Solving Dependency Management Issues in Scala with SBT
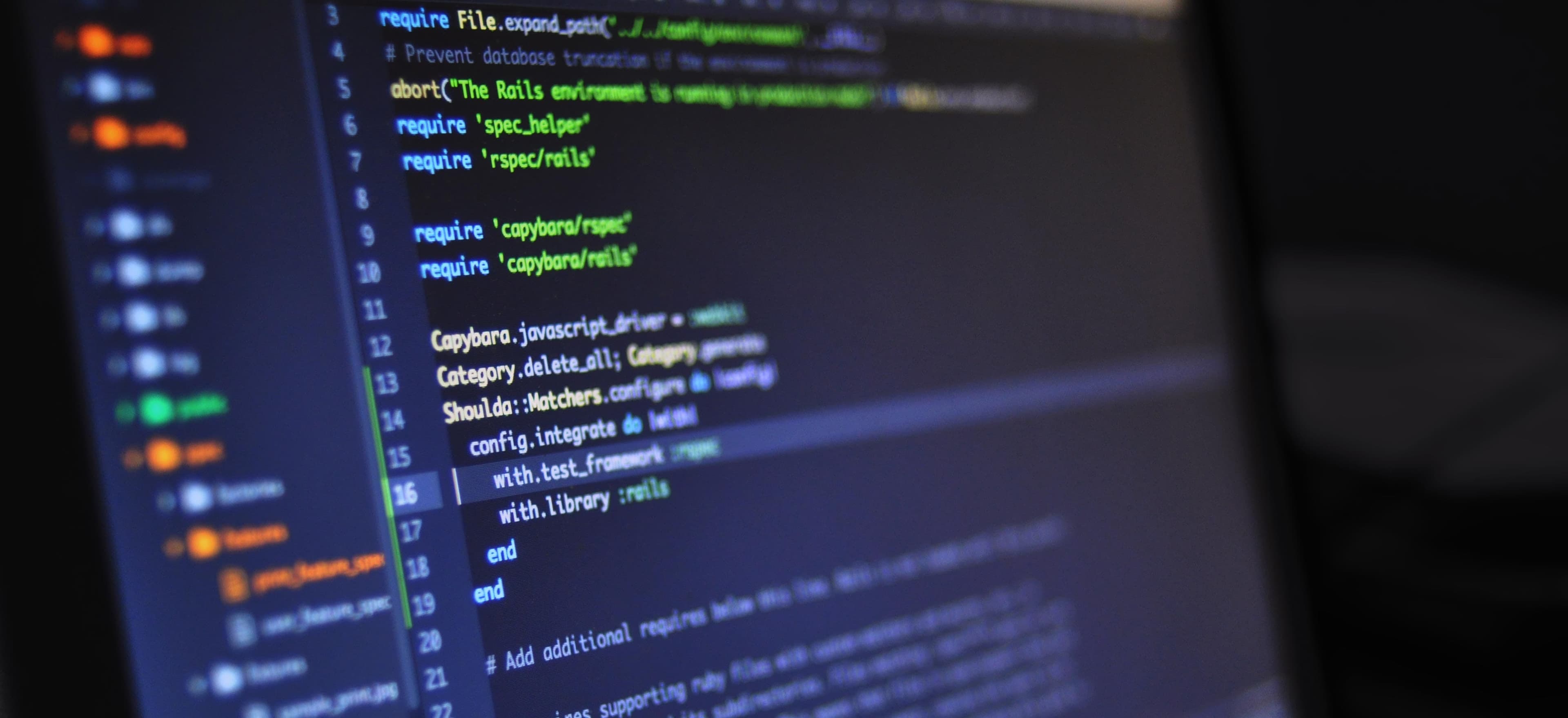
- Published on
Solving Dependency Management Issues in Scala with SBT
In the world of Scala, dependency management is a crucial aspect of software development. As developers, we often find ourselves struggling with issues related to dependency conflicts, incompatible versions, and missing libraries.
