Mastering JUnit: Understanding Unit Test Assertions
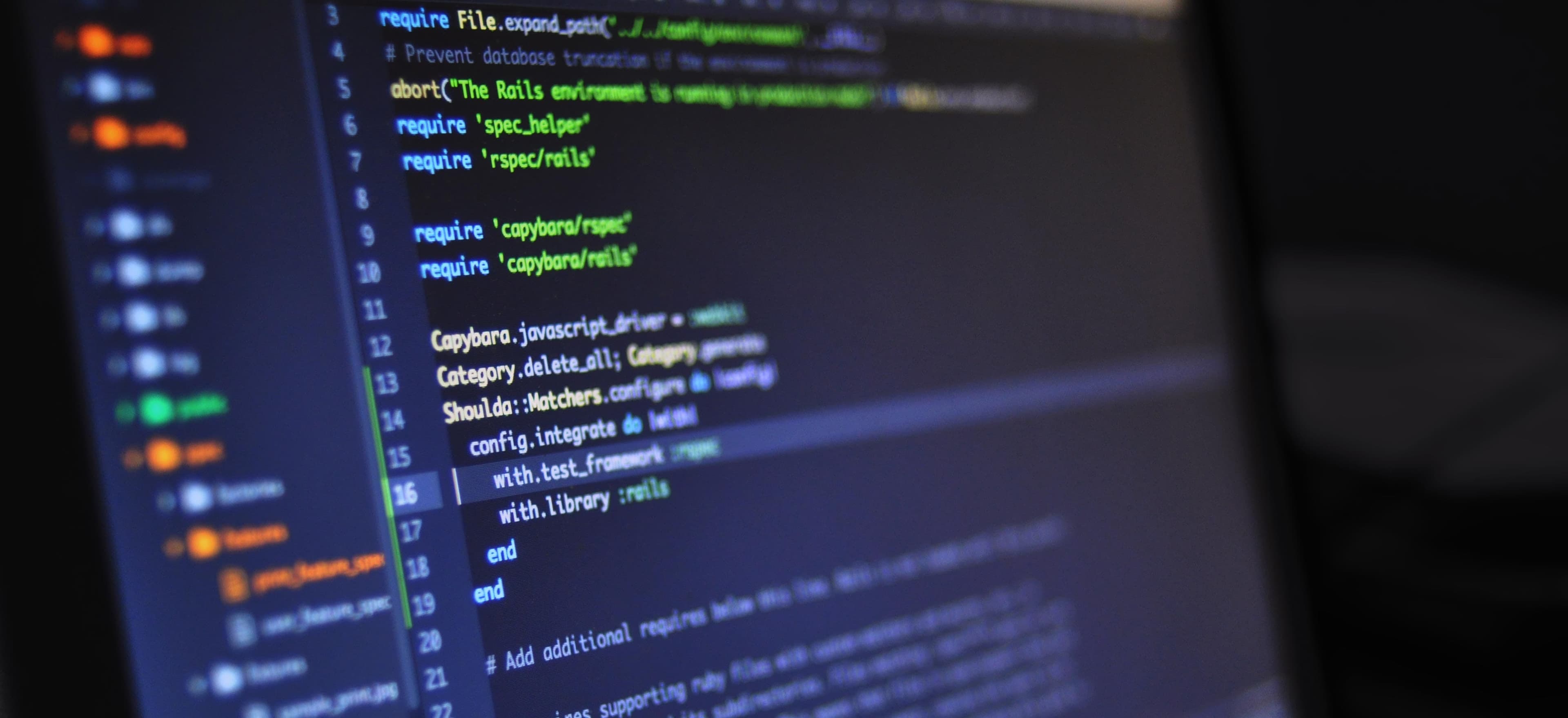
- Published on
Mastering JUnit: Understanding Unit Test Assertions
When it comes to writing robust and effective Java code, incorporating unit tests into your development process is crucial. JUnit, the most popular testing framework in the Java ecosystem, provides a comprehensive suite of features for creating and running unit tests. One of the fundamental aspects of writing unit tests with JUnit is understanding and using assertions effectively.
