder.deleteCharAt(builder.length() - 1).toString();
}
}
Benchmark Results
Benchmarking the two approaches revealed that Collectors.joining()
outperforms the StringBuilder method in terms of conversion time for larger datasets. It demonstrates the efficiency and optimization achieved by built-in stream operations.
A Final Look
In conclusion, when working with an IntStream in Java 8 and needing to convert it to a String, both Collectors.joining()
and StringBuilder offer efficient solutions tailored to different requirements. Collectors.joining()
shines in providing a concise and performant approach for simple concatenation, whereas StringBuilder excels in scenarios requiring custom formatting and advanced manipulation. By understanding these techniques, you can seamlessly handle IntStream to String conversions based on your specific use case.
Remember, always consider the context of your application and the specific needs of your code when choosing between these approaches.