Optimizing Performance in JPA Entity Mapping
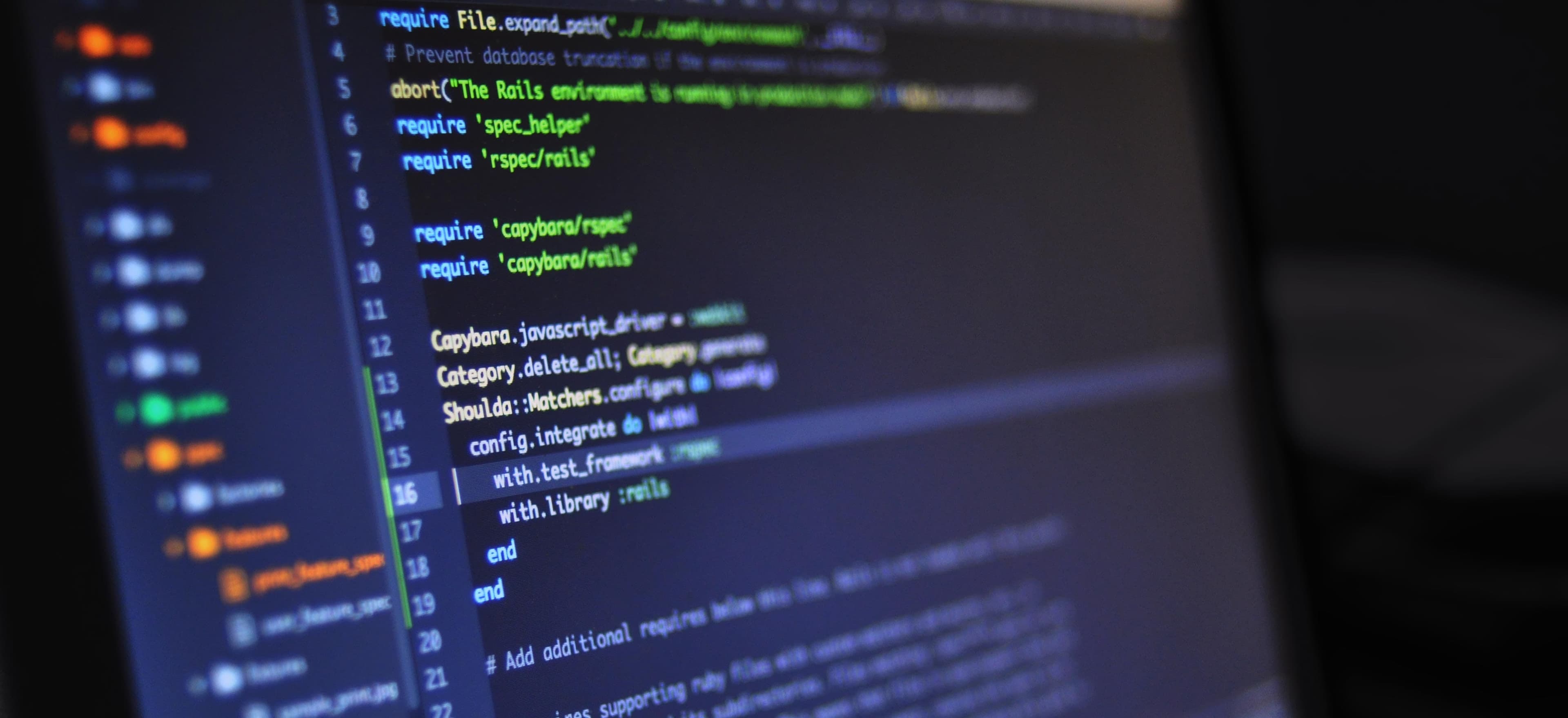
- Published on
Optimizing Performance in JPA Entity Mapping
When working with Java Persistence API (JPA), it's important to optimize the performance of entity mapping to ensure efficient data retrieval and manipulation. In this article, we'll explore some best practices for optimizing performance in JPA entity mapping.
Use Lazy Loading for Relationships
One of the key aspects of optimizing performance in JPA entity mapping is to use lazy loading for relationships. By default, JPA uses eager loading, which means that associated entities are loaded from the database immediately when the owning entity is fetched. This can lead to performance issues, especially when dealing with large datasets or complex object graphs.
To address this, you can use lazy loading for relationships by annotating the relationship with @OneToMany
or @ManyToOne
and specifying the FetchType.LAZY
. This ensures that the associated entities are loaded from the database only when they are accessed for the first time.
@Entity
public class Order {
// ...
@OneToMany(fetch = FetchType.LAZY, mappedBy = "order")
private List<OrderItem> items;
// ...
}
By using lazy loading, you can minimize the amount of data fetched from the database, thereby improving the overall performance of your JPA entity mappings.
Avoid N+1 Query Problem
Another common performance issue in JPA entity mapping is the N+1 query problem, where multiple additional queries are executed to fetch related entities for each parent entity. This can result in a large number of database queries, leading to significant performance overhead.
To mitigate the N+1 query problem, you can use entity graph fetching to define a graph of entities to be fetched in a single query. This can be achieved using the @NamedEntityGraph
annotation to specify the entity graph and the @EntityGraph
annotation to apply it to a specific query.
@Entity
@NamedEntityGraph(name = "order-items-graph", attributeNodes = @NamedAttributeNode("items"))
public class Order {
// ...
}
@Repository
public interface OrderRepository extends JpaRepository<Order, Long> {
@EntityGraph(value = "order-items-graph", type = EntityGraphType.LOAD)
Optional<Order> findById(Long id);
}
By using entity graph fetching, you can reduce the number of database queries and improve the performance of your JPA entity mappings.
Use Second-Level Caching
In JPA, second-level caching can be employed to cache entity data at the session factory or persistence unit level. This can help reduce the number of database queries by caching entity data in memory, thereby improving the performance of entity mappings.
To enable second-level caching, you can use the @Cacheable
annotation on your entities and configure a caching provider such as Ehcache or Infinispan. Additionally, you can specify caching options such as eviction policies and time-to-live settings to control the behavior of the cache.
@Entity
@Cacheable
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class Product {
// ...
}
By leveraging second-level caching, you can enhance the performance of entity mappings by reducing the load on the database and speeding up data retrieval.
Batch Fetching for Improved Performance
When working with JPA entity mappings, batch fetching can be used to optimize performance by fetching multiple entities in a single query. This can help reduce the number of round trips to the database and improve the overall efficiency of data retrieval.
You can utilize batch fetching by specifying the @BatchSize
annotation on associations, which allows you to define the batch size for fetching associated entities.
@Entity
public class Customer {
// ...
@OneToMany(mappedBy = "customer")
@BatchSize(size = 10)
private List<Order> orders;
// ...
}
By using batch fetching, you can minimize the overhead of executing multiple queries and enhance the performance of your JPA entity mappings.
Closing the Chapter
Optimizing performance in JPA entity mapping is crucial for ensuring efficient data retrieval and manipulation. By utilizing techniques such as lazy loading, entity graph fetching, second-level caching, and batch fetching, you can significantly improve the performance of your JPA entity mappings and enhance the overall responsiveness of your applications.
Incorporating these best practices will help you build high-performing JPA applications that deliver optimal user experiences and efficient data processing.
For further reading on optimizing JPA performance, you can check out the Hibernate Performance Tuning guide from the official Hibernate documentation and the JPA Performance Optimization article on Baeldung.
Remember, optimizing JPA entity mapping performance is an ongoing process, and it's essential to profile and analyze your application's performance regularly to identify and address any potential bottlenecks.
Happy coding!
Checkout our other articles