Debugging Common Java Issues on Apache HTTP Server
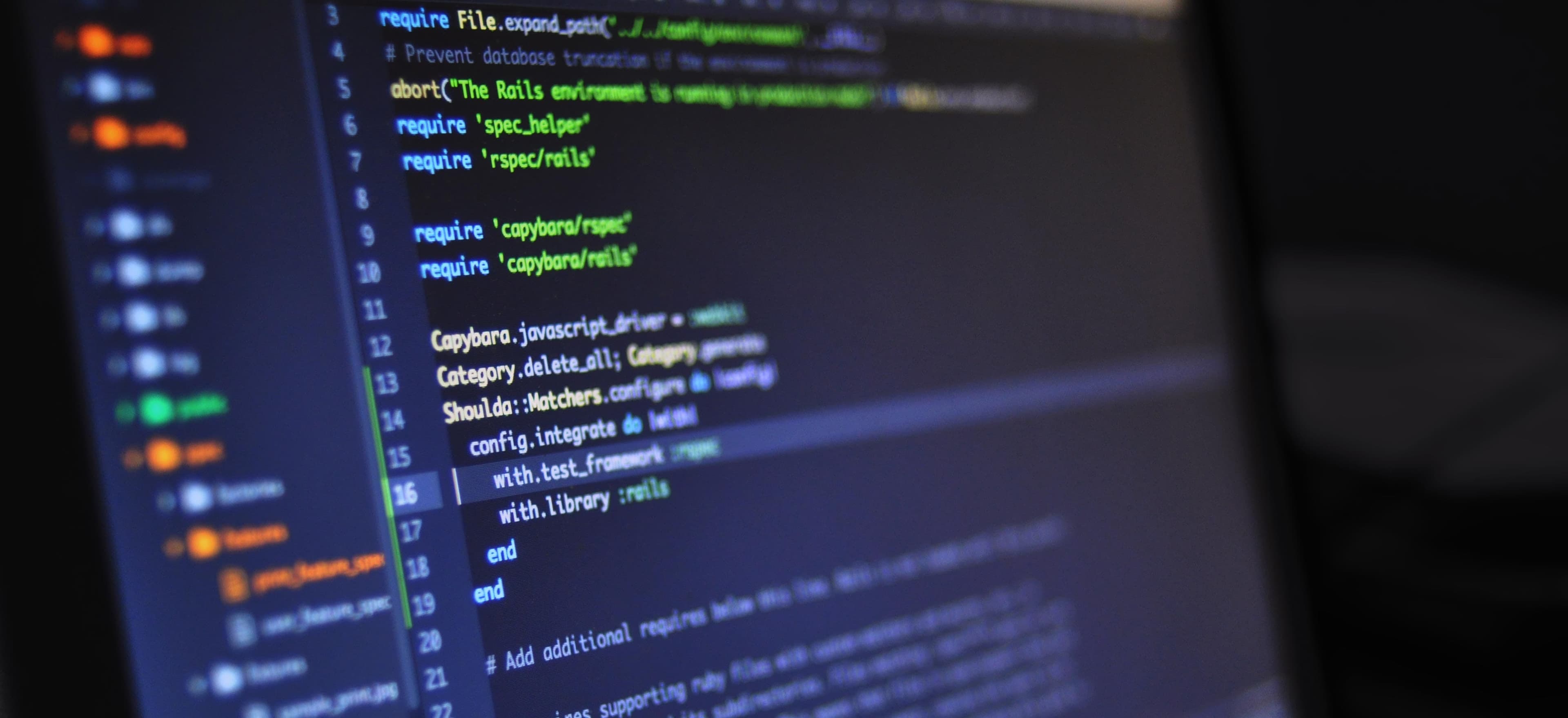
- Published on
Debugging Common Java Issues on Apache HTTP Server
Apache HTTP Server is one of the most widely used web servers for hosting Java applications. However, like any complex software environment, it can encounter issues that may complicate application deployment and server management. Debugging these issues can save time and reduce potential downtime, ensuring the smooth operation of your Java applications. In this post, we will explore common Java issues that might arise when using Apache HTTP Server, their potential causes, and how to resolve them.
