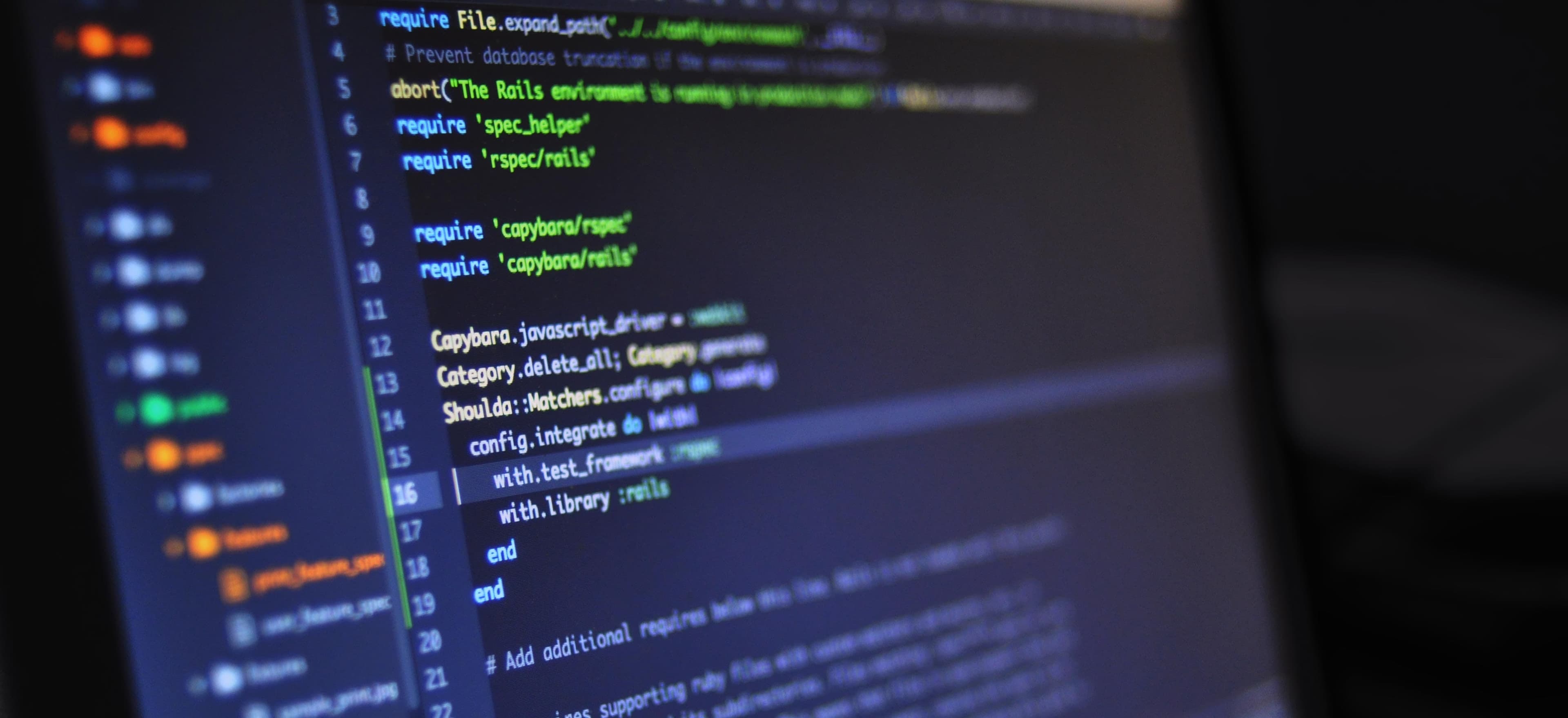
- Published on
Eliminating XML: Simplifying Spring Configuration
When it comes to Java development, the Spring framework has long been a cornerstone, providing a robust infrastructure for building enterprise applications. One of the traditional components of Spring development has been the configuration of beans through XML files. However, with the evolution of the framework, alternative approaches have emerged, offering more streamlined and developer-friendly ways to configure Spring applications.
In this blog post, we will explore the transition from XML-based configuration to the more modern, code-centric approaches offered by the Spring framework. We'll delve into the benefits of moving away from XML, the enhanced readability and maintainability of code-based configuration, and how these changes align with modern development practices.
The Problem with XML Configuration
Traditionally, Spring applications have relied heavily on XML configuration files for defining beans, dependencies, and various aspects of the application's behavior. While this approach provided a standardized way to configure the application, it also introduced several pain points:
-
Boilerplate Code: XML configuration often led to verbose and boilerplate code, making it challenging to maintain and understand the application's configuration.
-
Loosely Typed: XML files lacked the type safety and compile-time checks that are inherent in Java code. This often resulted in runtime errors that could have been caught earlier with a code-centric approach.
-
Externalized Configuration: Storing configuration in external XML files added complexity to the deployment and management of Spring applications.
