Developing Scalable Web Applications with Play Framework
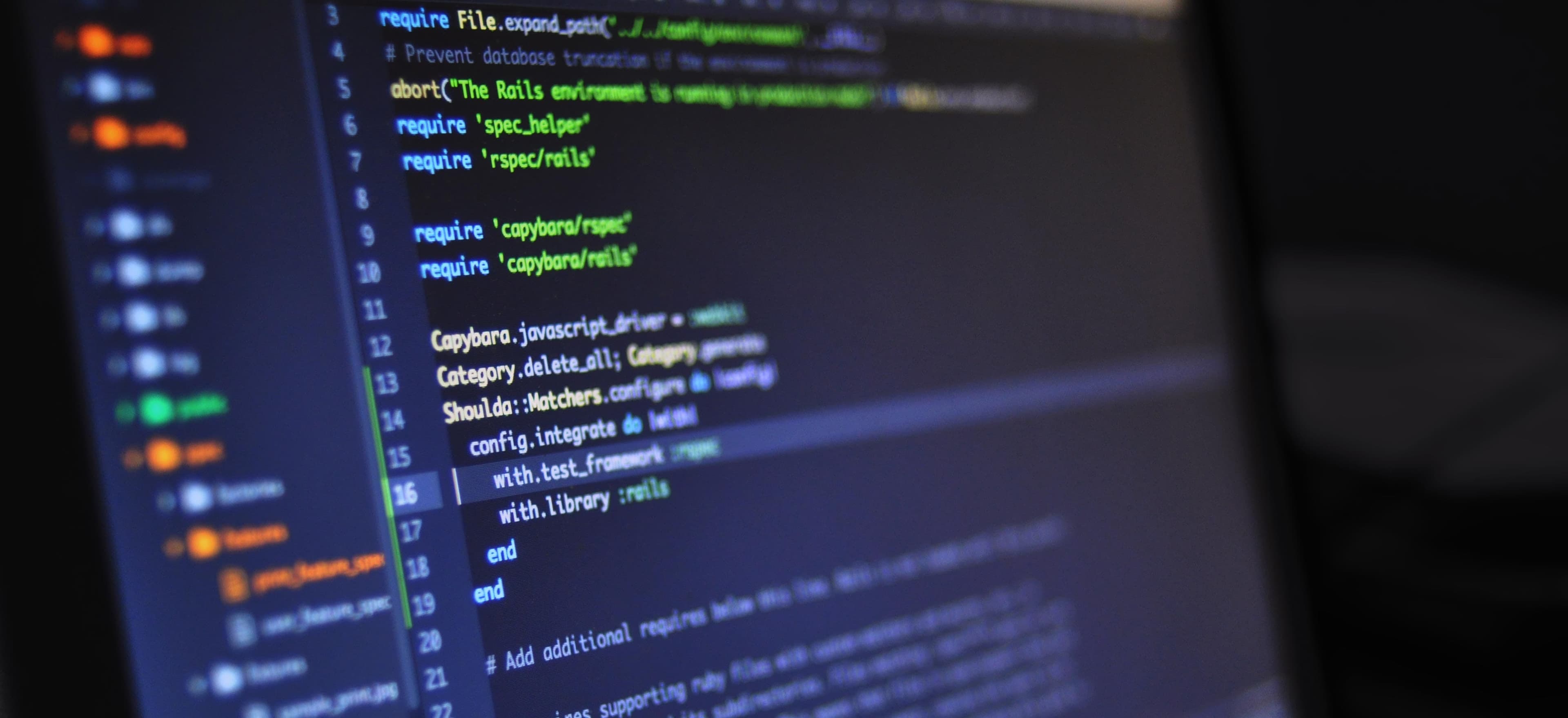
- Published on
Developing Scalable Web Applications with Play Framework
When it comes to developing web applications, scalability is a crucial factor. To meet the demands of growing user bases, it's essential to choose the right framework. Play Framework, with its asynchronous, non-blocking I/O model, is an excellent choice for building scalable web applications in Java. In this article, we will explore how Play Framework enables developers to create highly scalable web applications and discuss best practices for achieving scalability.
What is Play Framework?
