Mastering Test-Driven Development for Better Code Quality
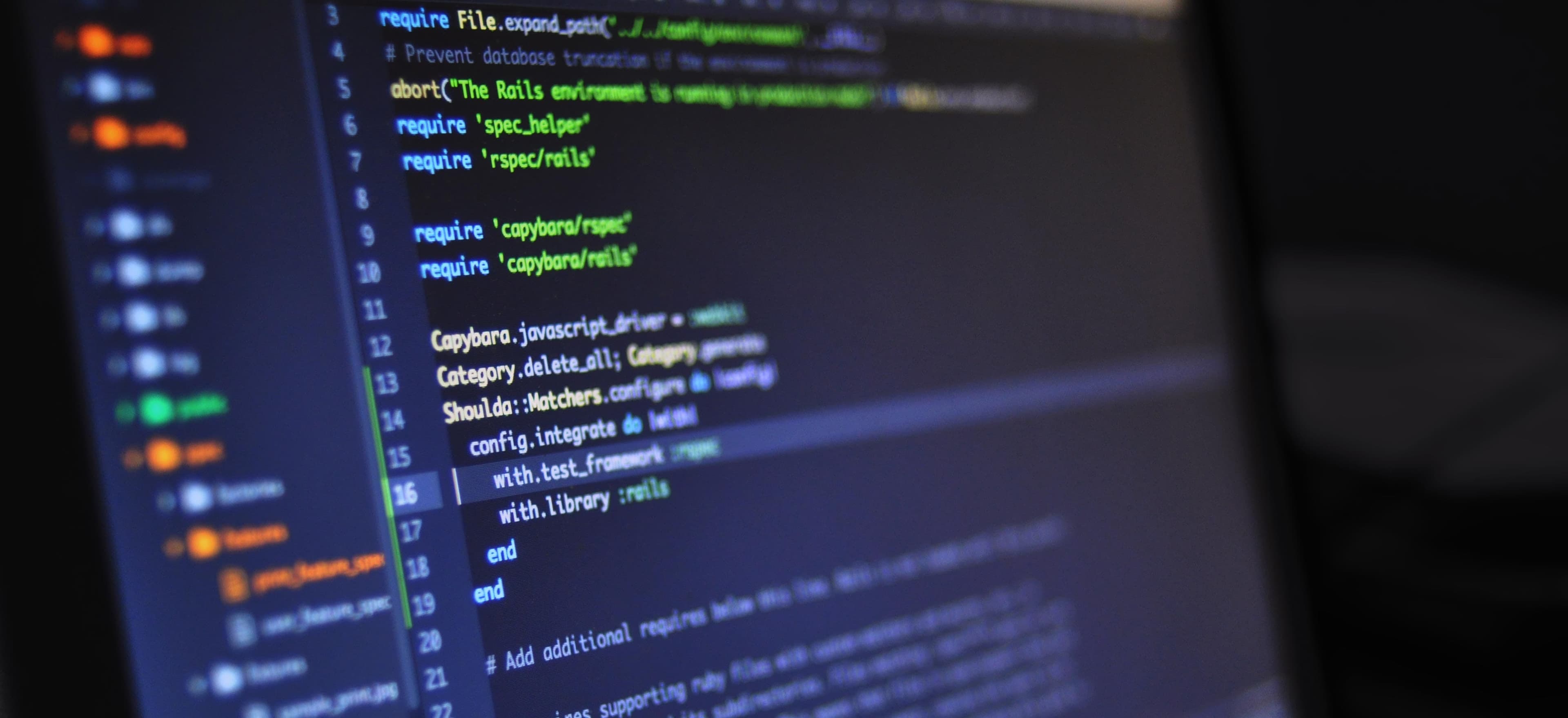
- Published on
Mastering Test-Driven Development for Better Code Quality
In today's software development landscape, maintaining code quality is crucial. Test-Driven Development (TDD) is a popular methodology that focuses on writing tests before the actual code. This approach not only ensures the code's correctness but also leads to better-designed, maintainable software. In this article, we will delve into the world of TDD using Java, exploring its benefits and best practices.
