Implementing a Flexible Plugin System for Web Apps
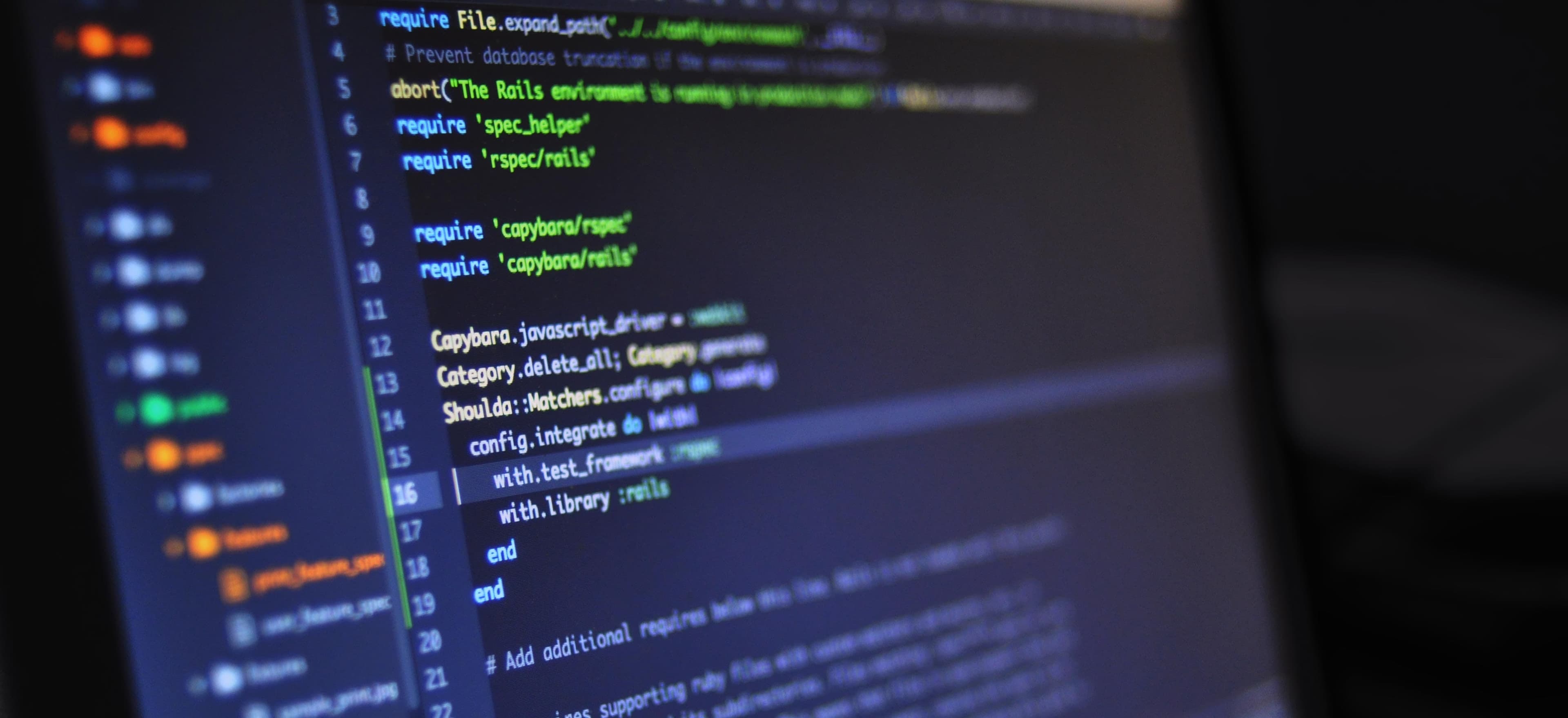
- Published on
In the world of web development, creating modular and extensible applications is crucial for scalability and maintainability. One way to achieve this is by implementing a flexible plugin system that allows developers to extend the functionality of the application without modifying its core codebase.
In this article, we will explore how to implement a flexible plugin system in Java web applications. We'll discuss the benefits of using a plugin system and walk through the steps to create one. By the end of this article, you will have a solid understanding of how to design and build a robust plugin architecture for your Java web applications.
Why Use a Plugin System?
Before diving into the implementation details, let's first understand why using a plugin system can be beneficial for Java web applications.
-
Modularity: A plugin system promotes modularity by allowing developers to encapsulate and separate distinct pieces of functionality. This makes it easier to manage, test, and evolve the codebase.
