Improving Cloud Infrastructure: Well-Architected Framework Essentials
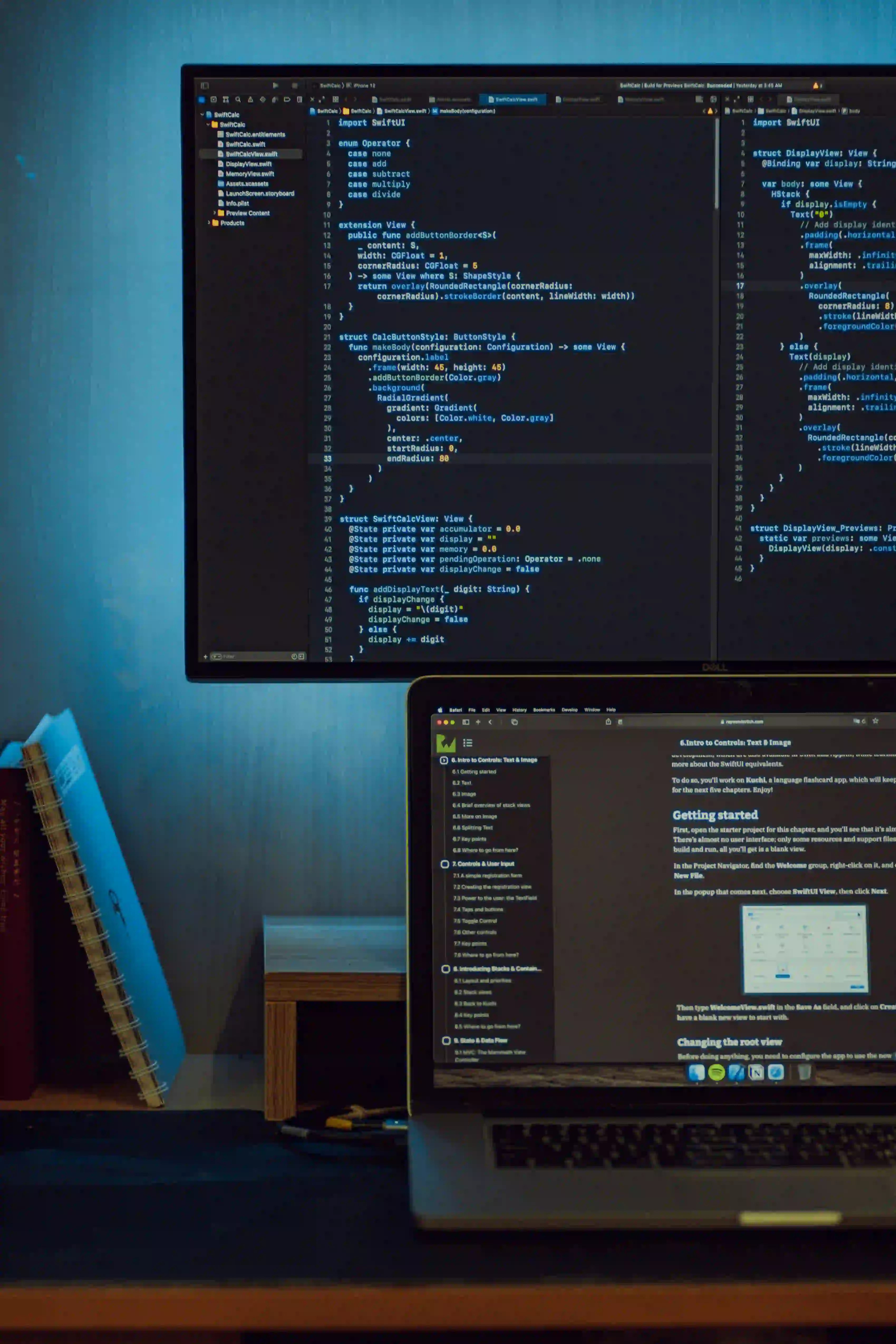
Improving Cloud Infrastructure: Well-Architected Framework Essentials
In the fast-paced world of technology, cloud infrastructure plays a pivotal role in ensuring the reliability, scalability, and security of applications. The Well-Architected Framework provides a set of best practices for architecting secure, high-performing, resilient, and efficient infrastructure for applications. The framework covers architecture, security, reliability, performance efficiency, and cost optimization, guiding developers and architects to make informed decisions that can positively impact their cloud environments. In this post, we will delve into the essentials of the Well-Architected Framework and explore how it can be leveraged to improve cloud infrastructure using Java.
What is the Well-Architected Framework?
The Well-Architected Framework, developed by AWS, offers a structured approach to evaluate architectures and implement designs that will scale with your application and organization. It is designed to help cloud architects build secure, high-performing, resilient, and efficient infrastructure for their applications and workloads.
Five Pillars of the Well-Architected Framework:
- Operational Excellence: Focuses on running and monitoring systems to deliver business value and continually improving processes and procedures.
- Security: Provides the capability to protect information, systems, and assets while delivering business value through risk assessments and mitigation strategies.
- Reliability: Ensures a system can recover from infrastructure or service disruptions, dynamically acquire computing resources to meet demand, and mitigate disruptions such as misconfigurations or transient network issues.
- Performance Efficiency: Uses IT and computing resources efficiently to meet system requirements, and to maintain that efficiency as demand changes and technologies evolve.
