Optimizing Communication: Observer/Observable Pattern in Ceylon
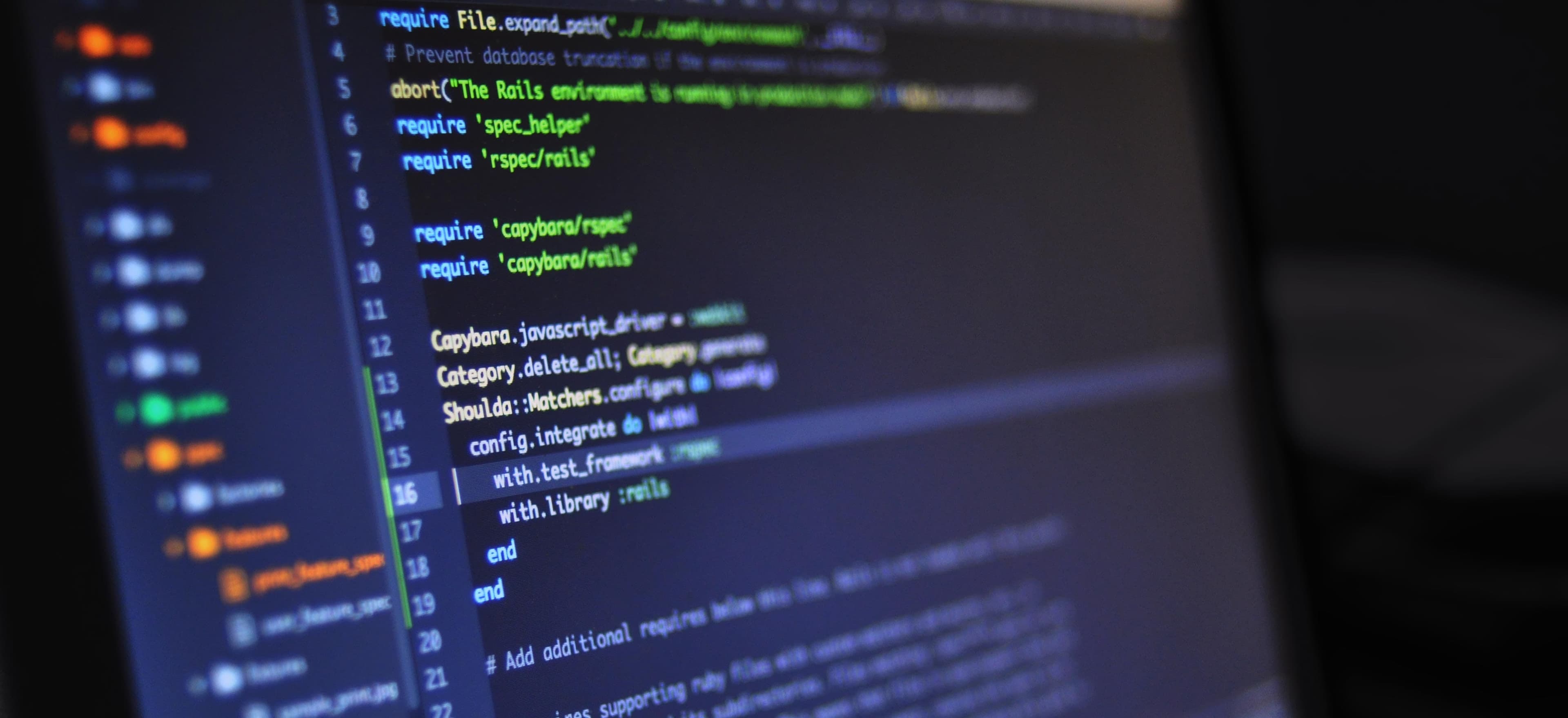
- Published on
Understanding the Observer/Observable Pattern in Ceylon
When it comes to building robust and efficient software, communication between components is crucial. One of the key principles of software design is to ensure that the different parts of a system can interact with each other in a seamless and decoupled manner. This is where design patterns come into play, offering time-tested solutions to common design challenges.
