Understanding Hibernate Entity Mapping: Common Mistakes
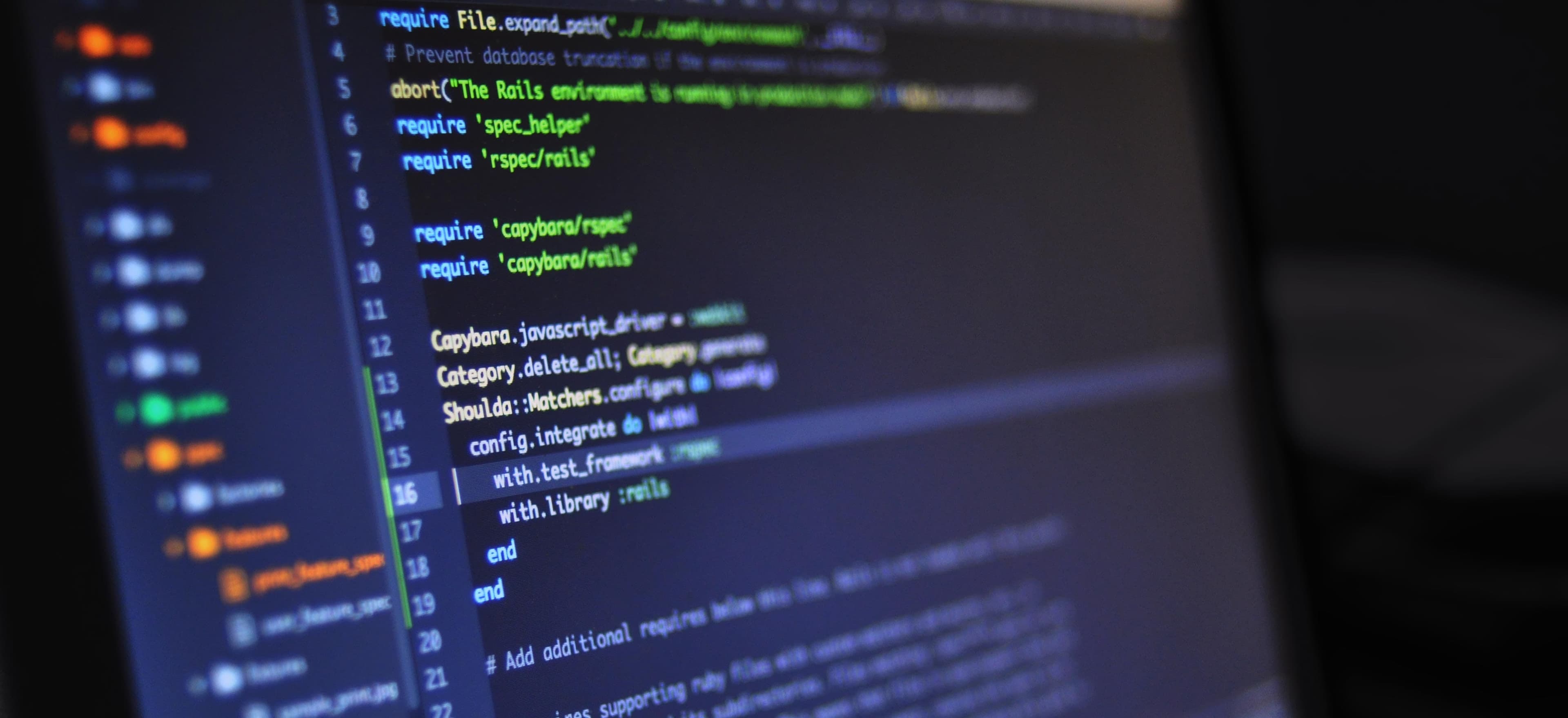
- Published on
Understanding Hibernate Entity Mapping: Common Mistakes
When it comes to working with Hibernate, one of the key aspects is entity mapping. Hibernate is an object-relational mapping (ORM) framework that provides a way to map Java objects to relational database tables. However, entity mapping can be tricky, and there are common mistakes that developers often make when working with Hibernate. In this article, we'll explore some of these common mistakes and discuss how to avoid them.
1. Misunderstanding the @Entity Annotation
In Hibernate, the @Entity
annotation is used to mark a Java class as an entity. This annotation is crucial for Hibernate to recognize the class as a persistent entity. One common mistake is to forget to add the @Entity
annotation to the entity class, which can lead to runtime errors such as org.hibernate.MappingException
.
@Entity
public class Product {
// Entity fields and methods
}
Why:
Adding the @Entity
annotation is essential because it tells Hibernate that the class should be mapped to a table in the database.
2. Incorrect Primary Key Mapping
Another mistake that developers make is incorrectly mapping the primary key of an entity. The primary key mapping is essential for Hibernate to uniquely identify each record in the database table.
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "product_id")
private Long id;
Why:
The @Id
annotation marks the field as the primary key, and the @GeneratedValue
annotation specifies the strategy for generating the primary key values. It's crucial to set the primary key mapping correctly to avoid issues with data integrity and querying.
3. Overusing Eager Fetching
Eager fetching is a strategy where related entities are loaded along with the main entity. While eager fetching can be useful in some scenarios, overusing it can lead to performance issues, especially when dealing with large datasets.
@ManyToOne(fetch = FetchType.EAGER)
@JoinColumn(name = "category_id")
private Category category;
Why:
By default, Hibernate uses lazy fetching, which defers the loading of related entities until they are explicitly accessed. It's important to carefully consider the fetching strategy to avoid unnecessary data loading and performance overhead.
4. Ignoring Cascading Operations
Cascading operations allow certain operations performed on an entity to cascade to associated entities. Forgetting to define cascading behavior can lead to inconsistent data and unexpected behavior when working with related entities.
@OneToMany(mappedBy = "category", cascade = CascadeType.ALL, orphanRemoval = true)
private Set<Product> products;
Why:
Defining cascading operations ensures that operations such as save, update, and delete are propagated from the parent entity to its associated entities, maintaining data integrity and consistency.
5. Lack of Indexing and Constraints
Indexing and constraints play a vital role in database performance and data integrity. Neglecting to define indexes and constraints on entity attributes can lead to suboptimal query performance and potential data inconsistencies.
@Column(name = "email", unique = true, nullable = false)
private String email;
Why:
Adding indexes on frequently queried columns and defining constraints such as uniqueness and nullability can significantly improve database performance and enforce data integrity at the database level.
The Last Word
In conclusion, understanding Hibernate entity mapping and avoiding common mistakes is crucial for building efficient and maintainable persistence layers. By paying attention to entity annotations, primary key mapping, fetching strategies, cascading operations, and database constraints, developers can ensure the proper functioning and performance of Hibernate-based applications.
To delve deeper into Hibernate entity mapping best practices and advanced techniques, consider exploring the official Hibernate documentation and relevant tutorials and guides.
Remember, mastery of entity mapping in Hibernate not only fosters cleaner and optimized code but also lays the foundation for seamless interaction between Java objects and relational databases. Avoiding these common pitfalls will lead to more predictable and robust data persistence solutions in your Java applications.