Common Challenges When Upgrading to Spring 4
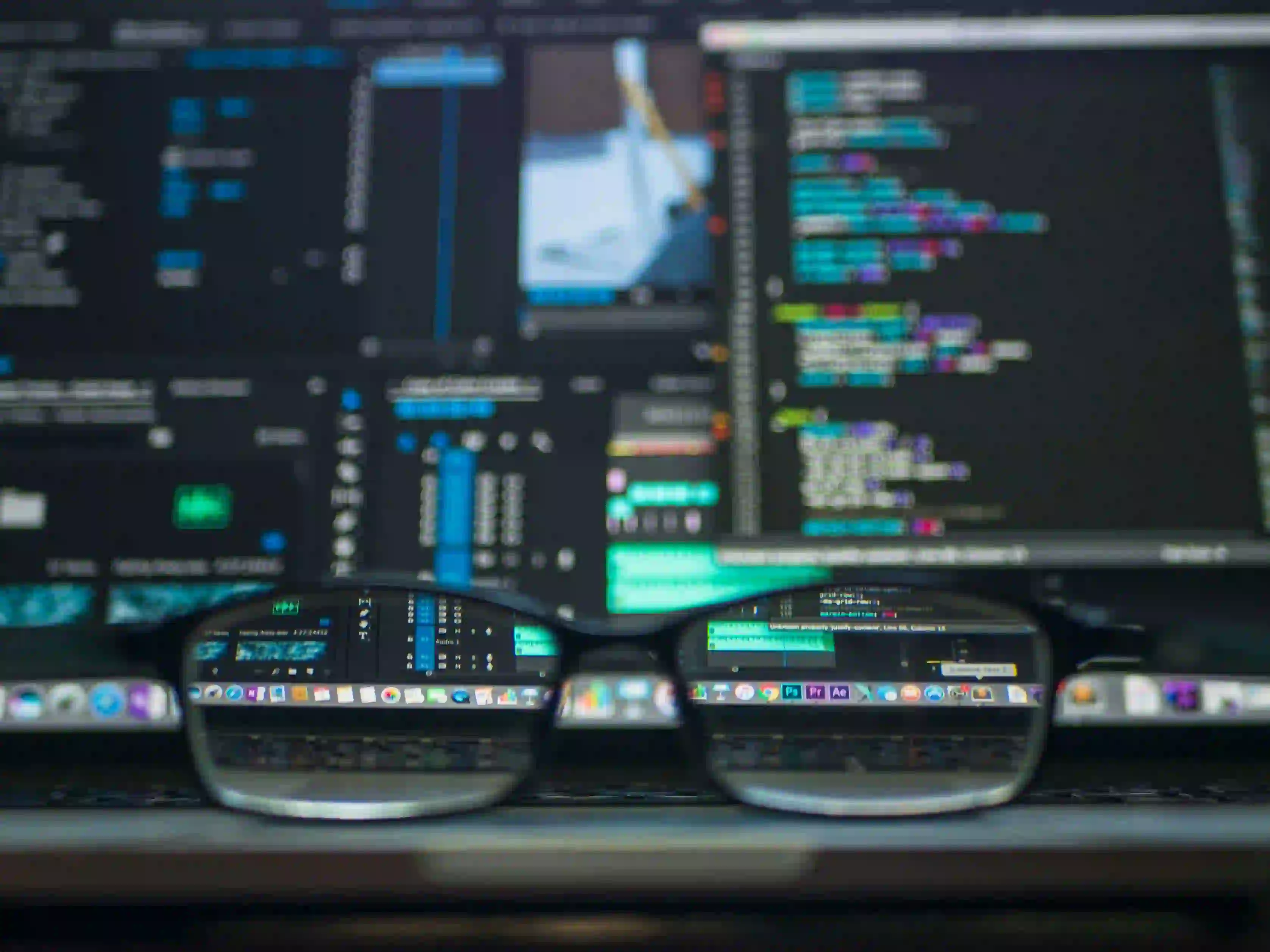
Common Challenges When Upgrading to Spring 4
Spring is a popular Java framework used for building enterprise-level applications. With the release of Spring 4, many developers are considering upgrading their existing applications to leverage the new features and improvements offered by the latest version. However, the upgrade process is not always seamless and can present several challenges. In this article, we will discuss some common challenges that developers may encounter when upgrading to Spring 4 and provide solutions to overcome them.
1. Deprecated Features
One of the primary challenges when upgrading to Spring 4 is dealing with deprecated features from previous versions. Many classes and methods have been marked as deprecated in Spring 4, requiring developers to refactor their code to replace these deprecated elements with their updated counterparts. This can be a time-consuming task, especially for large codebases.
Solution:
Using the Spring Migration Guide can be immensely helpful in identifying the deprecated features and understanding the recommended alternatives. It is crucial to systematically address each deprecated element and make the necessary changes to ensure compatibility with Spring 4.