In this article, we will delve into the Observer/Observable pattern, a powerful design pattern that facilitates communication between objects in a straightforward and loosely coupled way. We will explore how this pattern is implemented in Ceylon, a modern, modular, and statically-typed programming language for the Java Virtual Machine (JVM).
Understanding the Observer/Observable Pattern
The Observer/Observable pattern is a behavioral design pattern that defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically. This pattern is particularly useful in scenarios where an object's state change should trigger specific actions in other parts of the system without making the objects tightly coupled.
Key Components of the Observer/Observable Pattern
The pattern involves two key entities:
- Observer: This is an object that wishes to be informed about the changes in the state of another object. The observer registers itself with the subject (observable) to receive updates.
- Observable (Subject): This is the object being observed. It keeps a list of its observers and notifies them of any state changes by calling their update methods.
Implementing the Observer/Observable Pattern in Ceylon
Ceylon, with its expressive syntax and strong emphasis on modularity, provides an elegant way to implement the Observer/Observable pattern. Let's dive into an example to illustrate how this pattern can be used effectively in Ceylon.
Creating the Observable
We'll start by creating the Observable
class, which will maintain a list of observers and provide methods for registering and notifying observers.
shared class Observable() {
"List of observers"
shared unordered {Observer*} observers = {};
"Register an observer"
shared void addObserver(Observer observer) {
observers.add(observer);
}
"Notify all observers"
shared void notifyObservers() {
for (obs in observers) {
obs.update();
}
}
}
In the above code, we define the Observable
class with a set of observers and methods for registering observers and notifying them of state changes. The notifyObservers
method iterates through the list of observers and calls the update
method on each observer.
Creating the Observer
Next, we'll create the Observer
interface, which defines the contract for all observers.
shared interface Observer {
"Update method called by Observable"
shared void update();
}
The Observer
interface contains a single method, update
, which will be called by the Observable
to notify the observer of any state changes.
Putting It All Together
Now, let's create a simple example to demonstrate the Observer/Observable pattern in action. We'll define a WeatherStation
class as the Observable
, and a Display
class as an Observer
. The WeatherStation
will notify the Display
whenever the weather conditions change.
shared class WeatherStation() extends Observable() {
"Weather conditions"
shared String conditions = "";
"Update weather conditions and notify observers"
shared void updateConditions(newConditions) {
conditions = newConditions;
notifyObservers();
}
}
shared class Display() satisfies Observer {
"Update method implementation"
shared actual void update() {
print("Display updated with new conditions");
}
}
In this example, the WeatherStation
class extends the Observable
class, allowing it to maintain a list of observers and notify them of any changes. The Display
class implements the Observer
interface and provides an implementation for the update
method, which in this case simply prints a message.
Why Use the Observer/Observable Pattern in Ceylon?
The Observer/Observable pattern offers several benefits when implemented in Ceylon:
- Loose Coupling: The pattern promotes loose coupling between the observable and its observers, allowing for a more maintainable and adaptable codebase.
- Scalability: The pattern facilitates the addition of new observers without requiring changes to the observable, making the system more scalable and flexible.
- Modularity: By separating concerns and enforcing a clear contract between observables and observers, the pattern enhances the modularity of Ceylon applications.
Final Considerations
In conclusion, the Observer/Observable pattern is a versatile design pattern that fosters effective communication between components while maintaining a high degree of modularity and flexibility. When implemented in Ceylon, this pattern can significantly enhance the maintainability and scalability of your applications.
By leveraging the expressive features of Ceylon, such as classes, interfaces, and strong typing, you can seamlessly implement the Observer/Observable pattern to create robust and decoupled systems. So, next time you're designing a Ceylon application with communication needs, consider incorporating the Observer/Observable pattern to streamline inter-object communication and foster a more modular architecture.
To further explore design patterns and best practices in Ceylon, check out the Ceylon official documentation and deepen your understanding of how to leverage powerful patterns to build high-quality software.
Now, go forth and design your Ceylon applications with the Observer/Observable pattern in mind, and witness the enhanced modularity and maintainability it brings to your codebase!