Overcoming Challenges in Implementing Automation Testing
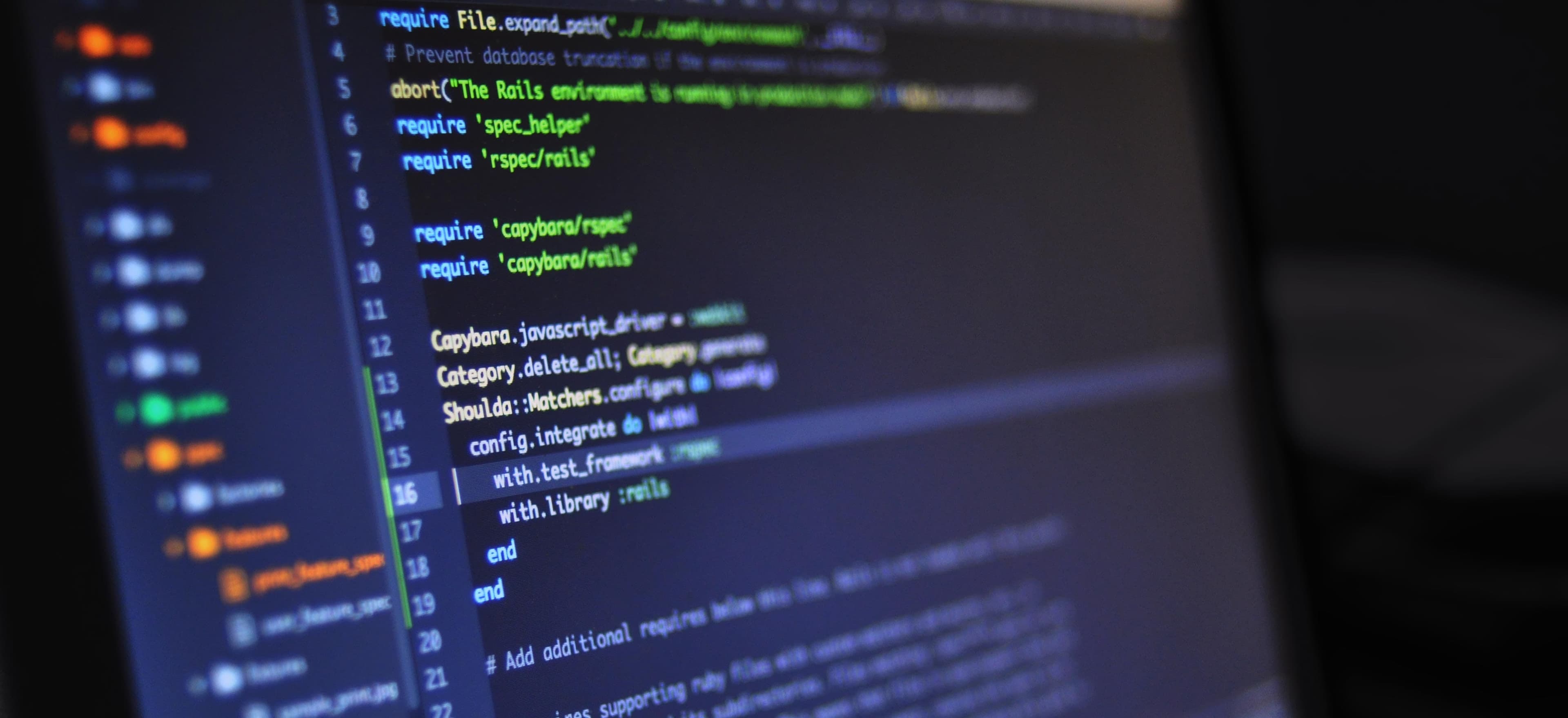
- Published on
Overcoming Challenges in Implementing Automation Testing
In the realm of software development, automation testing has become an indispensable asset for ensuring the quality and reliability of applications. Java, with its robust capabilities, is widely utilized for creating automation test scripts. However, despite its efficacy, implementing automation testing presents several challenges that necessitate adept solutions. This blog delineates some of the common impediments encountered in the implementation of automation testing using Java, along with strategies to overcome them.
