Optimizing Kotlin Performance with GraalVM C2
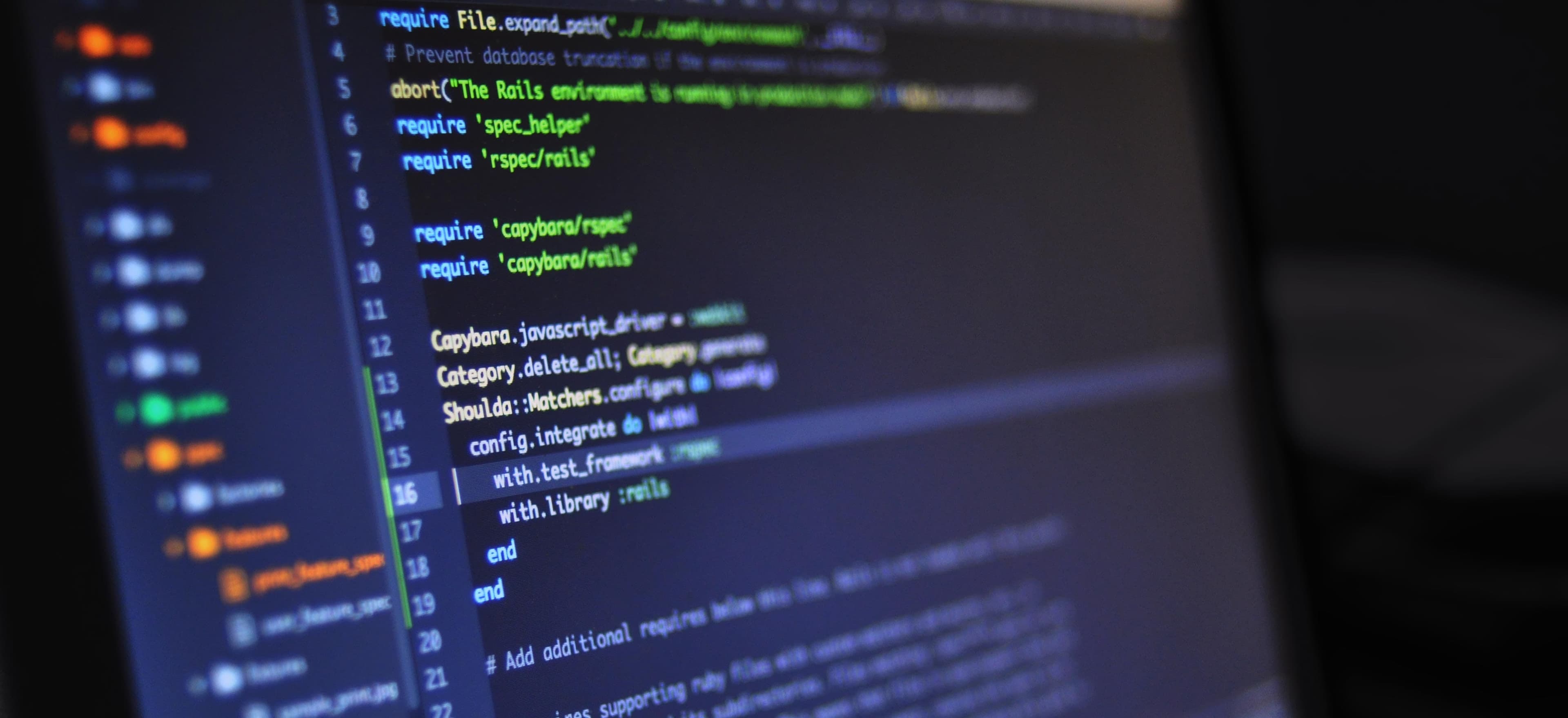
- Published on
Optimizing Java Performance with GraalVM's C2 Compiler
GraalVM is a high-performance runtime that provides a polyglot environment for running applications written in multiple languages, including Java, JavaScript, Python, and more. One of the key components of GraalVM is the C2 compiler, which is responsible for translating Java bytecode into optimized machine code. In this article, we'll take a deep dive into how the C2 compiler works and explore best practices for optimizing Java performance with GraalVM.
Understanding the C2 Compiler
The C2 compiler, also known as the "server" compiler, is a key component of the Java Virtual Machine (JVM) that performs just-in-time (JIT) compilation of Java bytecode into highly-optimized native machine code. This process involves various stages such as parsing, optimizing, and generating machine code.
When a Java application runs on GraalVM, the C2 compiler kicks in to analyze the hotspots in the code, i.e., the parts of the code that are executed frequently, and applies aggressive optimization techniques to improve performance.
Leveraging C2 Compiler Optimizations
To take full advantage of the C2 compiler optimizations, it's essential to write Java code that aligns well with the compiler's optimization strategies. Here are some best practices to consider:
Use Final and Inline
public final class PerformanceOptimization {
private static final int CONSTANT = 10;
public int calculate(int input) {
return input * CONSTANT;
}
}
In the above example, marking the class as final
and the CONSTANT
as final
enables the C2 compiler to perform inlining and constant folding, leading to more efficient code execution.
Minimize Synchronization
Excessive synchronization can hinder the C2 compiler's ability to optimize the code due to potential data dependencies and race conditions. Consider the use of lock-free data structures or alternative synchronization mechanisms for improved performance.
Profile-guided Optimization
GraalVM's C2 compiler supports profile-guided optimization, which involves collecting runtime profiling information to guide the optimization process. It's advisable to use workload representative of the actual production environment to generate accurate profiles for effective optimization.
Enabling GraalVM's C2 Compiler
To enable GraalVM's C2 compiler for Java applications, you can use the following command line option:
java -XX:+UseJVMCICompiler
The -XX:+UseJVMCICompiler
flag instructs the JVM to use the JVM Compiler Interface (JVMCI), which enables GraalVM's C2 compiler. Additionally, you can further fine-tune the behavior of the C2 compiler by specifying advanced options such as -XX:CompileThreshold
and -XX:CompileCommand
.
Monitoring C2 Compiler Activity
GraalVM provides tools for monitoring the activity of the C2 compiler to gain insights into its optimizations and generated machine code. The PrintCompilation
and PrintInlining
options can be used to output detailed information about the compilation and inlining processes, allowing developers to analyze the performance impact of the C2 compiler’s optimizations on their Java applications.
Final Considerations
In conclusion, GraalVM's C2 compiler plays a crucial role in optimizing the performance of Java applications running on GraalVM. By understanding the inner workings of the C2 compiler and adopting best practices for writing performance-oriented Java code, developers can harness the full potential of GraalVM's C2 compiler to achieve significant performance improvements.
With its ability to leverage the advanced optimizations provided by the C2 compiler, GraalVM stands out as a powerful platform for executing high-performance Java applications.
To further explore GraalVM and its C2 compiler, refer to the official GraalVM documentation and GraalVM GitHub repository.
Checkout our other articles