Play Framework is a modern web framework for building web applications with Java and Scala. It follows the principles of reactive programming, emphasizing high performance and scalability. Play Framework's built-in support for asynchronous I/O and its lightweight, stateless architecture make it well-suited for building scalable applications.
Asynchronous and Non-Blocking I/O
One of the key features that makes Play Framework highly scalable is its support for asynchronous and non-blocking I/O. Traditional web frameworks typically use a synchronous model where each request is handled by a separate thread, potentially leading to thread exhaustion under heavy load. In contrast, Play Framework's asynchronous model allows a small number of threads to handle a large number of connections, making more efficient use of system resources.
Let's take a look at an example of how Play Framework handles asynchronous operations:
public class MyController extends Controller {
public CompletionStage<Result> index() {
return CompletableFuture.supplyAsync(() -> {
// Perform asynchronous operation
return "Hello, World!";
}).thenApplyAsync(result -> ok(result), httpExecutionContext.current());
}
}
In this example, the index
method returns a CompletionStage
which represents a potentially asynchronous computation. Inside the supplyAsync
method, we perform the asynchronous operation, and then use the thenApplyAsync
method to apply the result to the ok
function, which generates the HTTP response. This asynchronous and non-blocking approach allows the server to handle a large number of concurrent requests without requiring a thread per request.
Statelessness and Horizontal Scalability
Play Framework promotes a stateless architecture, where each request is handled independently without relying on server-side session state. This makes it easier to achieve horizontal scalability by adding more server instances, as requests can be distributed across multiple servers without the need for shared state. Additionally, the stateless nature of Play Framework simplifies the management of server instances in a cluster, as any server can handle any request without relying on affinity to specific clients.
Reactive Streams and Back-Pressure
Play Framework leverages Reactive Streams to handle asynchronous streams of data with back-pressure. This allows applications to react to varying demand and avoid overwhelming resources by applying back-pressure to slow down the data source when the data consumer is unable to keep up. Reactive Streams enable Play Framework applications to handle streaming data in a scalable and efficient manner, making it suitable for building real-time web applications and APIs.
Let's see how Play Framework uses Reactive Streams to handle data streaming:
public Result streamData() {
Source<Integer, NotUsed> dataSource = Source.range(1, 1000);
Source<ByteString, NotUsed> dataStream = dataSource.map(i -> ByteString.fromString(i.toString()));
return ok().chunked(dataStream);
}
In this example, we create a data source using Source.range
and transform it into a stream of ByteString
using the map
operator. We then return the data stream as a chunked HTTP response. This approach allows Play Framework to efficiently handle streaming data with back-pressure, ensuring that the data source does not overwhelm the client.
Best Practices for Scalability
While Play Framework provides powerful features for building scalable web applications, developers should also follow best practices to fully leverage its capabilities. Some best practices for achieving scalability with Play Framework include:
Use Asynchronous APIs
Whenever possible, use asynchronous APIs for I/O operations to avoid blocking threads and make efficient use of system resources. Play Framework provides built-in support for asynchronous programming, allowing developers to easily write non-blocking code.
Embrace Reactive Patterns
Utilize reactive programming patterns to handle asynchronous and streaming data in a scalable manner. Reactive Streams, as supported by Play Framework, provide a standardized way to handle asynchronous streams with back-pressure, ensuring efficient resource utilization.
Horizontal Scaling
Design applications with a stateless and distributed architecture to facilitate horizontal scaling. By avoiding server-side state and relying on independent request handling, Play Framework applications can easily scale horizontally by adding more server instances.
Performance Testing and Optimization
Regularly perform performance testing and optimization to identify bottlenecks and improve the overall scalability of the application. Play Framework provides tools for profiling and monitoring application performance, allowing developers to optimize critical components for scalability.
Bringing It All Together
In conclusion, Play Framework is an excellent choice for developing highly scalable web applications in Java. Its support for asynchronous and non-blocking I/O, stateless architecture, and integration with Reactive Streams make it well-suited for handling the demands of scalable web applications. By following best practices and leveraging the features of Play Framework, developers can build web applications that are capable of handling large numbers of concurrent users while maintaining high performance and responsiveness.