The Challenge of Managing Data Consistency in Microservices
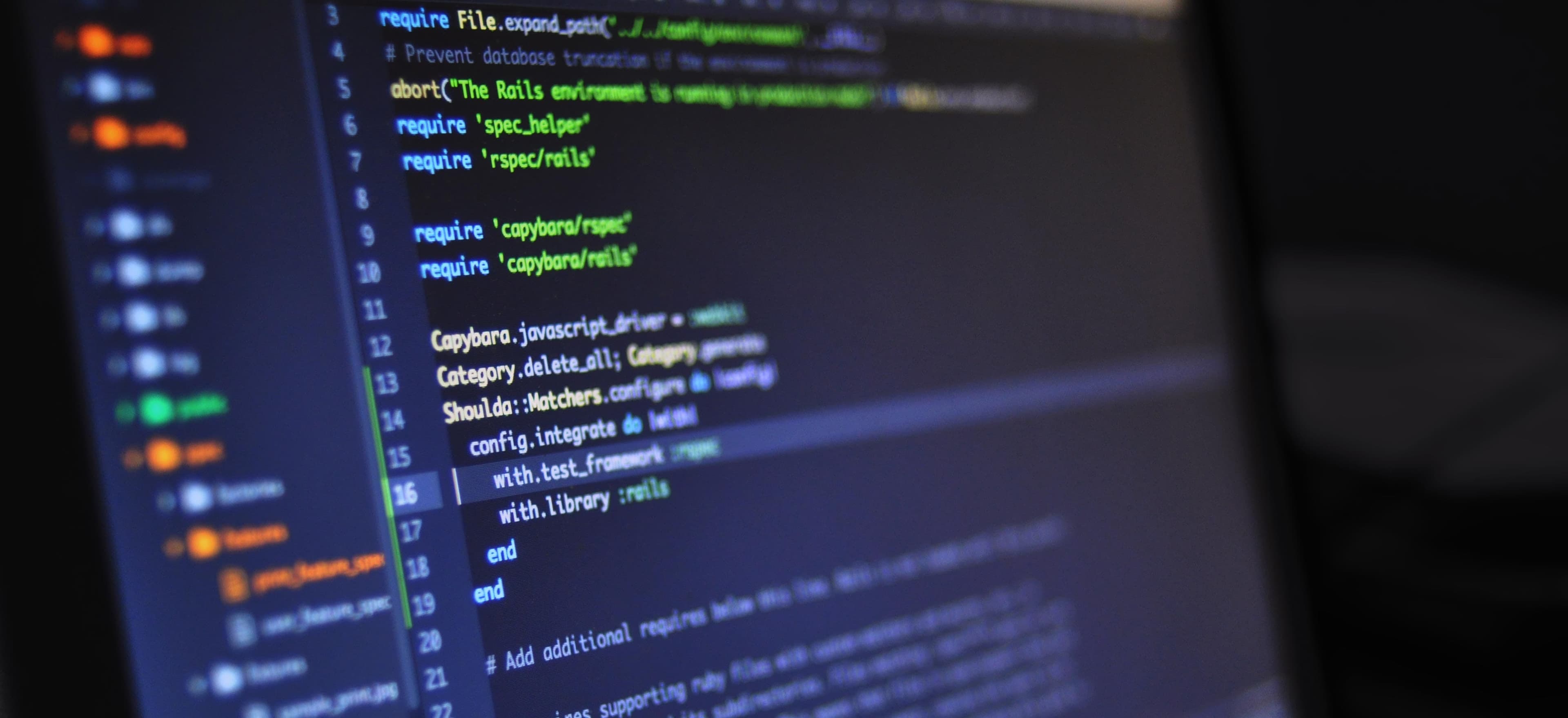
- Published on
The Challenge of Managing Data Consistency in Microservices
Microservices architecture has gained popularity due to its flexibility, scalability, and ease of deployment. However, this approach also brings about a significant challenge when it comes to managing data consistency across multiple services. In this blog post, we'll explore the complexities involved in maintaining data consistency within a microservices environment and discuss some strategies for addressing this challenge.
Understanding the Data Consistency Challenge
In a traditional monolithic application, data is typically stored in a single database, and transactions are managed within the scope of that single database. However, in a microservices architecture, each service may have its own database, and interactions between services can result in distributed transactions that span multiple databases. This distributed nature introduces complexities that can lead to data consistency issues.
Consider a scenario where a customer places an order, and the order service needs to update the inventory in the product catalog service. If the order is successfully placed but the inventory update fails, the system's data will be left in an inconsistent state. Achieving consistency across these distributed transactions becomes a non-trivial task.
Strategies for Managing Data Consistency
1. Synchronous Communication
One approach to managing data consistency is to use synchronous communication between services, where a service makes a request to another service and waits for a response before proceeding. This allows for immediate feedback and can help ensure consistency. However, this approach can introduce problems such as increased latency and decreased resilience, especially as the number of services grows.
2. Asynchronous Event-Based Communication
Another approach is to use asynchronous, event-based communication between services. When an action occurs in one service, it publishes an event that other services can subscribe to and react accordingly. This approach decouples services and can improve scalability and resilience. However, it introduces complexities related to handling out-of-order events and ensuring exactly-once processing.
3. Saga Pattern
The saga pattern is a pattern for managing distributed transactions, where a long-running transaction is divided into a sequence of smaller, local transactions across multiple services. Each step in the saga is designed to be either completed or compensated if a failure occurs. This pattern provides a way to maintain data consistency in a distributed environment but requires careful design and implementation.
Handling Data Consistency in Practice
Let's consider a practical example of managing data consistency in a microservices environment using Java. Suppose we have an e-commerce application with separate services for orders, inventory, and payment processing. When a customer places an order, we need to ensure that the inventory is updated and the payment is processed successfully.
Using the Saga Pattern in Java
@Compensate(OrderCompensate.class)
public class PlaceOrderSaga implements Saga {
@Autowired
private OrderService orderService;
@Autowired
private InventoryService inventoryService;
@Autowired
private PaymentService paymentService;
@Start
public void placeOrder(Order order) {
// Step 1: Place order
orderService.placeOrder(order);
// Step 2: Reserve inventory
try {
inventoryService.reserveInventory(order.getProducts());
} catch (Exception e) {
compensate(order);
throw e;
}
// Step 3: Process payment
try {
paymentService.processPayment(order.getTotalAmount());
} catch (Exception e) {
compensate(order);
throw e;
}
}
}
In this example, we use the Saga pattern to manage the process of placing an order. The PlaceOrderSaga
class represents the saga, and it includes the necessary steps for placing an order, reserving inventory, and processing payment. If any step fails, the compensate
method is called to compensate for the failed transaction.
Wrapping Up
Managing data consistency in a microservices environment is a challenging and complex task. It requires careful consideration of the trade-offs between consistency, latency, resilience, and complexity. By understanding the challenges and implementing appropriate strategies such as synchronous communication, asynchronous event-based communication, and the saga pattern, developers can work towards maintaining data consistency while reaping the benefits of a microservices architecture.
In conclusion, data consistency in microservices requires a thoughtful and deliberate approach, balancing trade-offs and considering the specific requirements of the application. By leveraging the right patterns and technologies, such as the Saga pattern in Java, developers can build resilient and consistent microservices architectures.
For further exploration of microservices and Java, please refer to Java Microservices: What Is It and What Tools to Use for a comprehensive overview.
Remember, managing data consistency is paramount to the success of a microservices architecture. It is crucial to understand the complexities and employ the right strategies to ensure data consistency in a distributed environment.