-
Extensibility: By providing extension points, a plugin system enables developers to add new features or modify existing ones without having to change the core application code. This enhances the flexibility and adaptability of the system.
-
Customization: With a plugin system in place, end users can customize the application to suit their specific requirements by installing or developing plugins without altering the original source code.
Designing the Plugin System
Now that we understand the benefits, let's discuss how to design and implement a plugin system for Java web applications.
1. Define Extension Points
The first step is to identify the extension points in the application where plugins can hook into. These extension points represent specific areas or functionalities within the application that can be extended or modified.
For instance, in a content management system, an extension point could be defined for adding custom authentication mechanisms, integrating new content types, or extending the admin dashboard with custom widgets.
2. Develop a Plugin Interface
Once the extension points are identified, define a plugin interface that outlines the contract plugins must adhere to. This interface should specify the methods or hooks that plugins can implement to integrate with the application.
Here's an example of a simple plugin interface for a content management system:
public interface CmsPlugin {
void initialize();
void onPostPublish(Post post);
void onUserLogin(User user);
// Additional hooks and methods
}
The CmsPlugin
interface declares methods that a plugin can implement to initialize itself and respond to events such as post publishing and user login.
3. Implement Plugin Loading Mechanism
Next, create a mechanism to discover, load, and manage plugins within the application. Java's built-in reflection API can be utilized to dynamically load and instantiate plugin classes at runtime.
When the application starts, it can scan predefined directories or classpath locations for classes that implement the plugin interface. These classes can then be instantiated and registered as active plugins within the system.
4. Provide Configuration and Lifecycle Management
To make the plugin system more robust, consider adding support for configuration and lifecycle management. Plugins may require configuration parameters, and they should have the ability to perform initialization and cleanup operations.
Keeping the plugin lifecycle in mind is crucial for ensuring that plugins can be dynamically loaded, enabled, disabled, and unloaded without disrupting the application's operation.
Example Implementation
Let's walk through a simplified implementation of a plugin system for a Java web application using the Spring Framework.
1. Define the Plugin Interface
Create a simple plugin interface that defines the contract for all plugins:
public interface WebPlugin {
void initialize();
void shutdown();
}
2. Implement a Plugin
Develop a concrete plugin by implementing the plugin interface:
public class LoggingPlugin implements WebPlugin {
@Override
public void initialize() {
// Initialization logic such as setting up loggers
}
@Override
public void shutdown() {
// Cleanup logic such as closing open resources
}
}
3. Discover and Load Plugins
Utilize Spring's component scanning to automatically discover and instantiate all classes implementing the WebPlugin
interface:
@Configuration
@ComponentScan("com.example.plugins")
public class PluginConfig {
// Spring configuration for plugin scanning
}
4. Managing Plugin Lifecycle
Integrate the plugin lifecycle with the application context to ensure proper initialization and shutdown:
public class PluginManager {
@Autowired
private ApplicationContext applicationContext;
public void initializePlugins() {
Map<String, WebPlugin> plugins = applicationContext.getBeansOfType(WebPlugin.class);
plugins.values().forEach(WebPlugin::initialize);
}
public void shutdownPlugins() {
Map<String, WebPlugin> plugins = applicationContext.getBeansOfType(WebPlugin.class);
plugins.values().forEach(WebPlugin::shutdown);
}
}
In this example, Spring's component scanning is used to automatically discover and instantiate all classes implementing the WebPlugin
interface. The PluginManager
is responsible for initializing and shutting down the plugins managed by the Spring application context.
The Last Word
In this article, we've explored the importance of implementing a flexible plugin system for Java web applications and discussed the steps involved in designing and building one. By following the guidelines outlined here, you can create a modular, extensible, and customizable architecture for your web applications, empowering both developers and end users to enhance the functionality of the system.
Remember, a well-designed plugin system can significantly contribute to the success and longevity of your Java web applications, so invest the time and effort to architect it thoughtfully.
Implementing a flexible plugin system is a powerful tool in a developer's arsenal. By doing so, you're not only making your code more maintainable and scalable, but you're also opening the doors for the community to extend and customize your application.
By providing extension points, defining clear plugin interfaces, and managing plugin lifecycle, you can ensure that your Java web application can grow and adapt to meet evolving requirements without becoming bloated or monolithic.
So, go ahead and start designing your plugin system. Your future self and your fellow developers will thank you for it.
Happy coding!
Checkout our other articles