Challenge 1: Identifying Stable Locators
One of the foremost challenges in automation testing is the identification and utilization of stable locators to interact with elements on a web page. As the structure and attributes of web elements often undergo dynamic changes, identifying locators that are resistant to such fluctuations is crucial for robust test scripts.
// Example of using stable locators in Selenium WebDriver
WebElement usernameField = driver.findElement(By.id("username"));
WebElement passwordField = driver.findElement(By.id("password"));
Solution: Employing unique attributes like id
or name
for locating elements, as they are less likely to change during UI modifications. Additionally, using XPath and CSS selectors with careful consideration of their stability can contribute to resilient test scripts.
Challenge 2: Handling Asynchronous Behavior
The asynchronous nature of web applications, characterized by dynamic content loading and processing, often leads to synchronization issues in automation testing. This can result in flaky tests due to elements not being immediately available for interaction.
// Code illustrating explicit wait in Selenium WebDriver
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("someid")));
Solution: Implementing explicit waits to synchronize with the dynamic elements, thereby ensuring that the test actions are performed only when the elements are ready. Additionally, leveraging implicit waits to handle asynchronous behavior can aid in stabilizing test scripts.
Challenge 3: Data Management and Test Scalability
Managing test data effectively and ensuring scalability across varied test scenarios pose significant challenges in automation testing. The need to handle diverse input data, along with maintaining test data integrity, necessitates a robust approach.
// Utilizing TestNG data providers for supplying test data
@DataProvider
public Object[][] authenticationData() {
return new Object[][] {{"user1", "pass1"}, {"user2", "pass2"}};
}
Solution: Leveraging parameterization and data-driven testing using frameworks like TestNG, where test data can be supplied from external files or databases. This facilitates scalability and streamlines the management of diverse test data.
Challenge 4: Test Maintenance and Reusability
The maintenance of automation test scripts, especially in complex applications, demands meticulous effort to ensure relevance and reliability amid evolving application features. Additionally, maximizing code reusability to minimize redundancy and enhance test maintainability is imperative.
// Creating reusable functions for login actions in Selenium WebDriver
public void login(String username, String password) {
// Perform login actions
}
Solution: Adopting the Page Object Model (POM) design pattern to encapsulate web elements and their related interactions, thereby fostering easier test maintenance and enhanced reusability. This approach decouples the test scripts from the UI and promotes streamlined modifications.
Challenge 5: Continuous Integration and Execution Efficiency
Integrating automation testing seamlessly into the continuous integration (CI) pipeline and optimizing test execution efficiency are fundamental challenges. The need to orchestrate swift test runs and integrate them with build processes necessitates adept strategies.
// Integrating Maven Surefire plugin for test execution
<plugins>
<!-- Other plugins -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M5</version>
</plugin>
</plugins>
Solution: Integrating automation tests with CI tools like Jenkins, TeamCity, or Bamboo to orchestrate automated test runs during the build process. Furthermore, parallel test execution and optimal resource allocation can be facilitated by leveraging tools like TestNG or JUnit.
My Closing Thoughts on the Matter
In conclusion, while implementing automation testing using Java engenders various challenges, adept strategies and best practices can surmount these impediments. Embracing stable locators, synchronizing with asynchronous behavior, adopting robust data management, leveraging the Page Object Model, and optimizing test execution efficiency are pivotal in realizing the full potential of automation testing. By addressing these challenges effectively, software development teams can fortify their testing processes, bolster application quality, and expedite the delivery of reliable software solutions.
Implementing automation testing is a critical aspect of modern software development. To dive deeper into this topic, I recommend exploring the Selenium WebDriver documentation for Java, as well as resources on TestNG and other testing frameworks. These materials can provide profound insights and best practices for mastering automation testing with Java.