Table of Contents
- Common Java Issues
- 1.1 OutOfMemoryError
- 1.2 ClassNotFoundException
- 1.3 NullPointerException
- 1.4 Connection Timeout
- Effective Debugging Techniques
- 2.1 Logging
- 2.2 Thread Dumps
- 2.3 Profiling
- Conclusion
Common Java Issues
OutOfMemoryError
One of the most notorious issues developers face is OutOfMemoryError
. This occurs when the Java Virtual Machine (JVM) cannot allocate memory required for creating objects.
Causes
- Poorly optimized code that retains references to objects no longer in use.
- Heavy loads that require more memory than allocated to the JVM.
Solution
To mitigate this error, consider the following:
-
Increase JVM Memory: Use the
-Xms
and-Xmx
flags to set the initial and maximum heap sizes.java -Xms512m -Xmx2048m -jar yourapp.jar
This command initializes the JVM with 512 MB and allows it to use up to 2048 MB of memory.
-
Memory Leak Detection: Utilize tools like VisualVM or Eclipse Memory Analyzer to identify leaks by observing memory usage patterns.
ClassNotFoundException
Another common error is ClassNotFoundException
, which occurs when the JVM cannot find a specified class.
Causes
- Classpath issues, such as not including JAR files or directories holding classes.
Solution
To resolve this:
-
Check Classpath: Ensure all necessary libraries are included in the classpath. You can set the classpath using the
-cp
or-classpath
option.java -cp .:lib/* your.package.MainClass
-
Verify Library Versions: Make sure that the correct versions of the libraries are referenced, as incompatible versions may lead to classes not being found.
NullPointerException
NullPointerException
is one of the most common runtime exceptions and occurs when the JVM attempts to access a method or property of an object that is null
.
Causes
- Uninitialized object references.
- Accessing elements from collections that might be empty.
Solution
Prevent NullPointerException
by following best practices:
-
Avoid Null References: Implement defensive programming techniques by checking if objects are null before accessing them.
if (myObject != null) { myObject.someMethod(); } else { System.out.println("myObject is null"); }
-
Use Optional: Java 8 introduced the
Optional
class to help manage nullable references.Optional<MyObject> optionalObj = Optional.ofNullable(myObject); optionalObj.ifPresent(MyObject::someMethod);
Connection Timeout
Connection timeout issues can occur when Apache HTTP Server cannot reach your Java application, often throwing a 504 Gateway Timeout
error.
Causes
- Network issues.
- Long-running operations in your Java application.
- Insufficient server resources.
Solution
To troubleshoot connection timeouts:
-
Increase Timeout Settings: Adjust Apache's
Timeout
directive and proxy settings in your configuration.Timeout 300 ProxyTimeout 300
-
Optimize Java Application: Profile your application to identify bottlenecks, ensuring long operations are either optimized or run asynchronously.
Effective Debugging Techniques
When tracking down these issues, various methods can greatly assist in diagnosing problems.
Logging
Logging is an indispensable tool in any developer's toolkit. Using comprehensive logging frameworks, such as Log4j or SLF4J, can help you track down issues.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApplication {
private static final Logger logger = LoggerFactory.getLogger(MyApplication.class);
public void performAction() {
logger.info("Action started");
// action code
logger.info("Action completed");
}
}
Logging allows you to maintain a record of the application run, making it easier to backtrack from errors.
Thread Dumps
In cases of high CPU usage or deadlocks, thread dumps can provide insights into what threads are currently executing and what resources they hold.
To generate a thread dump, use the following command:
jstack <pid>
Replace <pid>
with the process ID of your Java application. This will help you visualize the state of all threads and can pinpoint where your application might be stuck.
Profiling
Profiling tools help identify performance issues within your applications. Tools like YourKit or JProfiler can highlight slow methods and memory consumption.
To use a profiling tool effectively:
- Integrate the profiler into your Java application during testing or staging phases.
- Analyze the reports generated to pinpoint inefficiencies.
Wrapping Up
Debugging Java applications running on Apache HTTP Server is a critical skill that can significantly enhance your development workflow and improve application performance. By understanding common issues such as OutOfMemoryError
, ClassNotFoundException
, NullPointerException
, and connection timeouts, you can take proactive steps to mitigate these risks. Implementing effective debugging techniques, including thorough logging, thread dumps, and profiling, will enable you to diagnose and resolve issues swiftly.
By honing your debugging skills, you not only ensure that your applications run more smoothly but also enhance your effectiveness as a Java developer.
For more insights on optimizing Java applications, check out this Java Performance Tuning Guide.
Happy coding!
Checkout our other articles