Troubleshooting Redis Connection Issues in Spring Boot
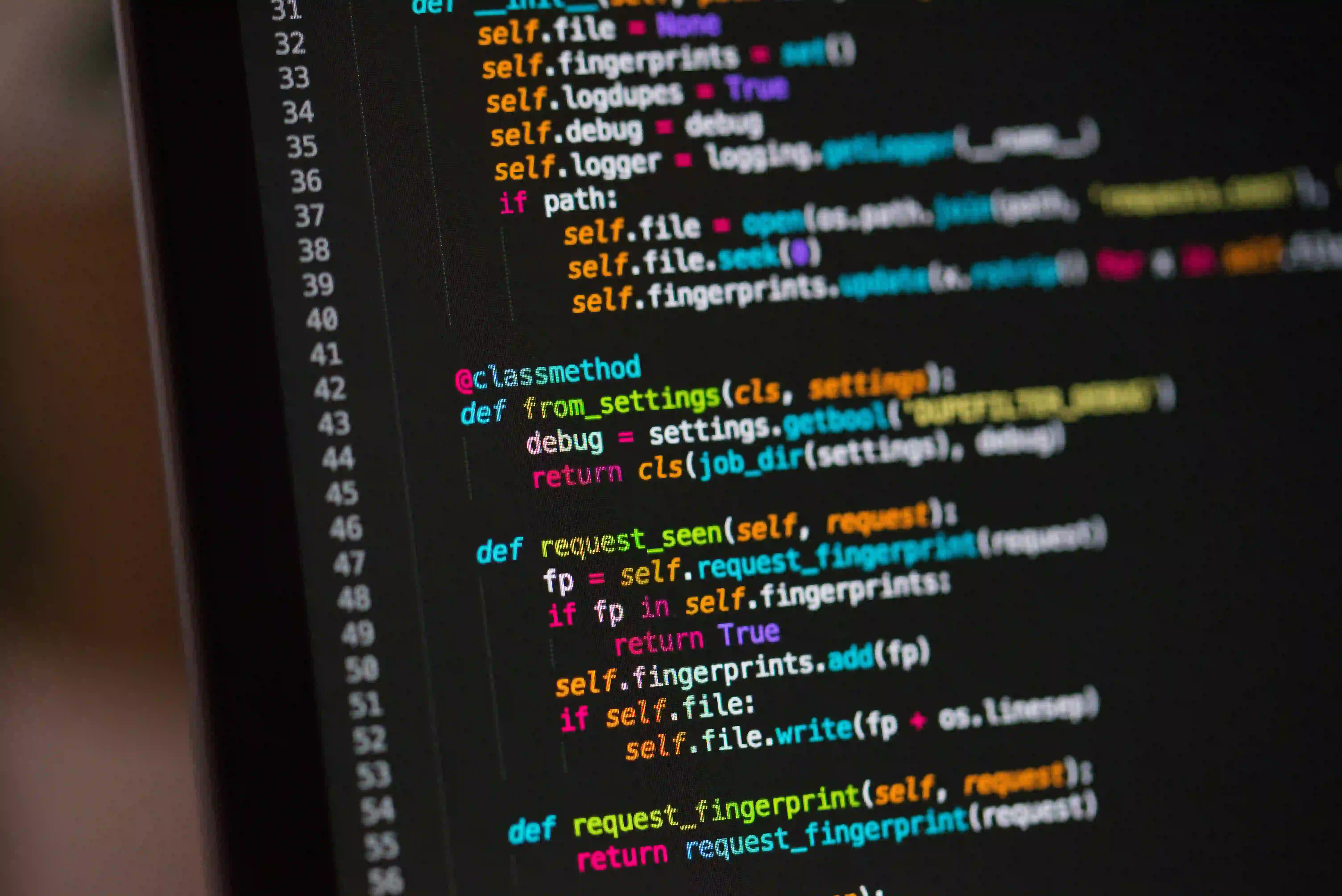
Troubleshooting Redis Connection Issues in Spring Boot
When developing a Spring Boot application that utilizes Redis as a caching or messaging broker, encountering connection issues with Redis is not uncommon. Whether it's a timeout, authentication failure, or simply an inability to connect, these issues can be challenging to troubleshoot. In this article, we will explore common causes of Redis connection problems in a Spring Boot application and discuss how to address them.