Enter Java-based Configuration
In response to these challenges, the Spring framework introduced support for Java-based configuration, allowing developers to define beans and their dependencies using standard Java classes and annotations. This approach, often referred to as "JavaConfig," offers several advantages over XML configuration:
-
Type Safety: By leveraging Java's strong typing system, JavaConfig provides compile-time checks, reducing the likelihood of runtime errors related to configuration.
-
Refactoring and Tooling: Java-based configuration allows for easy refactoring using standard IDE features, such as "find usages" and "rename," making configuration changes more manageable.
-
Integrated with Code: With JavaConfig, the configuration is integrated directly into the application's codebase, promoting better code organization and readability.
Let's take a look at a simple example to illustrate the transition from XML to Java-based configuration in a Spring application.
XML Configuration Example
<!-- beans.xml -->
<beans>
<bean id="userService" class="com.example.UserService">
<property name="userDao" ref="userDao"/>
</bean>
<bean id="userDao" class="com.example.UserDao"/>
</beans>
In this XML configuration, we define two beans: userService
and userDao
, along with their dependencies. Now, let's reimplement the same configuration using Java-based configuration.
Java-based Configuration Example
@Configuration
public class AppConfig {
@Bean
public UserService userService() {
return new UserService(userDao());
}
@Bean
public UserDao userDao() {
return new UserDao();
}
}
In this Java-based configuration, we use the @Configuration
annotation to indicate that the class contains bean definitions. The @Bean
annotation is used to denote methods that return bean instances. This approach not only eliminates the verbosity of XML but also provides enhanced readability and type safety.
Leveraging Spring Annotations
In addition to JavaConfig, Spring offers a rich set of annotations that can further simplify the configuration of beans and other components. Let's explore some of the commonly used annotations and their benefits:
@ComponentScan
The @ComponentScan
annotation enables automatic discovery of Spring-managed components, such as beans, within the specified base packages. This eliminates the need to manually register each bean, promoting a more dynamic and flexible application configuration.
@Configuration
@ComponentScan("com.example")
public class AppConfig {
// No explicit bean definitions
}
@Autowired
The @Autowired
annotation is used to inject dependencies into beans without the need for explicit configuration. This annotation is especially useful when working with complex object graphs, as it reduces the need for manual wiring of dependencies.
@Service
public class UserService {
private final UserDao userDao;
@Autowired
public UserService(UserDao userDao) {
this.userDao = userDao;
}
// ...
}
By leveraging annotations such as @ComponentScan
and @Autowired
, developers can streamline the configuration of Spring applications, reducing the amount of explicit configuration code while improving maintainability.
Leveraging Spring Boot for Even Simpler Configuration
While JavaConfig and annotations have simplified Spring configuration, Spring Boot takes this a step further by offering a convention-over-configuration approach. With Spring Boot, many aspects of the application, including configuration, are handled automatically, requiring minimal developer intervention.
Let's explore some of the key features of Spring Boot that contribute to simplified configuration:
Auto-configuration
Spring Boot's auto-configuration feature automatically configures the application based on its classpath and the presence of certain beans. This eliminates the need for extensive manual configuration, allowing developers to focus on writing application logic rather than setting up infrastructure.
Externalized Configuration
Spring Boot provides support for externalized configuration, allowing developers to define properties in various formats, such as YAML or properties files. This approach simplifies the management of configuration settings, especially in microservices architectures where multiple instances of an application may require different configurations.
Opinionated Defaults
Spring Boot embraces opinionated defaults, providing pre-configured settings for various components, such as embedded servers, database connections, and logging. This eliminates the need for developers to make numerous decisions when setting up a new project, while still allowing for customization when necessary.
By embracing Spring Boot, developers can further reduce the complexity of Spring configuration, allowing for rapid application development with minimal effort spent on boilerplate configuration code.
My Closing Thoughts on the Matter
In conclusion, the evolution of the Spring framework has led to a significant shift in the way developers configure their applications. By moving away from XML-based configuration in favor of Java-based configuration, along with the use of powerful annotations and the adoption of Spring Boot, developers can simplify the configuration process, reduce boilerplate code, and align with modern development practices.
As the Spring framework continues to evolve, it's essential for developers to stay informed about the latest best practices and tools for simplifying configuration, ultimately enabling them to focus more on building robust and maintainable applications.
With the rise of modern development practices and the continued evolution of the Spring framework, the trend of simplifying Spring configuration through code-centric approaches is set to continue, providing developers with the tools they need to build powerful, efficient, and maintainable applications.
In your experience, what challenges have you faced with XML-based Spring configuration, and how have you simplified the configuration process using Java-based configuration, annotations, or Spring Boot? Let's continue the conversation in the comments.
Join us in the next blog post as we explore the migration from Struts to Spring MVC and the benefits it brings to modern web application development.