Optimizing Java Performance with GraalVM Community Edition
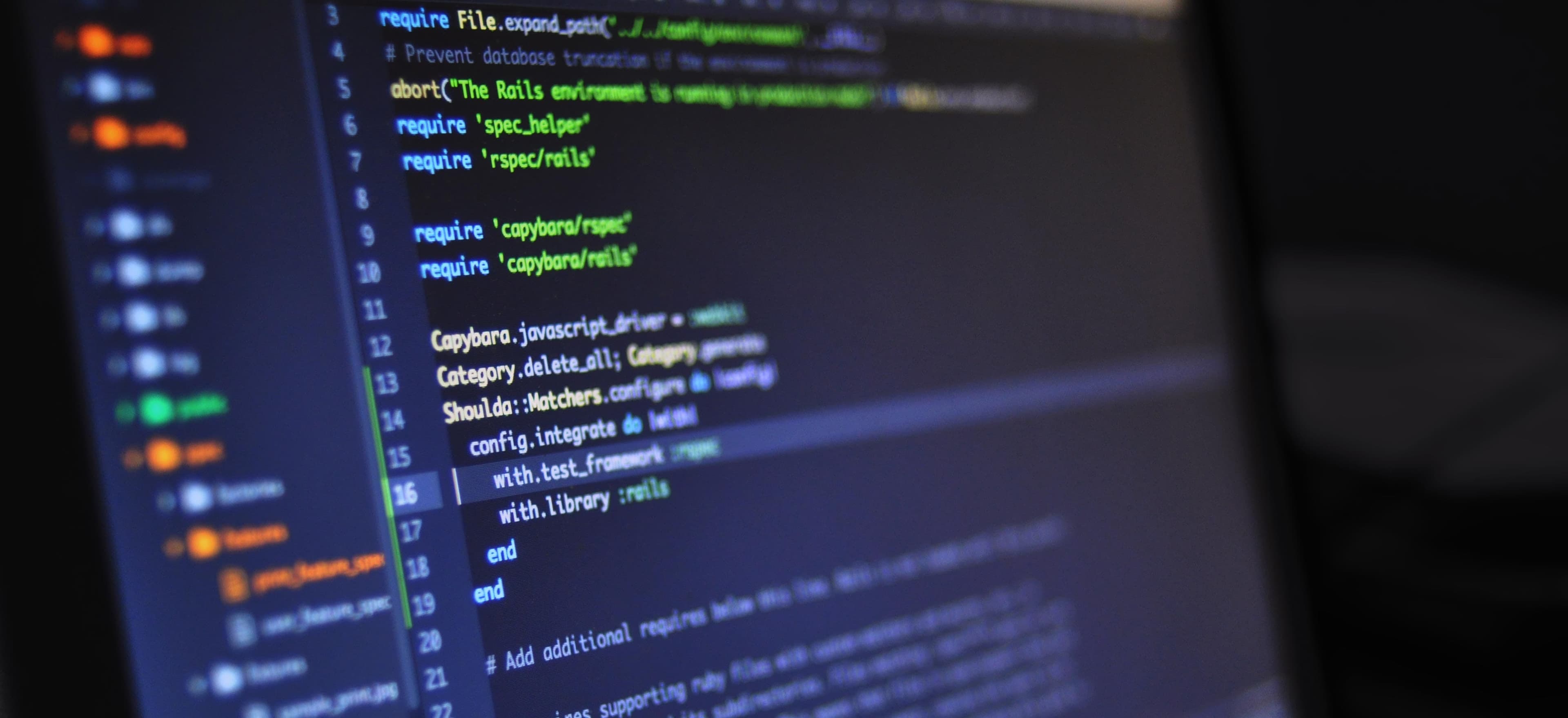
- Published on
Optimizing Java Performance with GraalVM Community Edition
GraalVM is a high-performance virtual machine that offers a polyglot runtime environment for running applications written in multiple languages, including Java, JavaScript, Python, and more. In this article, we will focus on leveraging GraalVM Community Edition to optimize the performance of Java applications. We'll explore the key features of GraalVM and how it can be used to boost the performance of Java applications, including ahead-of-time (AOT) compilation and just-in-time (JIT) compilation, and how it enables efficient interoperability between different languages.