1. Dependency Configuration
The first step in troubleshooting Redis connection issues is to ensure that the necessary dependencies are correctly configured in your pom.xml
file. If you are using Maven, you should have the spring-boot-starter-data-redis
dependency included. Make sure to check for any version compatibility issues with other dependencies.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2. Redis Configuration in application.properties
In your application.properties
file, it's essential to configure the Redis connection properties. Ensure that the host, port, and any necessary authentication details are correctly set.
spring.redis.host=your-redis-host
spring.redis.port=6379
spring.redis.password=your-redis-password
3. Correct Redis Template Configuration
When using Redis as a cache or a messaging broker, you'll likely be interacting with Redis through the StringRedisTemplate
or RedisTemplate
in your code. It's crucial to ensure that the template bean is configured correctly. One common issue is the misconfiguration of the bean, leading to connection problems.
@Configuration
public class RedisConfig {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory connectionFactory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(connectionFactory);
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(new Jackson2JsonRedisSerializer<>(Object.class));
return template;
}
}
4. Checking Redis Server Availability
Before diving deep into your Spring Boot application, ensure that the Redis server is up and running. You can use the Redis CLI to check the connection to the server. Use the following command to connect:
redis-cli -h your-redis-host -p 6379 -a your-redis-password
If the connection fails at this stage, it indicates an issue with the server or authentication details. Ensure that the server is running and that the authentication details are correct.
5. Timeout Configuration
Timeout issues are prevalent when dealing with network-based services such as Redis. If you are facing timeouts during Redis operations, consider adjusting the timeout configurations in your application.properties
file.
spring.redis.timeout=3000
spring.redis.lettuce.pool.timeout=2000
Adjust these values based on your application's requirements and network conditions.
6. Firewall and Network Issues
Firewalls and network configurations can sometimes block the connection to the Redis server. Ensure that the necessary ports are open, and there are no network configuration issues preventing the connection.
7. Redis Cluster Configuration
If you are using a Redis cluster, ensure that the cluster configuration is correct in your application. Verify that the RedisClusterConfiguration
is properly set up to establish connections with the Redis cluster.
@Configuration
public class RedisClusterConfig extends CachingConfigurerSupport {
@Bean
public RedisClusterConfiguration redisClusterConfiguration() {
Map<String, Object> source = new HashMap<>();
source.put("spring.redis.cluster.nodes", "your-redis-nodes");
return new RedisClusterConfiguration(new MapPropertySource("RedisClusterConfiguration", source));
}
@Bean
public LettuceConnectionFactory redisConnectionFactory(RedisClusterConfiguration clusterConfiguration) {
return new LettuceConnectionFactory(clusterConfiguration);
}
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory connectionFactory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(connectionFactory);
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(new Jackson2JsonRedisSerializer<>(Object.class));
return template;
}
}
8. Logging and Monitoring
Enable debug logging for the Redis-related packages in your application. This can provide valuable insights into the Redis connection process and any potential issues that arise.
logging.level.org.springframework.data.redis=DEBUG
Additionally, consider using monitoring tools such as Spring Boot Actuator to gain visibility into the health and metrics of your Redis connection.
9. Exception Handling
Implement robust exception handling in your code to gracefully capture and handle Redis connection-related exceptions. Wrap your Redis operations with try-catch blocks to handle potential connection failures and retries where applicable.
The Last Word
Troubleshooting Redis connection issues in a Spring Boot application requires a systematic approach, starting from dependency configurations to advanced connection settings and monitoring. By following these steps and paying attention to detail, you can effectively diagnose and resolve Redis connection problems, ensuring the smooth functioning of your application's interactions with Redis. Remember, understanding the intricacies of the Redis connection process and being proactive in addressing potential issues is key to maintaining a reliable and performant Spring Boot application.