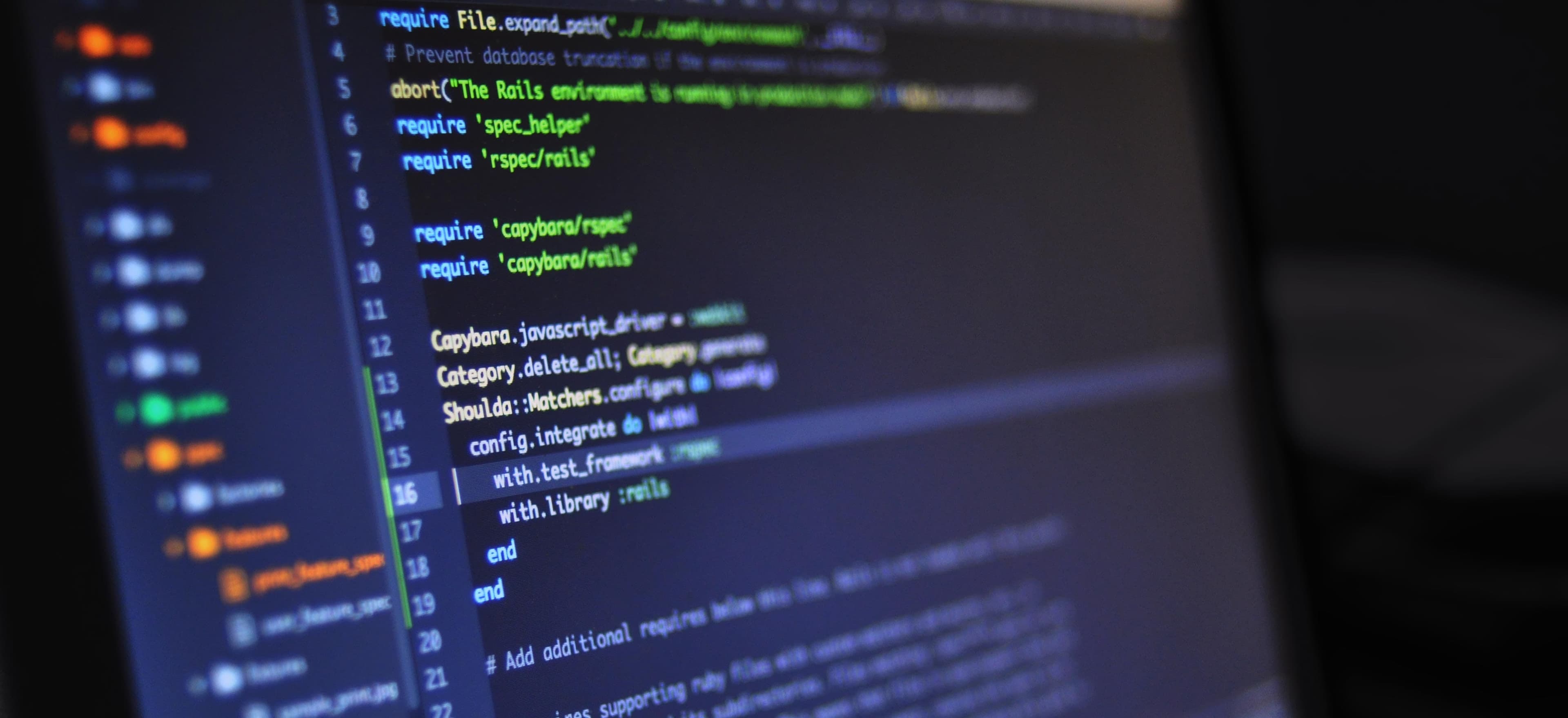
- Published on
Managing Threads in JFXPanel for Java Swing Integration
Integrating JavaFX into a Java Swing application using the JFXPanel can introduce threading complexities. To ensure a smooth and responsive user interface, it's crucial to understand how to manage threads effectively. In this post, we'll explore the best practices for managing threads in JFXPanel for seamless Java Swing integration.
Understanding the Threading Model
Before diving into thread management, it's essential to grasp the threading model of both JavaFX and Swing. JavaFX follows a single-threaded model, where all UI updates and event handling occur on the JavaFX Application Thread. On the other hand, Swing components should only be accessed and modified on the Event Dispatch Thread (EDT) to prevent concurrency issues.
Incorporating JFXPanel into Swing
When integrating JavaFX content into a Swing application, the JFXPanel serves as a bridge between the two frameworks. It allows JavaFX content to be embedded within a Swing application while ensuring that the threading requirements of both platforms are respected.
Running Tasks on the JavaFX Application Thread
Given JavaFX's single-threaded nature, any task that directly manipulates JavaFX components must be executed on the JavaFX Application Thread. To achieve this within a JFXPanel, the Platform.runLater()
method can be utilized to post a Runnable to the JavaFX Application Thread's queue.
Platform.runLater(() -> {
// Update JavaFX components here
});
By using Platform.runLater()
, tasks can safely interact with JavaFX components without causing threading conflicts.
Handling Long-Running Tasks
When dealing with long-running tasks within a JFXPanel, it's crucial to offload these operations to background threads to prevent UI unresponsiveness. Java provides various concurrency utilities, such as ExecutorService
, to manage background tasks effectively.
ExecutorService executor = Executors.newSingleThreadExecutor();
executor.submit(() -> {
// Perform time-consuming task
Platform.runLater(() -> {
// Update JavaFX components with results
});
});
In the above snippet, the time-consuming task is executed on a separate thread via an ExecutorService
, while the UI update is delegated to the JavaFX Application Thread using Platform.runLater()
.
Synchronizing Data Between JavaFX and Swing
In a Java Swing application that incorporates JavaFX via JFXPanel, it's common to encounter scenarios where data needs to be shared between the two frameworks. To ensure data consistency and thread safety, proper synchronization mechanisms must be employed.
One approach is to leverage javafx.application.Platform.runLater()
for invoking JavaFX-related operations, while Swing-related operations can be executed on the Event Dispatch Thread using SwingUtilities.invokeLater()
.
// From the Swing thread
SwingUtilities.invokeLater(() -> {
// Access Swing components
});
// From the JavaFX thread
Platform.runLater(() -> {
// Access JavaFX components
});
By adhering to these threading practices, data synchronization between JavaFX and Swing can be achieved reliably.
Managing Resource Cleanup
In any application involving concurrent programming, resource cleanup is a critical aspect to prevent memory leaks and resource exhaustion. Within the context of JFXPanel, it's essential to ensure proper cleanup of resources such as threads and event listeners when they are no longer needed.
Using try-with-resources is a convenient way to manage resources in Java, ensuring that they are properly closed or released after being used.
try (ExecutorService executor = Executors.newSingleThreadExecutor()) {
executor.submit(() -> {
// Perform task
Platform.runLater(() -> {
// Update JavaFX components with results
});
});
}
By encapsulating the ExecutorService within a try-with-resources block, the executor will be automatically closed when it goes out of scope, leading to proper resource cleanup.
Final Considerations
Incorporating JavaFX content into a Java Swing application using JFXPanel can lead to a more dynamic and visually appealing user interface. However, it's vital to understand and adhere to the threading requirements of both frameworks to maintain a responsive and stable application.
By following the best practices outlined in this post, you can effectively manage threads within JFXPanel, ensuring seamless integration between JavaFX and Swing while maintaining a smooth user experience.
To further enhance your understanding of threading in Java, consider exploring the intricacies of Java multithreading and the principles of concurrency.