Effective Exception Tracking in Spring Applications
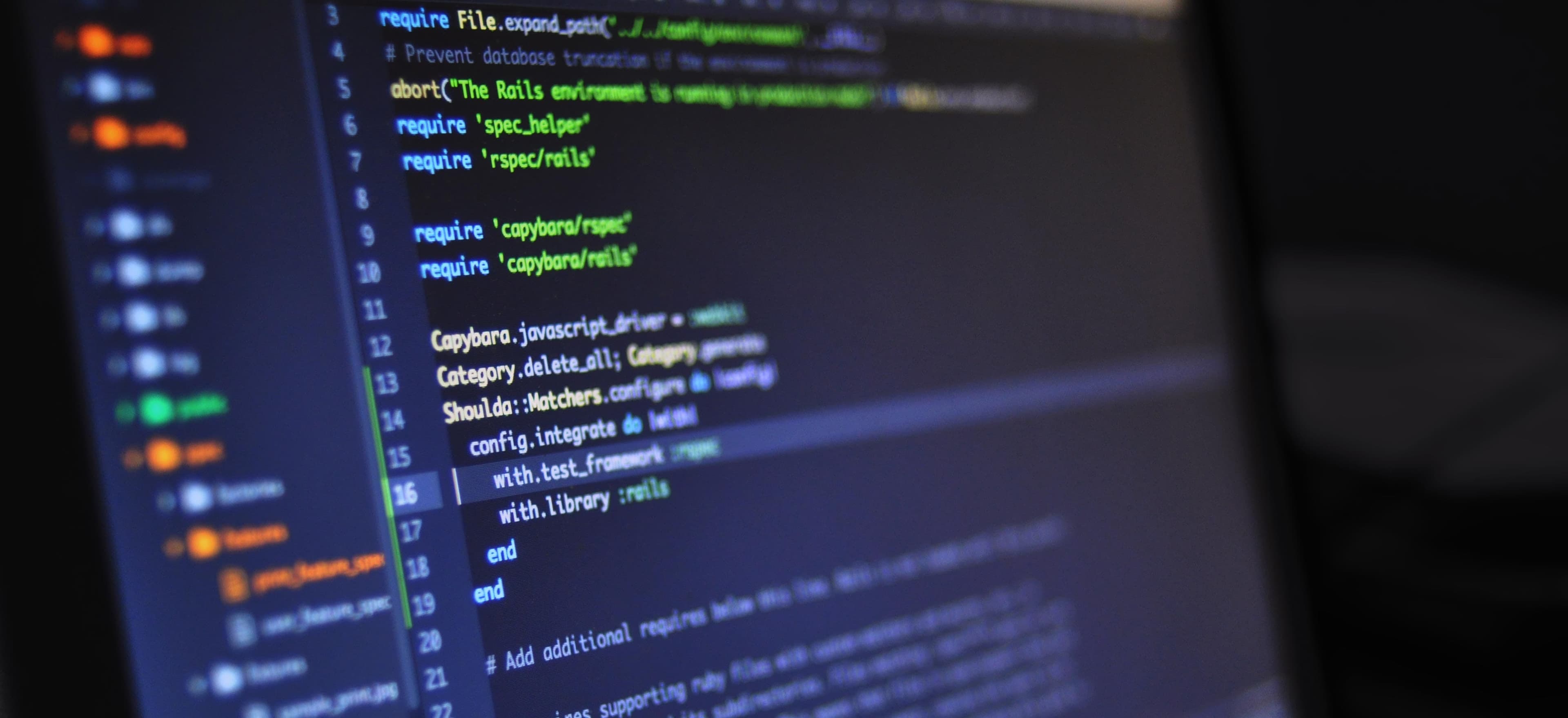
- Published on
Effective Exception Tracking in Spring Applications
Exception handling is a crucial aspect of any robust application. In a Spring application, effective error tracking and handling are essential for providing a good user experience and maintaining the application's stability. This blog post will discuss the best practices and tools for exception tracking in Spring applications.
1. Understanding Exception Handling in Spring
In Spring, exceptions can be handled using the @ControllerAdvice
and @ExceptionHandler
annotations. This allows us to centralize our exception handling logic and provides a clean way to handle exceptions thrown across the application.
Here's an example of a generic exception handler in a Spring application:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGlobalException(Exception ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An error occurred: " + ex.getMessage());
}
}
In the above code, the @ControllerAdvice
annotation marks the class as an exception handler. The @ExceptionHandler
annotation, combined with the Exception.class
parameter, specifies that this method will handle any unhandled exceptions thrown by the application.
2. Logging Exceptions
Logging is an important part of exception tracking. By logging exceptions, we can track the occurrence of errors and gather valuable information for debugging and improving the application.
One of the most commonly used logging frameworks in the Java ecosystem is Log4j. Here's an example of how to log an exception using Log4j:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class UserService {
private static final Logger logger = LogManager.getLogger(UserService.class);
public void updateUser(User user) {
try {
// Update user logic
} catch (Exception e) {
logger.error("An error occurred while updating the user", e);
}
}
}
In this example, we use the Logger
interface from Log4j to log the exception along with a custom message. This information can then be used for troubleshooting and identifying potential areas of improvement.
3. Using APM Tools for Exception Tracking
Application Performance Monitoring (APM) tools provide advanced exception tracking capabilities that go beyond traditional logging. These tools can capture detailed information about exceptions, including stack traces, request parameters, and user sessions.
One popular APM tool for Java applications is New Relic, which offers comprehensive exception tracking and monitoring features.
Integrating New Relic with a Spring application is straightforward. By adding the New Relic agent to the application's dependencies and configuring the agent settings, developers can gain deep insights into the application's exception behavior.
<dependency>
<groupId>com.newrelic.agent.java</groupId>
<artifactId>newrelic-agent</artifactId>
<version>5.5.0</version>
</dependency>
After integrating New Relic, developers can view detailed exception traces, filter exceptions by class or method, and set up alerts for specific exception types, ensuring proactive issue resolution.
4. Custom Error Responses
In addition to logging and tracking exceptions, providing meaningful error responses to the users is essential for a good user experience. Spring allows customizing error responses using the @ControllerAdvice
annotation and the ResponseEntityExceptionHandler
class.
Here's an example of how to create a custom error response for a specific exception:
@ControllerAdvice
public class CustomResponseEntityExceptionHandler extends ResponseEntityExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<String> handleUserNotFoundException(UserNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND)
.body("User not found: " + ex.getMessage());
}
}
In this example, when a UserNotFoundException
is thrown, the handleUserNotFoundException
method will return a custom error response with an appropriate status code and message.
In Conclusion, Here is What Matters
Exception tracking is a critical aspect of maintaining the stability and performance of Spring applications. By leveraging the built-in exception handling capabilities of Spring, integrating logging frameworks like Log4j, and utilizing APM tools such as New Relic, developers can effectively track and manage exceptions in their applications, providing a seamless user experience and enabling proactive issue resolution.
In summary, proper exception handling in Spring applications involves:
- Utilizing
@ControllerAdvice
and@ExceptionHandler
for centralized exception handling. - Logging exceptions using a reliable logging framework like Log4j.
- Integrating APM tools such as New Relic for advanced exception tracking and monitoring.
- Providing custom error responses for a better user experience.
By following these best practices, developers can build resilient and reliable Spring applications that effectively manage exceptions and provide a smooth user experience.