Common Mistakes to Avoid in Spring Environment Initialization
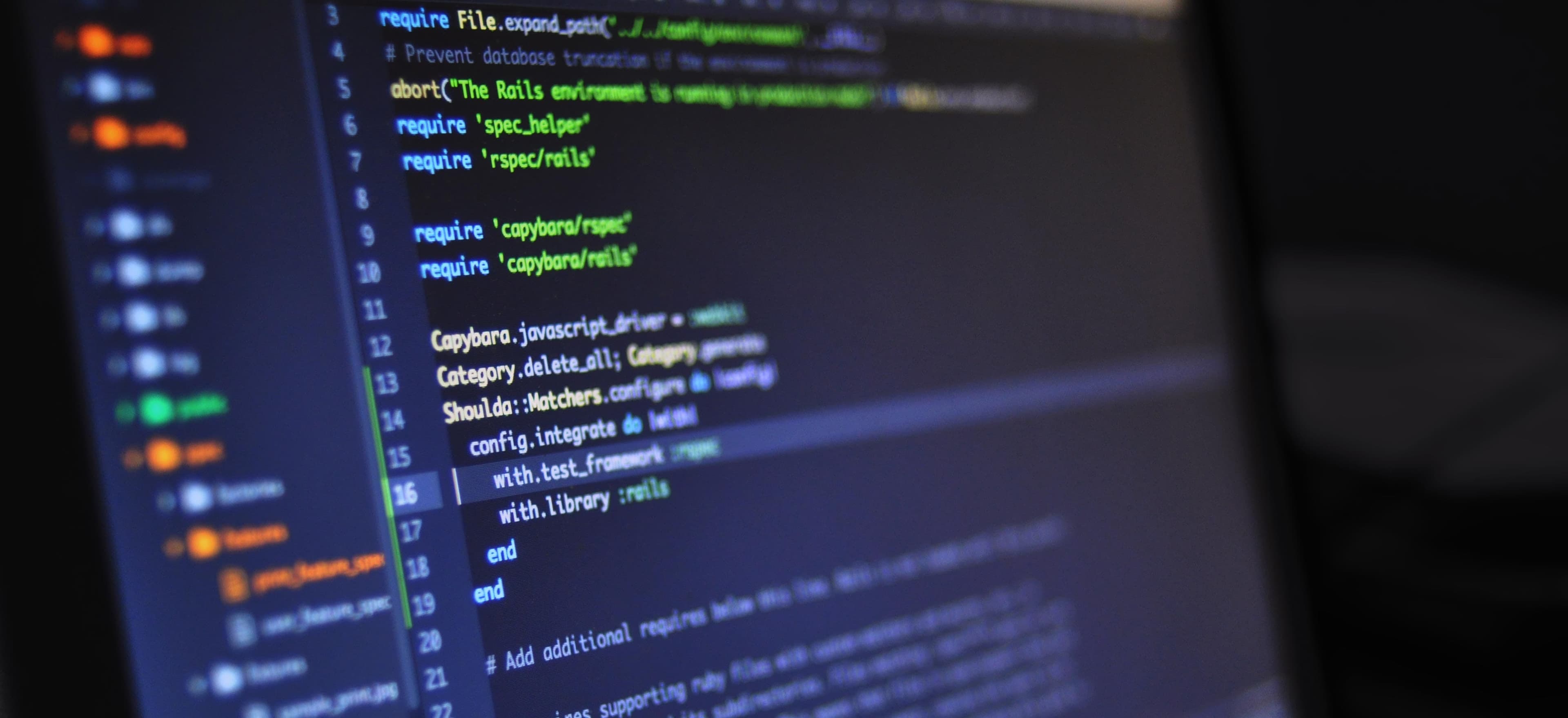
- Published on
Common Mistakes to Avoid in Spring Environment Initialization
When working with the Spring framework in Java, environment initialization plays a crucial role in ensuring the smooth functioning of the application. However, there are several common mistakes that developers make during this phase that can lead to issues such as performance bottlenecks, security vulnerabilities, and configuration problems.
In this article, we will explore some of the most common mistakes to avoid when initializing the Spring environment, and provide best practices to help you mitigate these issues.
