Troubleshooting Apache CXF JAX-WS Implementation
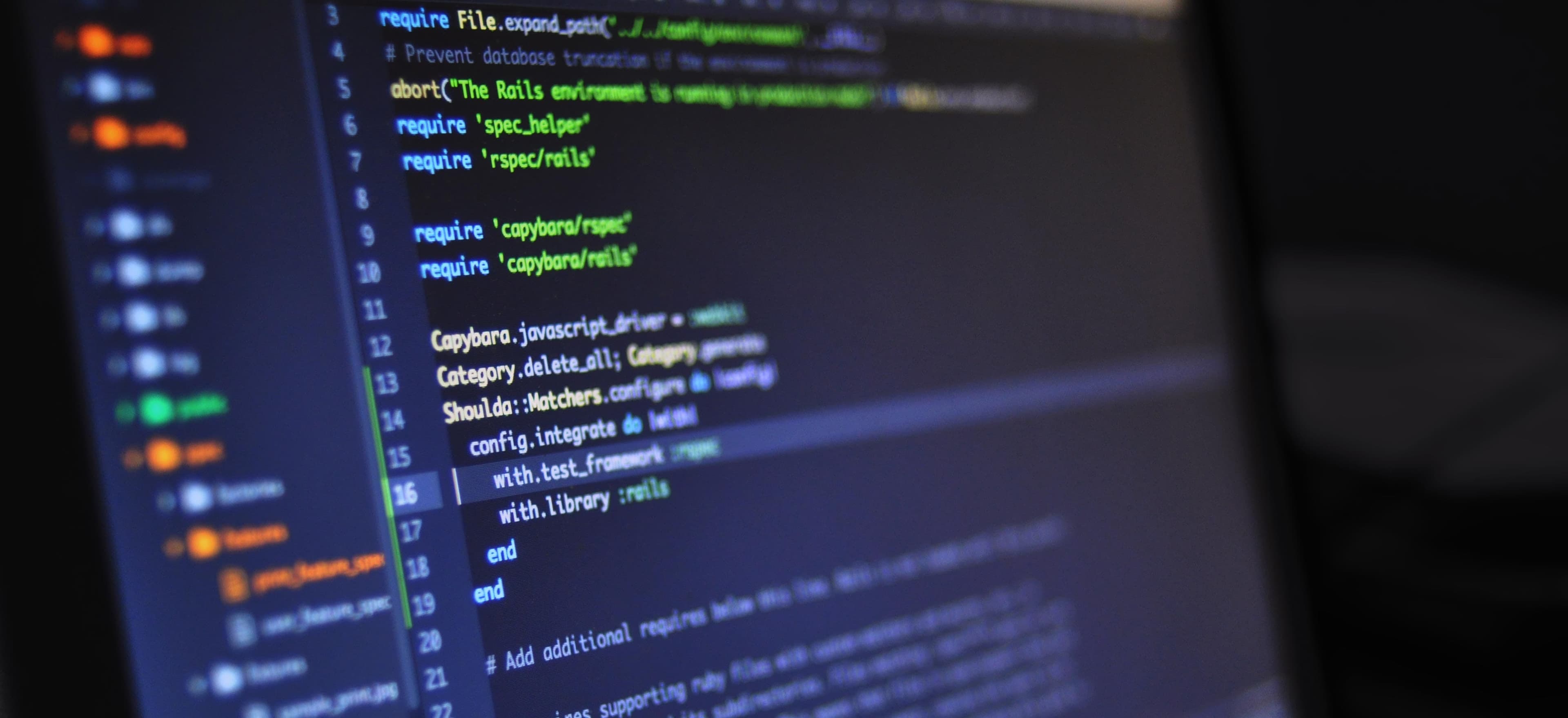
- Published on
Troubleshooting Apache CXF JAX-WS Implementation
When working with web services in Java, Apache CXF is a powerful and flexible framework for building and consuming SOAP and RESTful web services. However, despite its robustness, troubleshooting issues may arise during the development and implementation process.
In this blog post, we will discuss common issues that developers may encounter when working with the Apache CXF JAX-WS implementation and provide practical solutions to address these challenges.
Issue 1: Unable to Generate Client Code from WSDL
One common issue when using Apache CXF is the inability to generate client code from a WSDL file. This can be frustrating, especially when trying to consume an external web service.
Solution:
Make sure you have the necessary dependencies in your project. Include the cxf-codegen-plugin
plugin in your Maven POM file. This plugin is responsible for generating Java code from WSDL. Here's an example configuration for the plugin:
<build>
<plugins>
<plugin>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-codegen-plugin</artifactId>
<version>${cxf.version}</version>
<executions>
<execution>
<id>generate-sources</id>
<phase>generate-sources</phase>
<configuration>
<sourceRoot>${basedir}/target/generated/src/main/java</sourceRoot>
<wsdlOptions>
<wsdlOption>
<wsdl>${basedir}/src/main/resources/wsdl/your_wsdl_file.wsdl</wsdl>
</wsdlOption>
</wsdlOptions>
</configuration>
<goals>
<goal>wsdl2java</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
By configuring the cxf-codegen-plugin
, you can generate Java classes from the WSDL files.
Issue 2: Bean Validation Not Being Applied to JAX-WS Endpoints
Another common issue is when bean validation annotations are not being applied to JAX-WS endpoints, even though they are present in the code.
Solution:
Ensure that you have the cxf-rt-rs-validation
module in your classpath. This module provides support for bean validation in JAX-RS and JAX-WS endpoints.
Include the following Maven dependency in your project to utilize this module:
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-rs-validation</artifactId>
<version>${cxf.version}</version>
</dependency>
After adding the cxf-rt-rs-validation
module to your project, the bean validation annotations should be properly applied to your JAX-WS endpoints.
Issue 3: Unable to Set Custom Message Context Properties
Sometimes, developers have trouble setting custom message context properties in CXF JAX-WS.
Solution:
To set custom message context properties, you can use an Interceptor
to manipulate the message context before and after the message is handled. Here's an example of an Interceptor
that sets custom message context properties:
public class CustomMessageInterceptor extends AbstractPhaseInterceptor<Message> {
public CustomMessageInterceptor() {
super(Phase.PRE_INVOKE);
}
@Override
public void handleMessage(Message message) {
// Set custom message context property
message.put("customProperty", "customValue");
}
}
In this example, we create a custom Interceptor
that operates at the PRE_INVOKE
phase and sets a custom message context property. By adding this Interceptor
to your JAX-WS endpoint, you can manipulate the message context as needed.
Final Thoughts
In this blog post, we discussed common issues encountered when working with the Apache CXF JAX-WS implementation and provided practical solutions to address these challenges. By addressing issues such as generating client code from WSDL, applying bean validation to JAX-WS endpoints, and setting custom message context properties, developers can streamline the development and implementation process of web services using Apache CXF.
Remember, troubleshooting is an integral part of the development process, and mastering the art of resolving these issues will make you a more proficient developer when working with Apache CXF.
For more in-depth information on Apache CXF, you can refer to the official Apache CXF documentation. Additionally, the CXF User's Mailing List is a valuable resource for seeking assistance and engaging in discussions with the CXF community.
Checkout our other articles