Creating Custom Interactive Thermostat Control
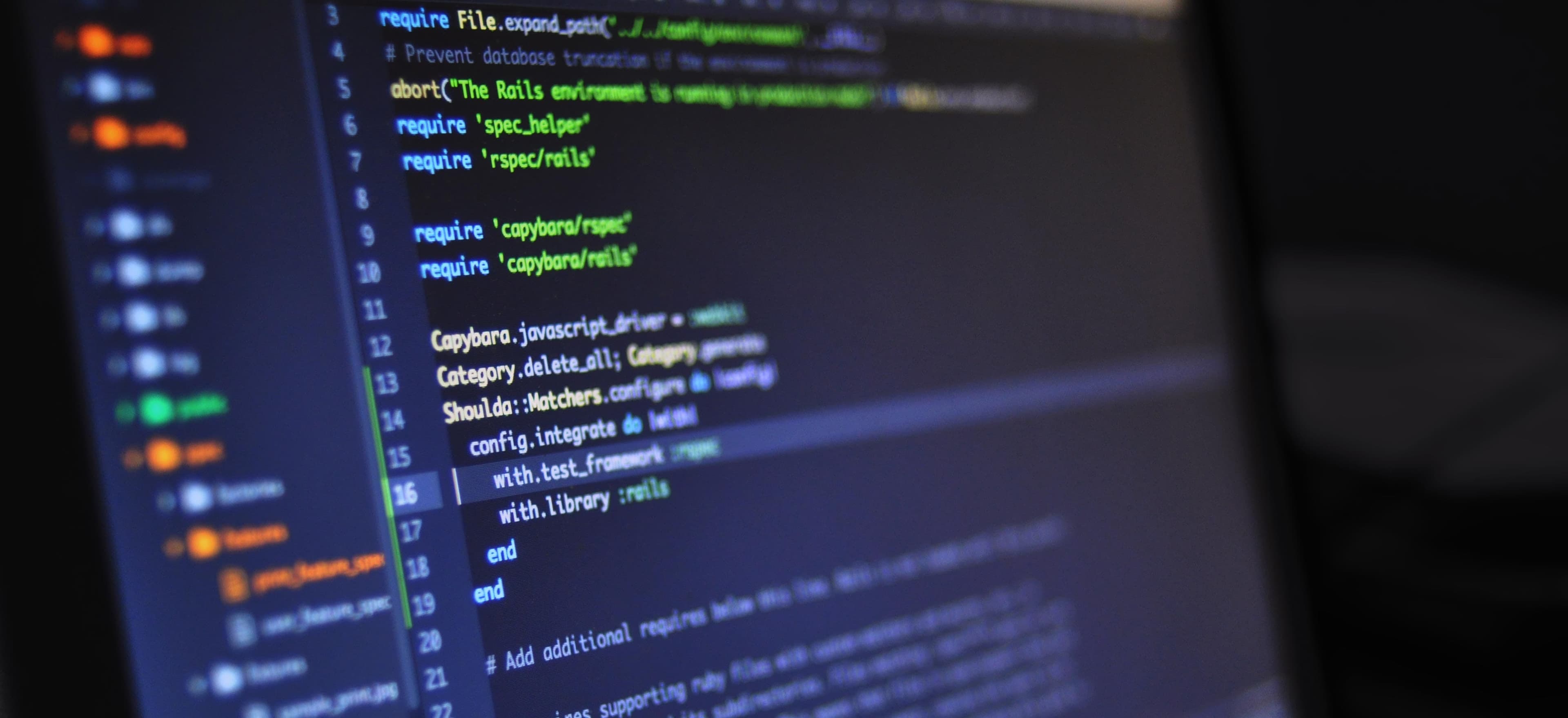
- Published on
Improving User Experience with a Custom Interactive Thermostat Control
In the realm of home automation, the thermostat plays a crucial role in ensuring comfort and energy efficiency. In this blog post, we will explore the process of creating a custom interactive thermostat control using Java. This project will not only enhance the functionality of the thermostat but also provide an immersive user experience. We will cover the implementation of the backend logic, user interface design, and integration with external APIs for real-time weather updates.
Understanding the Requirements
Before diving into the coding aspect, it's essential to outline the requirements for our custom interactive thermostat control. We aim to achieve the following functionality:
- Display current temperature and humidity.
- Allow users to set the desired temperature.
- Provide a visual representation of heating and cooling modes.
- Integrate with a weather API to display real-time weather information.
With these requirements in mind, we can proceed with the implementation.
Backend Logic
We'll start by implementing the backend logic to handle temperature control and integration with the weather API. Java provides robust support for making HTTP requests, making it an ideal choice for integrating external APIs.
// Using HttpURLConnection to make HTTP GET request to weather API
URL url = new URL("https://api.openweathermap.org/data/2.5/weather?q=<city>&APPID=<api_key>");
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("GET");
// Reading the API response
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Parsing the JSON response to extract weather data
JSONObject json = new JSONObject(response.toString());
double currentTemperature = json.getJSONObject("main").getDouble("temp");
double currentHumidity = json.getJSONObject("main").getDouble("humidity");
The code snippet above demonstrates the use of HttpURLConnection
to make an HTTP GET request to a weather API and parse the JSON response to extract the current temperature and humidity.
User Interface Design
Creating an intuitive and visually appealing user interface is crucial for an interactive thermostat control. JavaFX provides a powerful toolkit for building desktop applications with rich UI components.
// Creating a simple thermostat control interface using JavaFX
public class ThermostatUI extends Application {
@Override
public void start(Stage stage) {
// UI code goes here
}
}
The above snippet showcases the entry point for our JavaFX application. Within the start
method, we can define the layout, controls, and event handling for the thermostat interface.
Integrating Backend with UI
To complete the interactive thermostat control, we need to integrate the backend logic with the UI components. This involves updating the UI with real-time temperature and humidity data, as well as handling user interactions to adjust the desired temperature.
// Updating UI with real-time temperature and humidity data
temperatureLabel.setText("Current Temperature: " + currentTemperature + "°C");
humidityLabel.setText("Current Humidity: " + currentHumidity + "%");
// Handling user interactions to adjust desired temperature
temperatureSlider.valueProperty().addListener((observable, oldValue, newValue) -> {
// Update desired temperature logic
});
In the above example, we update the UI labels with real-time temperature and humidity data, and use a slider control to allow users to adjust the desired temperature.
Enhancing User Experience
In addition to the core functionality, we can further enhance the user experience by adding visual cues for heating and cooling modes. This can be achieved by incorporating color changes or icon updates based on the temperature delta between current and desired settings.
// Adding visual cues for heating and cooling modes
if (desiredTemperature > currentTemperature) {
// Set heating mode visual feedback
} else if (desiredTemperature < currentTemperature) {
// Set cooling mode visual feedback
} else {
// Set default mode visual feedback
}
By providing visual cues, we can make the thermostat control more intuitive and engaging for users.
Closing Remarks
In this blog post, we explored the process of creating a custom interactive thermostat control using Java. By implementing the backend logic for data retrieval and user interface design with JavaFX, we were able to meet the requirements of displaying real-time temperature and humidity, allowing users to adjust the desired temperature, and enhancing the user experience with visual cues. Integrating with external APIs for weather updates further enriched the functionality of the thermostat control.
Creating custom interactive controls not only elevates user experience but also demonstrates the versatility of Java in developing innovative solutions for home automation and IoT applications.
By following the outlined steps and incorporating the provided code snippets, you too can create a seamless and immersive user experience with your own interactive thermostat control using Java.
Happy coding!