What Are Assertions in Unit Testing?
Assertions serve as the bedrock of unit testing, enabling developers to verify the expected behavior of their code. In the context of JUnit, assertions are used to make statements about the outcome of a test. Essentially, assertions are used to check whether the actual output of a piece of code matches the expected output. If the assertion fails, the test fails, indicating that there is a discrepancy between the actual and expected results.
The Anatomy of a JUnit Assertion
In JUnit, assertions are typically performed using static methods provided by the org.junit.Assert
class. These methods accept the actual value to be tested and an expected value, along with an optional message for clarification. Let's take a look at some of the most commonly used assertion methods in JUnit and explore how they can be used effectively.
1. assertEquals
The assertEquals
method compares the expected value with the actual value and throws an assertion error if they are not equal.
@Test
public void testAddition() {
int result = Calculator.add(3, 5);
assertEquals(8, result);
}
Why use assertEquals
?
- Ensures that the result of a method matches the expected value.
- Verifies that mathematical or logical calculations produce the correct output.
2. assertNotEquals
Conversely, the assertNotEquals
method verifies that the expected and actual values are not equal.
@Test
public void testDivision() {
double result = Calculator.divide(10, 2);
assertNotEquals(0, result);
}
Why use assertNotEquals
?
- Validates that two values are indeed different.
- Useful for testing scenarios where inequality is expected.
3. assertTrue
and assertFalse
The assertTrue
and assertFalse
methods validate whether a given condition is true or false, respectively.
@Test
public void testIsEven() {
assertTrue(MathUtils.isEven(4));
assertFalse(MathUtils.isEven(7));
}
Why use assertTrue
and assertFalse
?
- Useful for verifying logical conditions and boolean results.
- Allows for testing of conditional statements and Boolean methods.
4. assertNull
and assertNotNull
The assertNull
and assertNotNull
methods are used to check whether an object reference is null or not.
@Test
public void testFetchUserDetails() {
User user = userRepository.getUserById(123);
assertNotNull(user);
}
Why use assertNull
and assertNotNull
?
- Crucial for testing scenarios involving object references.
- Verifies the presence or absence of an expected object instance.
5. assertThrows
Introduced in JUnit 5, the assertThrows
method is used to verify that a specific exception is thrown by the code under test.
@Test
public void testWithdrawInvalidAmount() {
Account account = new Account(1000);
assertThrows(IllegalArgumentException.class, () -> account.withdraw(-100));
}
Why use assertThrows
?
- Ensures that an expected exception is thrown under certain conditions.
- Valuable for testing error-handling and exception scenarios.
Best Practices for Using Assertions in JUnit
While assertions are a cornerstone of unit testing, it's essential to adopt best practices to maximize their effectiveness. Here are some key guidelines to consider when using assertions in JUnit:
-
Clarity and Readability: Craft informative messages for assertions to provide clear context when a test fails.
-
Granularity: It's often beneficial to have assertions for individual behaviors or outcomes within a single test case, rather than combining multiple checks into one assertion.
-
Precision: Use the most suitable assertion for each scenario to ensure a precise evaluation of the test condition.
-
Maintainability: Regularly review and refine assertions as code evolves, ensuring tests remain accurate and relevant.
-
Consistency: Establish consistent naming and formatting conventions for assertions across the codebase, enhancing readability and maintainability.
The Last Word
Understanding unit test assertions and their effective utilization is essential for writing reliable and robust unit tests with JUnit. By mastering the intricacies of assertions and adhering to best practices, developers can fortify their codebases and confidently validate the correctness of their Java code. As you delve deeper into the world of unit testing, harnessing the power of assertions will undoubtedly elevate the quality and reliability of your Java applications.
To further expand your knowledge of JUnit and unit testing, consider exploring the official JUnit documentation and JUnit best practices for comprehensive insights. Happy testing!
Remember, robust and reliable code starts with effective unit testing, and a solid grasp of assertions is a key step towards achieving this goal.