This is where SBT (Simple Build Tool) comes to the rescue. SBT is a popular build tool for Scala and Java projects, known for its powerful dependency management capabilities. In this blog post, we will explore how SBT can help us solve dependency management issues in Scala.
Understanding Dependency Management
Before diving into SBT, let's first understand the basics of dependency management. When building a Scala project, we often rely on third-party libraries and frameworks to accomplish various tasks. These dependencies are specified in a build file (such as build.sbt
) using a specific syntax.
When we add dependencies to our project, they may in turn have their own dependencies. This creates a dependency tree, where each library depends on other libraries, and so on. Sometimes, conflicts can arise when different libraries require different versions of the same dependency, or when incompatible versions are pulled in.
Introducing SBT
SBT uses an innovative approach to handle dependency management, by leveraging the Ivy library for resolving dependencies. Ivy is a powerful transitive dependency manager, which means it can automatically resolve and download dependencies needed by a particular library.
Additionally, SBT uses a concept called "managed dependencies", where it automatically fetches and caches library dependencies. This ensures that a consistent set of dependencies is used across all developers working on the same project.
Declaring Dependencies in SBT
In SBT, dependencies are declared in the build.sbt
file using the libraryDependencies
setting. Each dependency is specified using the "groupId" % "artifactId" % "version"
syntax, where groupId
refers to the organization or group that owns the library, artifactId
is the name of the library, and version
specifies the desired version.
libraryDependencies += "org.apache.spark" %% "spark-core" % "3.1.2"
In this example, we are adding a dependency on Apache Spark's core library with version 3.1.2 to our project.
Resolving Dependency Conflicts
One common issue in dependency management is resolving conflicts between different versions of the same library. This can happen when two dependencies indirectly pull in different versions of a transitive dependency.
SBT provides an elegant solution to this problem by using the dependencyOverrides
setting. By specifying dependencyOverrides
, we can force SBT to use a specific version of a dependency, regardless of what other libraries require.
dependencyOverrides += "org.slf4j" % "slf4j-api" % "1.7.32"
In this example, we are overriding any conflicting versions of the slf4j-api
library and forcing SBT to use version 1.7.32.
Managing Exclusions
Sometimes, we may want to exclude certain transitive dependencies that are pulled in by a library. This is where the exclude
method comes in handy. We can use the exclude
method to exclude specific transitive dependencies based on their groupId
and artifactId
.
libraryDependencies += "org.apache.kafka" % "kafka-clients" % "3.0.0" exclude("org.slf4j", "slf4j-log4j12")
In this example, we are excluding the slf4j-log4j12
dependency pulled in by the Kafka clients library.
Handling Binary Incompatibility
Another common challenge in dependency management is dealing with binary incompatibility between different versions of a library. SBT provides a solution to this problem through the use of the %
operator in dependency declarations.
When specifying a dependency, we can use %%
instead of %
to automatically append the Scala version used in our project. This is particularly useful when working with Scala libraries, as it ensures that the correct version of a library compiled for our Scala version is used.
libraryDependencies += "org.scalaz" %% "scalaz-core" % "7.3.1"
In this example, the %%
operator appends the Scala version to the artifact name, ensuring that the correct version of scalaz-core
for our Scala version is used.
Wrapping Up
In conclusion, SBT provides a robust and intuitive solution to the challenges of dependency management in Scala. By leveraging its powerful features such as managed dependencies, conflict resolution, exclusion management, and binary compatibility, we can effectively overcome dependency-related issues and build reliable and maintainable Scala projects.
SBT's flexibility and ease of use make it an indispensable tool for Scala developers, enabling them to focus on writing code instead of dealing with dependency headaches.
By mastering SBT's dependency management capabilities, you can streamline your Scala projects and unleash your full potential as a developer.
So go ahead, embrace SBT, and take your Scala development to the next level!
Remember, mastering SBT takes time and practice, but it's well worth the effort in the long run.
Happy coding!
Additional resources: