Managing Module-specific Property Files in Spring Boot
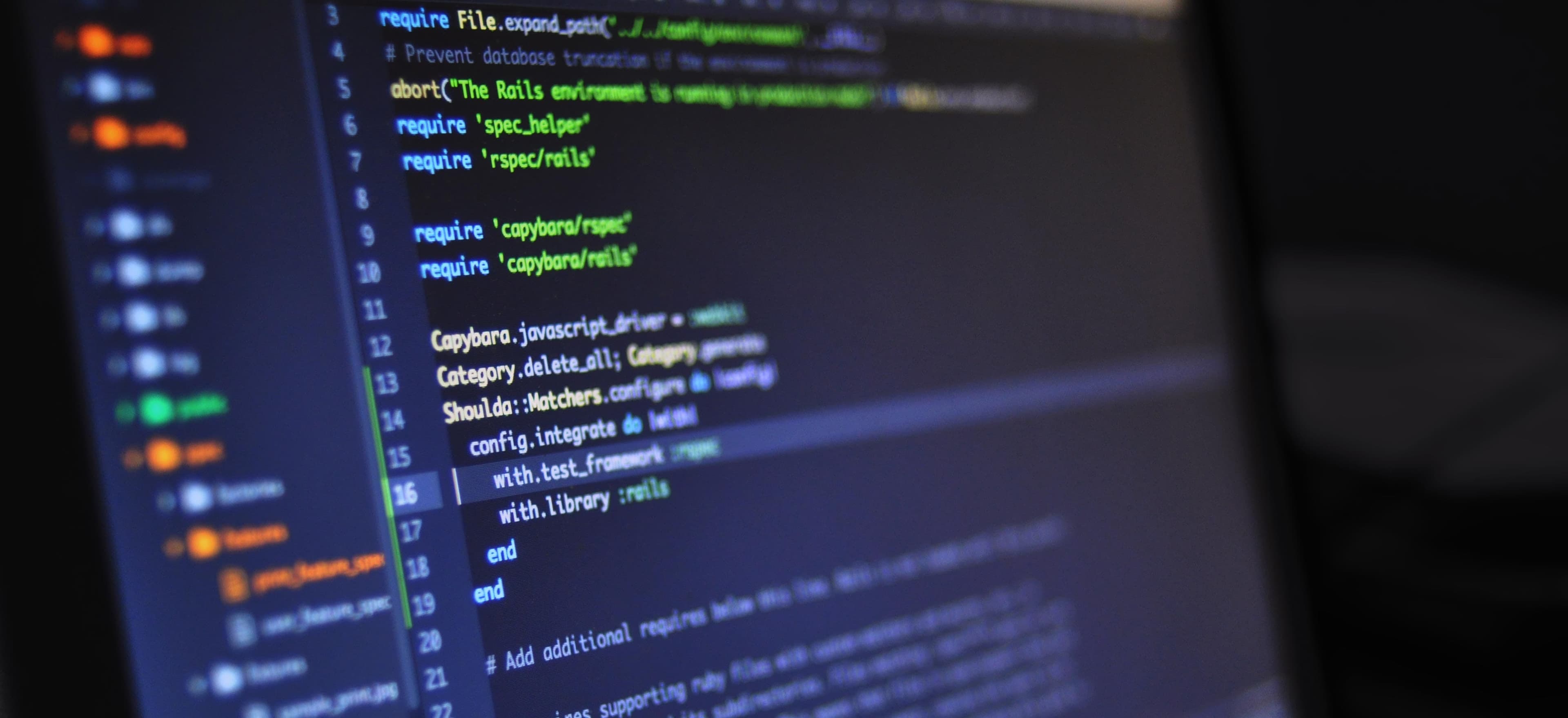
- Published on
Managing Module-specific Property Files in Spring Boot
When developing a Spring Boot application, it's common to have module-specific configurations that need to be managed separately. This allows for easier maintenance and configuration of individual modules without affecting the entire application. In this article, we'll explore how to manage module-specific property files in a Spring Boot application.
Understanding the Problem
In a typical Spring Boot application, the application.properties
or application.yml
file is used to store global configurations for the entire application. However, as the application grows, it becomes more challenging to manage module-specific configurations within this single file. This is especially true when different modules have their own configuration needs that may conflict with each other.
Managing module-specific property files alleviates this issue by allowing each module to have its own dedicated configuration file. This not only keeps configurations organized but also simplifies the process of understanding and maintaining module-specific settings.
Creating Module-specific Property Files
To begin, let's create separate property files for each module. For example, if we have two modules named module1
and module2
, we can create property files for each as follows:
module1.properties
module1.property1=value1
module1.property2=value2
module2.properties
module2.property3=value3
module2.property4=value4
It's worth noting that we could also use YAML format (module1.yml, module2.yml) based on our preference for the property file format.
Loading Module-specific Property Files
In a Spring Boot application, we can leverage the @PropertySource
annotation to load module-specific property files. This annotation is used to declare a property source and provide the location of the property file.
Let’s create a configuration class to load the module-specific property files:
ModuleConfig.java
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
@Configuration
@PropertySource("classpath:module1.properties")
@PropertySource("classpath:module2.properties")
public class ModuleConfig {
// Configuration specific to module properties
}
In the above example, @PropertySource
is used to specify the location of the module-specific property files. These files are then loaded into the application context, making the properties accessible for injection into other components.
Accessing Module-specific Properties
Once the module-specific property files are loaded, we can access the properties in a type-safe manner using the @Value
annotation.
Let’s explore how we can use the @Value
annotation to access the module-specific properties within a component:
ModuleComponent.java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class ModuleComponent {
@Value("${module1.property1}")
private String module1Property1;
@Value("${module2.property3}")
private String module2Property3;
// Other module-specific properties
// Accessors and Mutators
}
In the above example, the @Value
annotation is used to inject module-specific properties directly into the component. This allows for easy access to module-specific configurations within the code.
Why Managing Module-specific Property Files is Important
Managing module-specific property files is crucial for the following reasons:
-
Modularity: It promotes modularity by encapsulating configurations specific to each module, leading to a more maintainable and scalable codebase.
-
Separation of Concerns: It separates the concerns of different modules, making it easier to understand and manage configurations within each module.
-
Reduced Complexity: It simplifies the process of configuration management by breaking down the global configuration into smaller, more manageable parts.
-
Avoiding Conflicts: It helps prevent conflicts between module-specific configurations that may occur if all properties are maintained in a single file.
Closing the Chapter
In a Spring Boot application, managing module-specific property files is a best practice for organizing and maintaining module-specific configurations. By creating dedicated property files for each module, loading them into the application context, and accessing them in a type-safe manner, we can effectively manage module-specific configurations without cluttering the global configuration files.
This approach not only enhances the modularity and maintainability of the application but also simplifies the process of configuration management, ultimately leading to a more robust and scalable codebase.
By following these best practices, developers can ensure that their Spring Boot applications remain organized, maintainable, and easy to scale as the project grows.
Incorporating module-specific property files is a small but powerful step towards better organization and maintenance of Spring Boot applications.
Now, you can start organizing your module-specific configurations in a more streamlined and efficient manner, leading to a more robust and sustainable application architecture.
A comprehensive understanding of managing module-specific properties is vital for enhancing the organization and scalability of Spring Boot applications. By leveraging this practice, developers can achieve a more maintainable and robust codebase. If you're interested in learning more about Spring Boot, check out Spring Boot Documentation for further insights.
Checkout our other articles