Understanding the Risks of Correlating Subtype and Generic Polymorphism
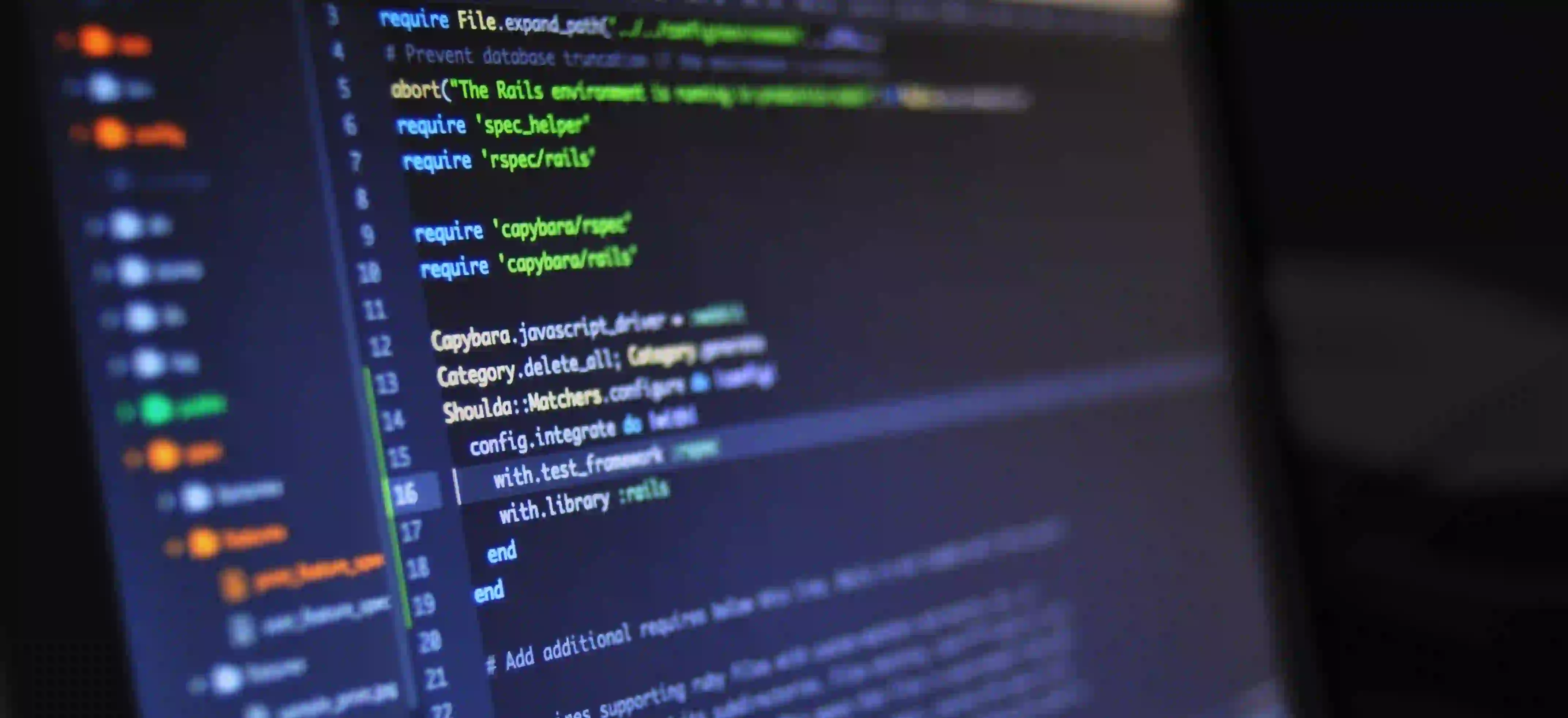
Understanding the Risks of Correlating Subtype and Generic Polymorphism
In the world of Java programming, polymorphism stands as one of the essential pillars of object-oriented programming (OOP). However, misconceptions and misinterpretations often arise when dealing with subtyping and generics. This article delves deep into the potential risks associated with inaccurately correlating subtype and generic polymorphism, shedding light on the intricacies and offering clarity on their distinctions.
The Essence of Polymorphism
At its core, polymorphism allows a single interface to represent various underlying forms. This flexibility brings in considerable advantages, enabling code to be written in a more generic fashion, thereby increasing reusability and flexibility.
Subtype Polymorphism
Subtype polymorphism, commonly known as dynamic polymorphism, comes into play when a class inherits from another class and provides its specific implementation of the inherited behavior. This allows one to treat objects of the derived class as objects of the base class, thereby enabling flexibility and extensibility in the system.
Generic Polymorphism
On the other hand, generic polymorphism, or parametric polymorphism, revolves around the concept of writing code in a way that is independent of a particular type. This is achieved through the use of generics, enabling classes, interfaces, and methods to operate on objects of various types while providing compile-time type safety.
Understanding the Risks
Correlating Subtype and Generic Polymorphism
The crux of the matter lies in understanding that while subtype polymorphism deals with inheritance and subtyping at runtime, generic polymorphism focuses on compile-time type safety. Subtype polymorphism is more concerned with the behavior of classes and their instances at runtime, whereas generic polymorphism emphasizes compile-time type checking, providing additional reassurance against type-related errors.
Example of Subtype Polymorphism
Let's consider an example where we have a base class Shape
and derived classes Circle
and Rectangle
. The subtype polymorphism allows us to write a method that operates on Shape
objects, and during runtime, the specific implementation from Circle
or Rectangle
will be invoked based on the actual object type.
public class Shape {
public void draw() {
// Draw the shape
}
}
public class Circle extends Shape {
@Override
public void draw() {
// Draw the circle
}
}
public class Rectangle extends Shape {
@Override
public void draw() {
// Draw the rectangle
}
}
Example of Generic Polymorphism
Now, let's consider an example of generic polymorphism using Java generics. Here, we have a generic class Box
that can hold objects of any type. This ensures type safety at compile time while allowing flexibility in using different types of objects with the same class.
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Risks of Correlation
The risks arise when one mistakenly tries to intertwine subtype and generic polymorphism, assuming that they operate on the same principles. In doing so, the essence of each polymorphic concept diminishes, and the code becomes susceptible to logical and type-related errors. Misusing generics to handle subtype polymorphism can lead to unexpected runtime behaviors and violate the principles of type safety that generics aim to enforce.
Best Practices
To navigate the potential risks of correlating subtype and generic polymorphism, it is crucial to adhere to best practices that honor the distinctions between the two concepts.
-
When dealing with subtype polymorphism, focus on leveraging inheritance, interfaces, and runtime polymorphism to encapsulate behavior and facilitate flexibility.
-
When utilizing generic polymorphism, embrace the power of Java generics to write more generic and reusable code while upholding compile-time type safety.
My Closing Thoughts on the Matter
In conclusion, while both subtype and generic polymorphism contribute significantly to the power and flexibility of Java programming, it is vital to acknowledge their independent functionalities and not conflate their principles. By understanding the risks associated with correlating subtype and generic polymorphism and adhering to best practices, developers can ensure robust, maintainable, and error-resilient code that upholds the core tenets of both subtype and generic polymorphism.
In Java, polymorphism serves as a cornerstone of object-oriented programming, and by grasping its nuances and intricacies, developers can harness its full potential while mitigating the risks of misinterpretation and misapplication.