What is Test-Driven Development?
Test-Driven Development is a software development approach where tests are written before the actual code. The cycle begins with writing a test that defines a small piece of desired functionality. Once the test is in place, the developer writes the code to fulfill that test. After the code is implemented, it is then refactored to improve its structure without changing its behavior. This process is iterative, and the cycle continues for each small piece of functionality.
Benefits of Test-Driven Development
Quality Code
By writing tests before the code, TDD ensures that every piece of functionality is covered by tests. This results in a more robust and reliable codebase.
Better Design
TDD promotes a modular and loosely coupled design. Since the tests are written from a client's perspective, the code is often more decoupled and coherent.
Continuous Integration
With a comprehensive suite of tests in place, continuous integration becomes more effective. Developers can confidently integrate their code knowing that any breaking changes will be caught by the tests.
Reduced Debugging Time
TDD aims to catch defects early in the development cycle. By writing tests upfront, developers can identify and fix issues as they arise, reducing debugging time in the long run.
Getting Started with Test-Driven Development in Java
To illustrate the TDD process, let's consider a simple example of creating a basic String utility class. We will implement functionalities such as reversing a string and checking for palindromes.
Setting Up the Project
First, we will set up a Maven project in Java, which will serve as the basis for our TDD example.
public class StringUtility {
public String reverse(String str) {
// Implementation code goes here
return null;
}
public boolean isPalindrome(String str) {
// Implementation code goes here
return false;
}
}
Writing the Test Cases
Using the JUnit framework for testing, we will write test cases for the functionalities we want to implement.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class StringUtilityTest {
@Test
public void reverseString() {
StringUtility stringUtility = new StringUtility();
assertEquals("tac", stringUtility.reverse("cat"));
}
@Test
public void checkPalindrome() {
StringUtility stringUtility = new StringUtility();
assertTrue(stringUtility.isPalindrome("radar"));
assertFalse(stringUtility.isPalindrome("hello"));
}
}
Implementing the Functionality
Now that we have our test cases in place, we can proceed to write the actual functionality to make the tests pass.
public class StringUtility {
public String reverse(String str) {
return new StringBuilder(str).reverse().toString();
}
public boolean isPalindrome(String str) {
String reversed = reverse(str);
return str.equals(reversed);
}
}
Running the Tests
After implementing the functionality, we run the test cases to ensure that they pass successfully.
Best Practices in Test-Driven Development
Keep Tests Simple and Specific
Each test should focus on a specific aspect of functionality. Avoid writing complex tests that cover multiple scenarios, as they make it harder to identify the cause of a failure.
Write Production Code to Pass Tests
The code should be written with the sole purpose of making the tests pass. It should not aim to implement additional functionality beyond what the test specifies.
Refactor with Confidence
Once the tests are passing, refactor the code to improve its design without changing its behavior. The existing test suite acts as a safety net, ensuring that any refactoring does not introduce regressions.
Strive for Red-Green-Refactor
Following the red-green-refactor cycle is fundamental to TDD. Start with a failing (red) test, implement the code to make it pass (green), and then refactor the code while ensuring that all tests still pass.
Bringing It All Together
Test-Driven Development is a powerful approach for writing high-quality, maintainable code. By focusing on writing tests first, developers can streamline the development process while building a comprehensive suite of tests that act as a safety net for future changes. In the Java ecosystem, frameworks like JUnit, Mockito, and PowerMock provide robust support for TDD. Embracing TDD not only improves the code quality but also fosters a mindset of thoroughness and precision in software development.
Incorporating TDD into your Java projects can lead to more reliable and maintainable code, ultimately saving time and effort in the long run. Embrace TDD and witness the transformation in your code quality and development process.
For more in-depth information on Test-Driven Development in Java, refer to the official JUnit documentation.