Dealing with Bug Fatigue
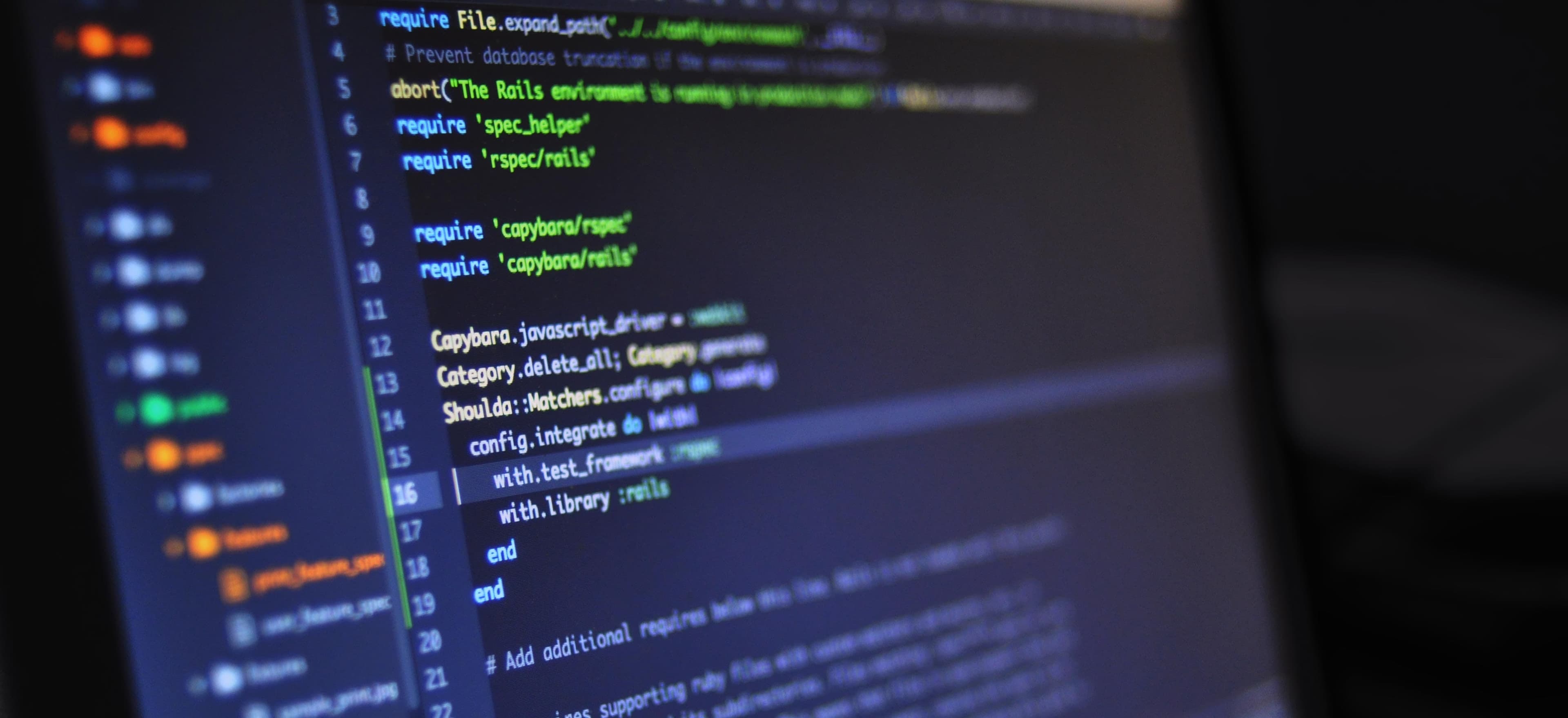
- Published on
The Struggle with Bug Fatigue: A Java Developer's Guide
As a Java developer, you're familiar with the struggle of dealing with bugs. It seems like no matter how meticulous you are with your code, bugs always find a way to creep in and wreak havoc. The constant battle with bugs can be draining, leading to what is known as "bug fatigue."
Understanding Bug Fatigue
Bug fatigue is the mental and emotional exhaustion that developers experience when dealing with a high volume of bugs over an extended period. It can lead to decreased productivity, frustration, and burnout. Fortunately, there are strategies that Java developers can employ to combat bug fatigue and regain control of their development process.
Writing Clean and Maintainable Code
One of the most effective ways to prevent bugs is by writing clean and maintainable code. When you adhere to best practices such as following naming conventions, writing modular code, and incorporating design patterns, you reduce the likelihood of introducing bugs. For example, consider the following Java code snippet:
// Bad practice
public class Example {
public void doSomething(int x, int y) {
// method implementation
}
}
// Good practice
public class Example {
public void calculateSum(int operand1, int operand2) {
// method implementation
}
}
In the above example, the use of self-explanatory method names and descriptive variable names enhances code readability, making it easier to spot and fix errors.
Automated Testing
Automated testing is a crucial aspect of the software development process. Writing unit tests using frameworks like JUnit not only helps catch bugs early but also serves as living documentation for your codebase. By incorporating test-driven development (TDD) practices, you can write tests before implementing the actual code, leading to a more robust and bug-resistant codebase.
@Test
public void testCalculateSum() {
Example example = new Example();
int result = example.calculateSum(3, 5);
assertEquals(8, result);
}
The test above validates the calculateSum
method, ensuring its correctness. By automating these tests, you can catch regressions and bugs before they manifest into larger issues.
Code Reviews
Engaging in peer code reviews is an effective way to catch bugs before they make their way into the production codebase. By having a fresh pair of eyes scrutinize your code, you can identify potential issues or bugs that you may have overlooked.
Utilizing Static Code Analysis Tools
Leverage static code analysis tools like FindBugs, PMD, and Checkstyle to automatically analyze your Java code for potential bugs, code style violations, and performance inefficiencies. Integrating these tools into your continuous integration pipeline allows for the automatic detection and prevention of common coding errors.
Embracing Continuous Integration and Continuous Deployment (CI/CD)
Implementing CI/CD practices not only streamlines the deployment process but also helps in catching bugs early through automated builds and testing. Tools like Jenkins, Travis CI, and GitLab CI/CD enable you to automate the entire software delivery process, reducing the likelihood of introducing bugs during manual deployments.
Leveraging Java Debugging Tools
When bugs do inevitably make their way into your codebase, efficient debugging becomes paramount. Utilize powerful Java debugging tools such as Eclipse Debugger, IntelliJ IDEA Debugger, or VisualVM to trace and analyze the root cause of bugs in your applications. These tools offer features like breakpoints, variable inspection, and stack trace analysis, aiding in the quick identification and resolution of bugs.
To Wrap Things Up
In the world of Java development, bugs are an inevitable part of the process. However, by adopting best practices such as writing clean code, automated testing, code reviews, static analysis, CI/CD, and efficient debugging, you can significantly reduce the occurrence of bugs and mitigate bug fatigue. Remember, a proactive approach to bug prevention is key to maintaining your sanity as a Java developer.
References:
- JUnit
- FindBugs
- PMD
- Checkstyle
- Jenkins
- Travis CI
- GitLab CI/CD
- Eclipse Debugger
- IntelliJ IDEA Debugger
- VisualVM
Bug fatigue may seem inevitable, but it doesn't have to consume your development efforts. With the right approaches and tools in place, you can minimize bugs and maintain your productivity and passion for Java development.