2. Configuration Changes
Spring 4 introduces several configuration changes, including updates to XML namespaces and changes in annotation usage. These modifications can impact the way applications are configured and initialized, potentially leading to runtime errors if not handled properly during the upgrade process.
Solution:
When faced with configuration changes, it is imperative to review the official Spring 4 documentation to understand the updated configuration patterns and best practices. Additionally, comprehensive testing of the application after making configuration adjustments is essential to catch any potential issues before deployment.
3. Java Version Compatibility
Spring 4 has a minimum Java version requirement of Java 6, rendering it incompatible with older Java versions that might have been used in existing applications. Upgrading to Spring 4 may necessitate upgrading the Java version as well, which can be a complex process, especially for legacy systems.
Solution:
Prior to upgrading to Spring 4, it is crucial to ensure that the application's runtime environment is compatible with the minimum required Java version. This may involve updating the Java Development Kit (JDK) and modifying the build configurations to target the appropriate Java version.
4. Dependency Management
Upgrading to Spring 4 often involves updating dependencies, such as Hibernate, JPA, or other libraries that integrate with the Spring framework. Managing these dependencies and ensuring compatibility with the new Spring version can be onerous, especially when dealing with a multitude of third-party libraries.
Solution:
Utilizing build automation tools like Maven or Gradle can streamline the process of updating dependencies to their compatible versions for Spring 4. Dependency management tools help in resolving transitive dependencies and maintaining consistency across the project's dependencies, simplifying the upgrade process.
Code Example: Handling Deprecated org.springframework.beans.factory.BeanFactory
Interface
// Deprecated usage
import org.springframework.beans.factory.BeanFactory;
public class MyService {
private BeanFactory beanFactory;
// Deprecated method
public void setBeanFactory(BeanFactory beanFactory) {
this.beanFactory = beanFactory;
}
}
In Spring 4, the org.springframework.beans.factory.BeanFactory
interface has been deprecated in favor of org.springframework.beans.factory.BeanFactoryAware
. Below is the updated code:
// Updated usage
import org.springframework.beans.factory.BeanFactoryAware;
public class MyService implements BeanFactoryAware {
private BeanFactory beanFactory;
@Override
public void setBeanFactory(BeanFactory beanFactory) {
this.beanFactory = beanFactory;
}
}
In this example, the deprecated BeanFactory
interface is replaced with BeanFactoryAware
, as recommended in the Spring Migration Guide.
5. Behavioral Changes
Spring 4 introduces behavioral changes in certain components, such as the MVC framework and core container functionalities. These changes can impact the runtime behavior of the application, potentially leading to unexpected outcomes if not accounted for during the upgrade.
Solution:
Thoroughly examining the release notes and change logs for Spring 4 can provide valuable insights into the behavioral changes introduced. Understanding and addressing these changes, such as alterations in request mapping behavior or resource handling, is vital to prevent runtime issues post-upgrade.
6. Testing and Validation
After upgrading to Spring 4, comprehensive testing and validation are crucial to ensure the stability and correctness of the application. Existing test suites, especially integration tests, may require adjustments to accommodate the changes introduced by Spring 4.
Solution:
Reviewing and updating test cases to align with the modified behavior of the application due to the Spring 4 upgrade is essential. Additionally, conducting thorough integration testing to validate the interactions between different components and modules is imperative to uncover any regressions or compatibility issues.
Code Example: Testing Controller with MockMVC in Spring 4
// Previous test using standalone setup
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = "classpath:test-config.xml")
public class MyControllerTest {
private MockMvc mockMvc;
@Autowired
private WebApplicationContext webApplicationContext;
@Before
public void setup() {
this.mockMvc = MockMvcBuilders.standaloneSetup(new MyController()).build();
}
@Test
public void testController() throws Exception {
mockMvc.perform(get("/endpoint"))
.andExpect(status().isOk());
}
}
With Spring 4, the recommended approach is to use WebAppConfiguration
and @WebMvcTest
in conjunction with MockMvc
instead of standalone setup. Here's the updated test:
// Updated test using @WebAppConfiguration and @WebMvcTest
@RunWith(SpringJUnit4ClassRunner.class)
@WebAppConfiguration
@WebMvcTest(MyController.class)
public class MyControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testController() throws Exception {
mockMvc.perform(get("/endpoint"))
.andExpect(status().isOk());
}
}
In this example, the test configuration is updated to align with the recommended testing approach for Spring 4.
In conclusion, upgrading to Spring 4 offers numerous benefits in terms of performance, security, and new features. However, it is essential for developers to be aware of the potential challenges and invest time in careful planning and thorough testing to ensure a smooth transition. By addressing the deprecated features, configuration changes, Java version compatibility, dependency management, behavioral changes, and testing considerations, developers can effectively navigate the upgrade process and leverage the capabilities of Spring 4 in their applications.