Mistake 1: Not Using Spring Profiles Effectively
One common mistake in Spring environment initialization is not utilizing Spring profiles effectively. Spring profiles allow you to segregate beans, properties, and configurations for different environments, such as development, testing, and production. Failing to use profiles effectively can result in misconfigured settings being applied to the wrong environment, leading to runtime errors or unexpected behavior.
Solution:
Use Spring profiles to define environment-specific configurations, such as database connection settings, logging levels, and external service endpoints. By utilizing profiles, you can ensure that the correct configuration is applied to each environment, reducing the risk of misconfiguration.
@Configuration
@Profile("dev")
public class DevelopmentConfig {
// Development-specific beans and configurations
}
@Configuration
@Profile("prod")
public class ProductionConfig {
// Production-specific beans and configurations
}
Mistake 2: Hard-coding Sensitive Information
Another common mistake is hard-coding sensitive information, such as database credentials, API keys, or security tokens, directly in the configuration files or source code. Hard-coding sensitive information can lead to security vulnerabilities and make it difficult to manage and update these values without modifying the codebase.
Solution:
Use property files, environment variables, or secret management tools to externalize and securely store sensitive information. Spring provides support for property placeholders and property file encryption to manage sensitive properties securely.
// application.properties
database.url=${database.url}
database.username=${database.username}
database.password=${database.password}
By using property placeholders, you can externalize sensitive information and provide environment-specific values without hard-coding them in the configuration files.
Mistake 3: Overlooking Bean Lifecycle Management
Improper management of bean lifecycles can lead to memory leaks, resource contention, and performance degradation. Failing to release resources or clean up dependencies when beans are destroyed can have a significant impact on the overall health and performance of the application.
Solution:
Utilize the @PreDestroy
and @PostConstruct
annotations to manage bean initialization and destruction. By using these lifecycle callbacks, you can ensure that resources are properly initialized and released when the bean is created and destroyed.
@Component
public class MyResourceHandler {
@PostConstruct
public void initialize() {
// Initialize and acquire resources
}
@PreDestroy
public void releaseResources() {
// Release and clean up resources
}
}
By properly managing bean lifecycles, you can prevent memory leaks and ensure that resources are released when they are no longer needed.
Mistake 4: Ignoring Dependency Injection Best Practices
Inadequate use of dependency injection can lead to tightly coupled and difficult-to-test components, violating the principles of SOLID design and making the codebase less maintainable.
Solution:
Adhere to dependency injection best practices, such as constructor injection or setter injection, to decouple components and improve testability. By leveraging dependency injection, you can easily swap dependencies, mock objects for testing, and improve the overall modularity of the codebase.
public class MyService {
private final MyRepository repository;
public MyService(MyRepository repository) {
this.repository = repository;
}
// Service methods using MyRepository
}
By using constructor injection, you can clearly define and inject dependencies, promoting a more modular and testable codebase.
Mistake 5: Neglecting Environment-Specific Configurations
Failing to account for environment-specific configurations, such as thread pool sizes, cache settings, and connection timeouts, can lead to suboptimal performance and compatibility issues across different environments.
Solution:
Create environment-specific configuration files or leverage conditional annotations to tailor configurations based on the target environment. By providing specific configurations for each environment, you can optimize the application's performance and ensure compatibility across different deployment environments.
@Configuration
@Profile("prod")
public class ProductionConfig {
@Bean
public ThreadPoolTaskExecutor taskExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(20);
return executor;
}
}
By defining environment-specific configurations, you can fine-tune the application's settings to match the requirements of each deployment environment.
In Conclusion, Here is What Matters
In conclusion, avoiding common mistakes in Spring environment initialization is essential for building robust and maintainable applications. By effectively utilizing Spring profiles, externalizing sensitive information, managing bean lifecycles, following dependency injection best practices, and accounting for environment-specific configurations, you can ensure the smooth functioning of your Spring-based applications.
By applying these best practices, you can mitigate potential issues related to misconfigurations, security vulnerabilities, and performance bottlenecks, ultimately delivering a more reliable and efficient software solution.
Remember, taking the time to establish proper environment initialization practices will pay off in the long run by reducing technical debt and ensuring the scalability and stability of your Spring applications.
To delve deeper into Spring environment initialization and best practices, refer to the official Spring Documentation.
Now, armed with the knowledge of these common mistakes and their solutions, you can elevate your Spring environment initialization practices and build more resilient and secure applications.