Troubleshooting Common Errors in Play Framework
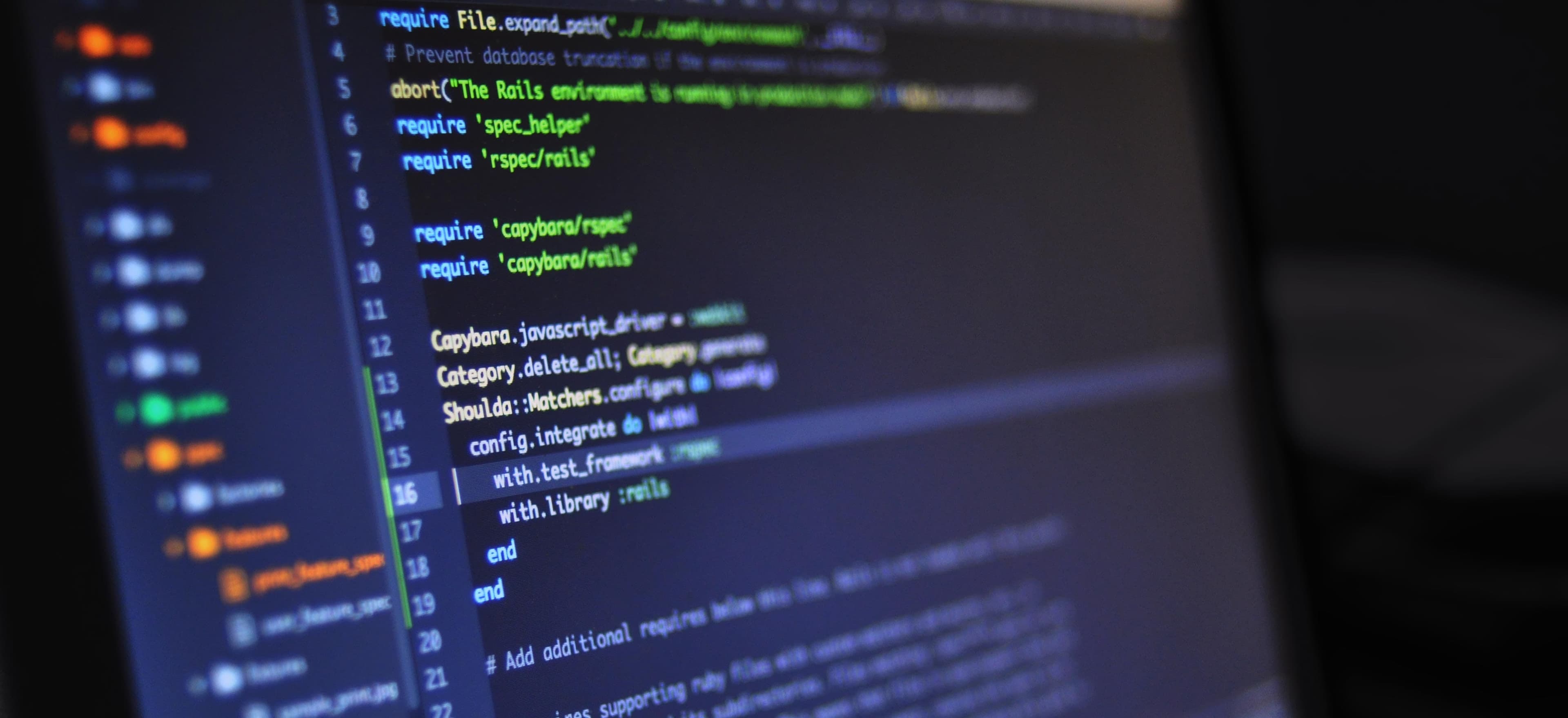
- Published on
Troubleshooting Common Errors in Play Framework
Play Framework is a powerful web application framework for Java developers, offering a seamless development experience. However, like any other framework, developers often encounter errors that can be frustrating to resolve. In this guide, we will discuss some common errors in Play Framework and their solutions.
1. Compilation Errors
Description
Compilation errors can occur due to various reasons such as syntax errors, missing dependencies, or incorrect configurations.
Solution
Check the logs for detailed error messages, resolve any syntax errors in the code, and ensure that all dependencies are correctly specified in the build.sbt
file. If the error persists, try running sbt clean
to remove any cached files that might be causing conflicts.
2. Configuration Errors
Description
Play Framework relies heavily on configuration files, and errors in these files can lead to runtime issues.
Solution
Carefully review the configuration files such as application.conf
and routes
file. Ensure that all configurations are properly formatted and referenced. Utilize the Typesafe Config library for managing configurations in a more structured manner.
3. Database Connection Issues
Description
Problems related to database connections often arise when the application is unable to establish a connection with the database.
Solution
Double-check the database configurations in the application.conf
file to ensure that the connection parameters are accurate. Also, verify that the database service is running and accessible from the application's environment.
4. Template Compilation Errors
Description
Template compilation errors occur when there are issues with the Twirl templates, such as syntax errors or incorrect usage of template tags.
Solution
Carefully review the template files and fix any syntax errors. Ensure that the template tags are used correctly, and all variables are properly passed from the controllers.
5. Dependency Management
Description
Managing dependencies in a Play Framework project is crucial, and errors related to dependency resolution can hinder the application's functionality.
Solution
Use the sbt
tool to manage dependencies and their versions. Always ensure that the specified versions are compatible with each other to avoid conflicts. Consider using the dependencyUpdates
task to check for any outdated dependencies.
6. Handling Asynchronous Operations
Description
Play Framework promotes non-blocking, asynchronous operations, and handling them incorrectly can lead to issues such as race conditions or deadlocks.
Solution
When writing asynchronous code, utilize the built-in features of Play Framework such as CompletionStage
and play.libs.concurrent.Futures
to manage asynchronous computations effectively. Avoid blocking operations within asynchronous code to prevent performance bottlenecks.
Code Sample: Asynchronous Action in Play Framework
public CompletionStage<Result> index() {
return CompletableFuture.supplyAsync(() -> {
// Perform asynchronous operations here
return "Hello, World!";
}).thenApplyAsync(result -> {
return ok(result);
}, httpExecutionContext.current());
}
In the above code, we define an asynchronous action using CompletionStage
to execute non-blocking operations.
7. Cross-Origin Resource Sharing (CORS) Issues
Description
When building API endpoints, CORS issues often arise due to restrictions on cross-origin requests.
Solution
Integrate the CORS filter provided by Play Framework to handle cross-origin requests. Configure the filter to allow requests from specific origins while adhering to security best practices.
A Final Look
While working with Play Framework, encountering errors is inevitable, but with a solid understanding of the common pitfalls and their solutions, developers can effectively troubleshoot and resolve issues. By familiarizing yourself with the intricacies of Play Framework and employing best practices, you can build robust and resilient applications.
Remember, when faced with errors, patience and systematic debugging always prevail.
For further reading and in-depth learning, consider referring to the official Play Framework documentation and the Play Framework GitHub repository.