Optimizing Java Performance: Understanding the JDK 13 AggressiveOpts Flag
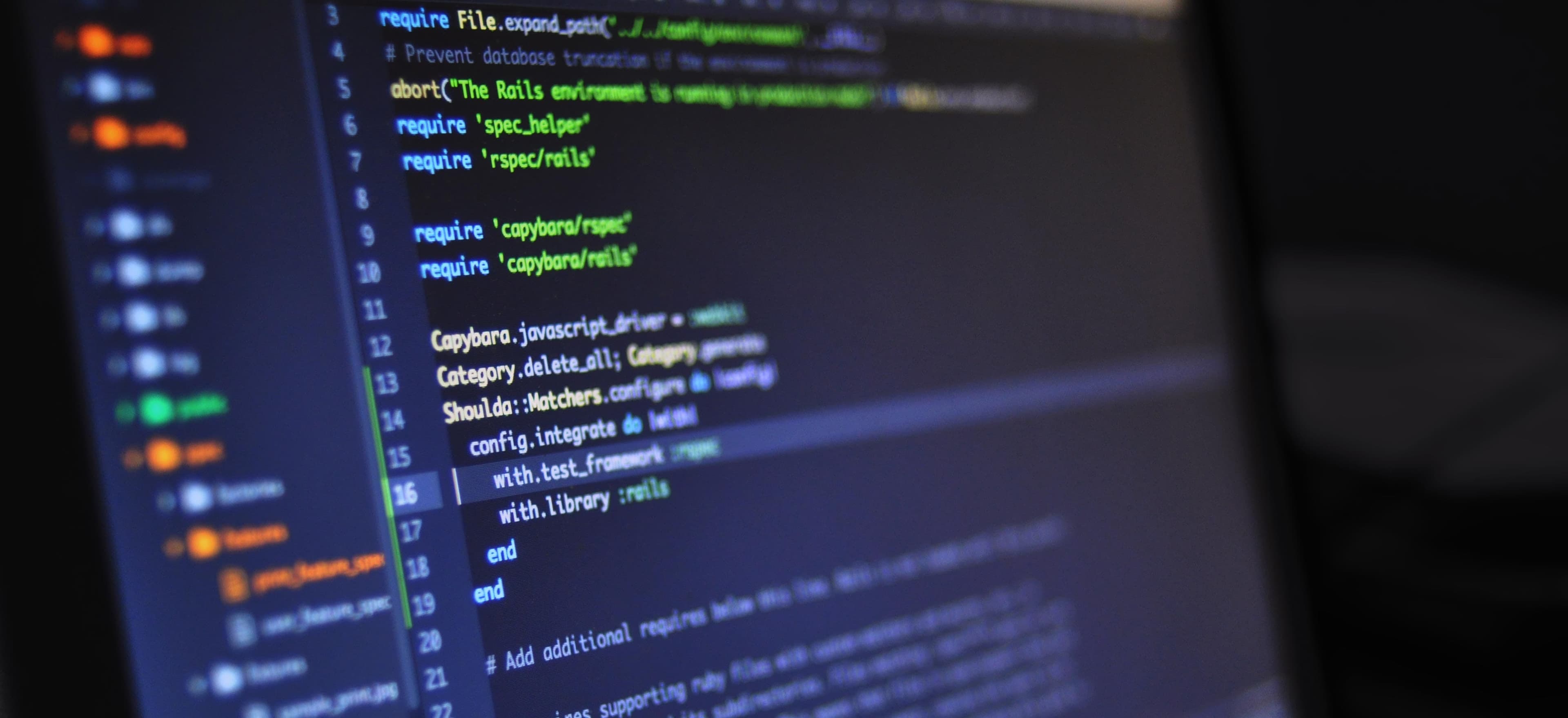
- Published on
Understanding the JDK 13 AggressiveOpts Flag for Optimizing Java Performance
Java developers are always on the lookout for ways to improve the performance of their applications. While there are various techniques and tools available for optimizing Java performance, one often overlooked method is leveraging the AggressiveOpts flag provided by the Java Development Kit (JDK).
In this article, we will delve into the AggressiveOpts flag, available in JDK 13, and explore how it can be utilized to enhance the performance of Java applications. We will also discuss the potential impact of using this flag and provide examples to illustrate its effectiveness.
What is the AggressiveOpts Flag?
The AggressiveOpts flag is a feature introduced in the JDK that enables a set of aggressive performance optimizations at the cost of increased compilation time and potentially higher resource consumption. When enabled, the Java Virtual Machine (JVM) applies a collection of aggressive optimizations to improve the runtime performance of Java applications.
It's important to note that the AggressiveOpts flag is not enabled by default and should be used with caution, as it can significantly impact the behavior of the JVM and the performance of the application.
Enabling the AggressiveOpts Flag
To enable the AggressiveOpts flag, you can simply add the following command-line option when launching your Java application:
java -XX:+AggressiveOpts YourApplication
By including the -XX:+AggressiveOpts
option, you are instructing the JVM to enable the aggressive performance optimizations.
Understanding the Impact
While enabling the AggressiveOpts flag can potentially lead to improved performance, it's crucial to understand the impact it may have on your Java application. The aggressive optimizations applied by the JVM may result in increased compilation time, higher memory usage, and varying effects on different hardware architectures.
Additionally, the AggressiveOpts flag may cause the JVM to generate different code patterns, potentially affecting the behavior of the application. Therefore, it's essential to thoroughly test the application under the influence of AggressiveOpts to ensure that the optimizations do not introduce unexpected issues.
Examples of AggressiveOpts in Action
Let's explore some examples to demonstrate the impact of the AggressiveOpts flag on Java application performance.
Example 1: Microbenchmark
Consider a simple microbenchmark that calculates the factorial of a given number using recursion. First, let's run the benchmark without the AggressiveOpts flag:
public class FactorialBenchmark {
public static void main(String[] args) {
long start = System.nanoTime();
long result = factorial(20);
long end = System.nanoTime();
System.out.println("Factorial: " + result);
System.out.println("Time taken: " + (end - start) + " ns");
}
private static long factorial(int n) {
if (n <= 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
}
Upon running the benchmark without AggressiveOpts, we observe the execution time.
Next, let's enable the AggressiveOpts flag and rerun the benchmark to compare the performance.
Example 2: Application Profiling
In a more complex scenario, consider a multi-threaded application that performs intensive computations. By profiling the application's performance using tools like JVisualVM or YourKit, you can analyze the impact of enabling AggressiveOpts on the application's runtime behavior, CPU utilization, and memory consumption.
By examining the profiler results before and after enabling AggressiveOpts, you can gain insight into the effectiveness of the aggressive optimizations and identify potential areas of improvement or regression.
Wrapping Up
The AggressiveOpts flag in the JDK 13 provides a powerful yet delicate mechanism to unlock performance optimizations in Java applications. When used judiciously and in conjunction with thorough testing and profiling, it can yield significant performance improvements.
However, it's essential to approach the utilization of AggressiveOpts with caution and make informed decisions based on the specific requirements and characteristics of the Java application. Understanding the potential impact and testing the application under various scenarios are crucial steps in harnessing the benefits of this feature.
In conclusion, the AggressiveOpts flag represents a valuable tool in the arsenal of Java performance optimization, empowering developers to fine-tune the behavior of the JVM and extract optimal performance from their applications.
By leveraging the AggressiveOpts flag, Java developers can potentially unlock significant performance improvements in their applications. Understanding the impact and judiciously testing the effects are critical steps in harnessing the full potential of this feature. If you're interested in further enhancing your Java skills, you may want to explore how to optimize memory management in Java.