What is GraalVM?
GraalVM is a universal virtual machine and a language runtime designed to support various programming languages and execution modes. It enables developers to run applications written in different languages on a single virtual machine. GraalVM provides high-performance execution for standalone Java applications, microservices, and polyglot applications.
Some key components and features of GraalVM Community Edition include:
-
GraalVM Compiler: The GraalVM compiler offers highly optimized JIT compilation, enabling improved runtime performance for Java applications.
-
GraalVM Substrate VM: Substrate VM supports AOT compilation, which allows Java applications to be ahead-of-time compiled into native executables, enhancing startup time and reducing memory overhead.
-
Polyglot Runtimes: GraalVM supports polyglot programming, allowing developers to write applications using multiple languages. This enables seamless integration of different language components within a single application.
-
Native Image: GraalVM's Native Image tool allows the AOT compilation of Java applications, resulting in standalone native executables that start up quickly and have reduced memory footprint.
Enhancing Java Performance with GraalVM
JIT Compilation with GraalVM
GraalVM's JIT compiler is one of its key components, providing highly optimized dynamic compilation for Java applications. By leveraging advanced optimization techniques and runtime profiling, GraalVM's JIT compiler can significantly improve the performance of Java applications during runtime.
Let's take a look at an example of how GraalVM's JIT compilation can enhance the performance of a Java application. Consider the following snippet of Java code:
public class Main {
public static void main(String[] args) {
long startTime = System.nanoTime();
for (int i = 0; i < 100_000_000; i++) {
// Perform some computation
}
long endTime = System.nanoTime();
System.out.println("Elapsed time: " + (endTime - startTime) / 1_000_000 + "ms");
}
}
In this example, the for
loop performs a computation 100 million times, and we measure the elapsed time using System.nanoTime()
. When running this code on GraalVM with JIT compilation enabled, the JIT compiler can optimize the loop and the computation, resulting in improved performance compared to running the same code on a traditional JVM without GraalVM.
AOT Compilation with GraalVM Substrate VM
In addition to JIT compilation, GraalVM's Substrate VM provides ahead-of-time compilation capabilities for Java applications. AOT compilation improves startup time and reduces the memory overhead of Java applications by directly generating native executables from Java bytecode.
Here's an example of using GraalVM's Native Image tool to perform AOT compilation:
-
Install GraalVM Community Edition: GraalVM Installation Guide.
-
Build the Java application JAR file:
javac Main.java
jar cf Main.jar Main.class
- Use the Native Image tool to perform AOT compilation:
native-image Main
The native-image
command compiles the Java application Main
into a native executable. Running the resulting native executable bypasses the JVM startup overhead, resulting in faster startup times and reduced memory usage.
Polyglot Programming with GraalVM
GraalVM's polyglot runtime environment facilitates seamless integration of multiple languages within a single application. This enables developers to leverage the strengths of different languages and libraries while building complex applications.
For example, a Java application can call JavaScript functions directly using GraalVM's polyglot capabilities. This allows Java developers to incorporate existing JavaScript libraries or code snippets into their Java applications without requiring external framework integration.
Here's a simplified example demonstrating polyglot programming with GraalVM:
import org.graalvm.polyglot.Context;
import org.graalvm.polyglot.Value;
public class PolyglotExample {
public static void main(String[] args) {
Context context = Context.create();
Value jsFunction = context.eval("js", "function add(a, b) { return a + b; }");
Value result = jsFunction.execute(10, 20);
System.out.println("Result: " + result);
}
}
In this example, we create a GraalVM Context
and use it to evaluate and execute a JavaScript function within a Java application. The js
argument passed to context.eval()
specifies that the JavaScript language is being used.
The Last Word
In this blog post, we've explored how GraalVM Community Edition can be utilized to optimize the performance of Java applications. We've discussed the benefits of GraalVM's JIT compilation, AOT compilation with Substrate VM, and the polyglot capabilities that enable seamless interoperability between different languages.
By harnessing the power of GraalVM, developers can significantly improve the runtime performance of their Java applications, reduce startup times, and leverage the strengths of polyglot programming. To learn more about GraalVM and its features, check out the official documentation on the GraalVM website.
Start optimizing your Java applications with GraalVM today and unleash their full potential!