- Cost Optimization: Avoids unnecessary costs and focuses on maximizing cost efficiency.
Now, let's dive deeper into the essentials of the Well-Architected Framework and see how it can be applied to improve cloud infrastructure using Java.
Applying the Well-Architected Framework in Java
1. Operational Excellence:
Essential Component: Monitoring and Logging
In a Java application, using a robust logging framework such as Log4j or SLF4J can greatly contribute to operational excellence by providing comprehensive insights into the application's behavior. This helps in identifying and troubleshooting issues, ensuring the application runs smoothly, and providing valuable metrics for continuous improvement.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyClass {
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void doSomething() {
try {
// Code implementation
} catch (Exception e) {
logger.error("An error occurred: {}", e.getMessage());
}
}
}
Why: Incorporating logging in Java applications is essential for monitoring the application's behavior and diagnosing issues, aligning with the operational excellence pillar of the Well-Architected Framework.
2. Security:
Essential Component: Input Validation and Sanitization
Securing a Java application involves ensuring that all user inputs are validated and sanitized to mitigate the risk of vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks. Utilizing libraries like OWASP ESAPI can aid in implementing robust input validation and sanitization mechanisms.
import org.owasp.esapi.ESAPI;
import org.owasp.esapi.errors.ValidationException;
public class UserController {
public void updateUser(String input) {
try {
String safeInput = ESAPI.validator().getValidInput("User input", input, "SafeString", 100, false);
// Update user with safe input
} catch (ValidationException e) {
// Handle validation exception
}
}
}
Why: Implementing input validation and sanitization in Java applications is crucial for bolstering security and aligns with the security pillar of the Well-Architected Framework.
3. Reliability:
Essential Component: Circuit Breaker Pattern Implementation
In Java, leveraging libraries like Resilience4j to implement the circuit breaker pattern can significantly enhance the reliability of the application by preventing cascading failures and providing fallback mechanisms during network or service disruptions.
import io.github.resilience4j.circuitbreaker.CircuitBreaker;
import io.github.resilience4j.circuitbreaker.CircuitBreakerRegistry;
public class MyService {
private CircuitBreaker circuitBreaker;
public MyService() {
CircuitBreakerRegistry registry = CircuitBreakerRegistry.ofDefaults();
circuitBreaker = registry.circuitBreaker("myCircuitBreaker");
}
public void invokeExternalService() {
try {
circuitBreaker.executeSupplier(() -> externalService.call());
} catch (Exception e) {
// Fallback mechanism
}
}
}
Why: Implementing the circuit breaker pattern in Java applications enhances reliability by isolating failures and preventing them from affecting the overall system, aligning with the reliability pillar of the Well-Architected Framework.
4. Performance Efficiency:
Essential Component: Asynchronous Processing with CompletableFuture
Using CompletableFuture in Java allows for efficient asynchronous processing, enabling tasks to execute independently and in parallel, thereby improving the overall performance of the application.
import java.util.concurrent.CompletableFuture;
public class MyService {
public CompletableFuture<String> performAsyncTask() {
return CompletableFuture.supplyAsync(() -> {
// Asynchronous task implementation
return "Task Result";
});
}
}
Why: Leveraging CompletableFuture for asynchronous processing in Java applications enhances performance efficiency by allowing tasks to execute concurrently, aligning with the performance efficiency pillar of the Well-Architected Framework.
5. Cost Optimization:
Essential Component: Efficient Resource Utilization
In Java, leveraging tools like the Java Management Extensions (JMX) to monitor and manage Java applications can aid in identifying resource-intensive components, optimizing resource utilization, and ultimately reducing operational costs.
import javax.management.MBeanServer;
import java.lang.management.ManagementFactory;
public class ResourceMonitor {
private MBeanServer mBeanServer;
public ResourceMonitor() {
mBeanServer = ManagementFactory.getPlatformMBeanServer();
}
public int getActiveThreadCount() {
// Retrieve and monitor active thread count
}
}
Why: Utilizing JMX for efficient resource utilization in Java applications aligns with the cost optimization pillar of the Well-Architected Framework by enabling proactive management of resources, ultimately leading to cost savings.
Wrapping Up
Incorporating the Well-Architected Framework essentials into Java applications is paramount for building resilient, secure, high-performing, and cost-effective cloud infrastructure. By leveraging the best practices of the framework and integrating them into Java development, organizations can ensure that their cloud environments are well-architected and primed for success.
Implementing operational excellence, security, reliability, performance efficiency, and cost optimization within Java applications not only aligns with the pillars of the Well-Architected Framework but also sets the foundation for sustainable and efficient cloud infrastructure.
Be sure to keep the Well-Architected Framework in mind as you architect and build your cloud infrastructure, and leverage the capabilities of Java to implement these essential components effectively. Embracing the framework is a step toward creating robust and optimized cloud infrastructure that can support the ever-evolving demands of modern applications.
By integrating the Well-Architected Framework essentials in Java, you're not only improving your cloud infrastructure but also positioning your applications for long-term success in the dynamic landscape of cloud computing.
Incorporate these essentials, harness the power of Java, and elevate your cloud infrastructure to new heights of performance, security, and efficiency.
For further insights on the Well-Architected Framework and its application, you can refer to the AWS Well-Architected homepage.
Start architecting with excellence, start building with Java.